display two items in carousel slider flutter
4,392
Solution 1
try: viewportFraction: 0.5, that the container take 50% of screen
options: CarouselOptions(
height: 250.0,
viewportFraction: 0.5,
),
Solution 2
import 'package:flutter/material.dart';
import 'package:carousel_slider/carousel_slider.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
const MyApp({Key key, this.choice, @required this.item}) : super(key: key);
final Choice choice;
final Choice item;
@override
MyAppState createState() => MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Basic demo')),
body: Container(
child: CarouselSlider(
options: CarouselOptions(
disableCenter: false,
),
items: choices
.map((Choice) => Container(
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
Card(
child: Container(
child: Column(
children: [
Text(
Choice.title,
style: null,
textAlign: TextAlign.left,
),
Text(
Choice.content,
style: null,
textAlign: TextAlign.left,
),
],
),
),
),
Card(
child: Container(
child: Column(
children: [
Text(
Choice.title,
style: null,
textAlign: TextAlign.left,
),
Text(
Choice.content,
style: null,
textAlign: TextAlign.left,
),
],
),
),
),
],
),
color: Colors.green,
))
.toList(),
)),
));
}
}
class Choice {
const Choice({this.title, this.content});
final String title;
final String content;
}
const List<Choice> choices = const <Choice>[
const Choice(title: 'title : 1', content: 'content : 1'),
const Choice(title: 'title : 2', content: 'content : 2'),
const Choice(title: 'title : 3', content: 'content : 3'),
const Choice(title: 'title : 4', content: 'content : 4'),
];
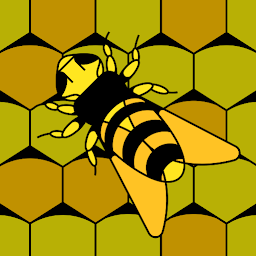
Author by
dinner formal
Updated on December 24, 2022Comments
-
dinner formal over 1 year
for now i`m passing my dummy data to carousel items.
showing two items at the same time in slider is what i aiming for...
but as you know map method traverse one item for the list at a time...
for now i divided carousel item by 2 with Row Widget...
is there any other way to accomplish?
something like increment to be increases 2 at a time for loop...
import 'package:flutter/material.dart'; import 'package:carousel_slider/carousel_slider.dart'; var reports = [ { 'title': '1', 'content': '1' }, { 'title': '2', 'content': '2' }, { 'title': '3', 'content': '3' }, { 'title': '4', 'content': '4' }, ]; Widget buildReport() { return CarouselSlider( options: CarouselOptions( height: 250.0, ), items: reports .asMap() .map( (i, report) { return MapEntry( i, Builder( builder: (BuildContext context) { return Container( child: Row( mainAxisAlignment: MainAxisAlignment.spaceAround, children: [ Card( child: Container( child: Column( children: [ Text( '${report['title']}', ), Text( '${report['content']}', ), ], ), ), ),//i wish these two cards have different data. Card( child: Container( child: Column( children: [ Text( '${report['title']}', ), Text( '${report['content']}', ), ], ), ), ), ], ), ); }, ), ); }, ) .values .toList(), ); }
and i thought about (for loop) but i don`t know how to do that in this code...
-
dinner formal over 3 yearsㅠㅠ so sad...thank you
-