Display list of only product names in flutter, using woocommerce api data in json format
Jbaat, I'd recommend creating a model for your response data and use the values accordingly from each item's instance. There are few online converters available which converts your json response to Dart models, here is one - https://javiercbk.github.io/json_to_dart/. Below is a quick example of what it would look like based on your response data,
class Items {
final List<Items> items;
Items({this.items});
factory Items.fromJson(List<dynamic> json) {
List<Items> itemList = json.map((i) => Items.fromJson(i)).toList();
return Items(
items: itemList
);
}
}
class Item {
final int id;
final String name;
......
Item({this.id, this.name,.....});
factory Item.fromJson(Map<String, dynamic> json) {
return Item(
id: json['id'],
name: json['name']
......
);
}
}
And then your getData()
would be,
Future<Items> getData() async{
var response = await http.get('https://jbaat.com/wp-json/wc/v3/products/?consumer_key=&consumer_secret=');
return Items.fromJson(json.decode(response.body)).items;
}
You should now have Item
list which can be used to get specific item info. You should also use FutureBuilder
to call your getData()
instead of calling it in initState
to make sure data is available before building widgets like so,
@override
Widget build(BuildContext context) {
return FutureBuilder(
future: getData(),
builder: (context, snapshot) {
if (snapshot.hasData) {
return Scaffold(
body: ListView.builder(
itemCount: snapshot.data.length,
itemBuilder: (BuildContext context, int index) {
Item item = snapshot.data[index]; //Your item
return Container(
child: Card(
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Text(item.name),
),
),
);
}),
);
} else {
return Center(child: CircularProgressIndicator());
}
});
}
Hope this helps. Good luck!
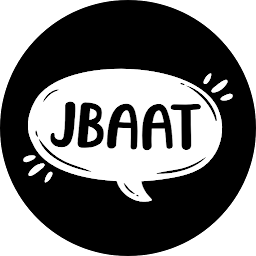
Jbaat Studio
Updated on December 16, 2022Comments
-
Jbaat Studio over 1 year
I am able to fetch and print the data in json format in console and I am able to display the entire body of data in list format in the flutter app. But I can't figure out how to display only a specific key and its value in list. Its a list of maps. I have removed the consumer key and secret from this code.
import 'dart:async'; import 'dart:convert'; import 'package:flutter/material.dart'; import 'package:http/http.dart' as http; void main(){ runApp(MaterialApp( home: CustomHome(), )); } class CustomHome extends StatefulWidget { @override _CustomHomeState createState() => _CustomHomeState(); } class _CustomHomeState extends State<CustomHome> { List data; Future<String> getData() async{ var response = await http.get('https://jbaat.com/wp-json/wc/v3/products/?consumer_key=&consumer_secret='); setState(() { var converted = jsonDecode(response.body); data = converted; }); } @override void initState() { // TODO: implement initState super.initState(); this.getData(); } @override Widget build(BuildContext context) { print(data); return Scaffold( body: ListView.builder( itemCount: data == null ? 0 : data.length, itemBuilder: (BuildContext context, int index) { return Container( child: Card( child: Padding( padding: const EdgeInsets.all(8.0), child: Text('$data'), ), ), ); }), ); } }
Below is the response
[{id: 493, name: Bas 5 Min White Half Sleeve T-Shirt, slug: bas-5-min-white-half-sleeve-t-shirt, permalink: https://www.jbaat.com/product/bas-5-min-white-half-sleeve-t-shirt/, date_created: 2019-12-14T23:39:08, date_created_gmt: 2019-12-14T18:09:08, date_modified: 2019-12-14T23:48:01, date_modified_gmt: 2019-12-14T18:18:01, type: variable, status: publish, featured: false, catalog_visibility: visible, description: , short_description: , sku: , price: 500.00, regular_price: , sale_price: , date_on_sale_from: null, date_on_sale_from_gmt: null, date_on_sale_to: null, date_on_sale_to_gmt: null, price_html: <span class="woocommerce-Price-amount amount"><span class="woocommerce-Price-currencySymbol">₹</span>500.00</span>, on_sale: false, purchasable: true, total_sales: 0, virtual: false, downloadable: false, downloads: [], download_limit: -1, download_expiry: -1, external_url: , button_text: , tax_status: taxable, tax_class: , manage_stock: false, stock_quantity: null, stock_status: instock, backorders: no,
-
Pro over 4 yearsCan you add an example of what your response data look like?
-
Jbaat Studio over 4 yearsI have updated the response I see in the console.
-