Displaying an uploaded file from $_FILES
13,047
Allright. Totally tested and commented piece of code.
upload.php
define ('MAX_FILE_SIZE', 1000000);
$permitted = array('image/gif', 'image/jpeg', 'image/png', 'image/pjpeg', 'text/plain'); //Set array of permitted filetypes
$error = true; //Define an error boolean variable
$filetype = ""; //Just define it empty.
foreach( $permitted as $key => $value ) //Run through all permitted filetypes
{
if( $_FILES['file']['type'] == $value ) //If this filetype is actually permitted
{
$error = false; //Yay! We can go through
$filetype = explode("/",$_FILES['file']['type']); //Save the filetype and explode it into an array at /
$filetype = $filetype[0]; //Take the first part. Image/text etc and stomp it into the filetype variable
}
}
if ($_FILES['file']['size'] > 0 && $_FILES['file']['size'] <= MAX_FILE_SIZE) //Check for the size
{
if( $error == false ) //If the file is permitted
{
move_uploaded_file($_FILES["file"]["tmp_name"], "upload/" . $_FILES["file"]["name"]); //Move the file from the temporary position till a new one.
if( $filetype == "image" ) //If the filetype is image, show it!
{
echo '<img src="upload/'.$_FILES["file"]["name"].'">';
}
elseif($filetype == "text") //If its text, print it.
{
echo nl2br( file_get_contents("upload/".$_FILES["file"]["name"]) );
}
}
else
{
echo "Not permitted filetype.";
}
}
else
{
echo "File is too large.";
}
Used with this form form.html
<form action="upload.php" method="post"
enctype="multipart/form-data" action="upload.php">
<input type="file" name="file" id="file" size="35"><br>
<input type="submit" name="submit" id="submit" value="Submit">
</form>
</body>
</html>
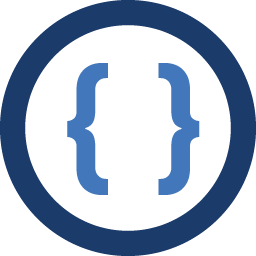
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm having some issues with uploading files from HTML to a PHP based web server. The following is my HTML code. No issues here I believe.
<html> <body> <form action="upload.php" method="post" enctype="multipart/form-data" action="2.2.2.cgi"> <input type="file" name="file" id="file" size="35"><br> <input type="submit" name="submit" id="submit" value="Submit"> </form> </body> </html>
PHP code:
<?php define ('MAX_FILE_SIZE', 1000000); $permitted = array('image/gif', 'image/jpeg', 'image/png', 'image/pjpeg', 'text/plain'); if ($_FILES['file']['type'] == $permitted && $_FILES['file']['size'] > 0 && $_FILES['file']['size'] <= MAX_FILE_SIZE) { move_uploaded_file($_FILES, $uploadedfile); echo ('<img src="'.$uploadedfile'" />'); } ?>
I can't figure out how to make it work in PHP. I've been trying several different ways and the above code is just my latest desperate attempt at figuring it out by trying to store the value into a new variable. What I want to do is the following:
- Restrict the type and size of files that can be uploaded.
- Is the file a picture? Display the picture.
- Is it a plaintext file? Display the contents of the file.
- Is it an unpermitted file? Display the name, type and size of the file.
Any kind of hint or help in any way would be greatly appreciated. Thanks!
PS. It's not going live, so any security issues are irrelevant at the moment.
-
Peter over 11 yearsNo, your answer is wrong. there is no
$uploadedfile
defined, and bad usage ofmove_uploaded_file
as he should point totmp_name
key -
Jonas m over 11 yearsThank you Peter, its getting late here. Edited my answer.
-
Admin over 11 yearsThanks for the help guys. @PeterSzymkowski: I probably should have read the manual a bit more carefully. I'm very new to this, so I'm still learning the ropes.
-
Robert Sinclair over 2 yearsbeautiful comment coding there!