Django check if value exists in the database and create and save if not
13,243
Solution 1
You can use the get_or_create()
method:
obj, created = MyModel.objects.get_or_create(first_name='John', last_name='Lennon')
This could return:
- If it already exist:
-
obj
: The object from your DB -
created
: False
-
- If it does not exist:
-
obj
: The new created object -
created
: True
-
Solution 2
Django has a get or create.
An example would be:
obj, created = CurrencyPairHistory.objects.get_or_create(currency_pair=currency_pair, date=datetime.now().date())
currency_pairs_values.append(obj)
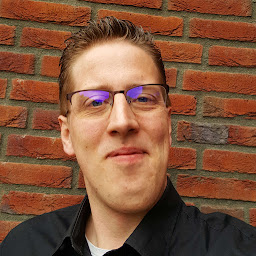
Author by
Johan Vergeer
Updated on June 08, 2022Comments
-
Johan Vergeer almost 2 years
I need to check if a value already exists in the database, if it already exists I can use that value, else I have to create the values, save them to the database and show them to the screen.
def currency_rates(request): currency_pairs_values = [] currency_pairs = CurrencyPair.objects.filter(default_show__exact=True) for currency_pair in currency_pairs: if not CurrencyPairHistory.objects.get(currency_pair__exact=currency_pair, date__exact=datetime.now().date()).exists(): currency_pair_history_value = CurrencyPairHistory() currency_pair_history_value.currency_pair = currency_pair currency_pair_history_value.currency_pair_rate = currency_pair.calculate_currency_pair( datetime.now().date()) currency_pair_history_value.date = datetime.now().date() currency_pair_history_value.save() currency_pairs_values.append(currency_pair_history_value) else: currency_pairs_values.append(CurrencyPairHistory.objects.get(currency_pair__exact=currency_pair, date__exact=datetime.now().date()).exists()) context = { 'currency_pairs_values': currency_pairs_values } return render(request, '../templates/client/currencypairs.html', context)
I got the idea for using the
exists()
method from this link: How to check if something exists in a postgresql database using django? When using this code I get an errorDoesNotExist at /currencypairs/
This is the full stacktrace
Environment: Request Method: GET Request URL: http://127.0.0.1:8000/currencypairs/ Django Version: 1.8.6 Python Version: 3.4.3 Installed Applications: ['django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'client'] Installed Middleware: ['django.middleware.security.SecurityMiddleware', 'django.contrib.sessions.middleware.SessionMiddleware', 'django.middleware.common.CommonMiddleware', 'django.middleware.csrf.CsrfViewMiddleware', 'django.contrib.auth.middleware.AuthenticationMiddleware', 'django.contrib.messages.middleware.MessageMiddleware', 'django.middleware.clickjacking.XFrameOptionsMiddleware'] Traceback: File "/home/johan/sdp/currency-converter/lib/python3.4/site-packages/django/core/handlers/base.py" in get_response 132. response = wrapped_callback(request, *callback_args, **callback_kwargs) File "/home/johan/sdp/currency-converter/currency_converter/client/views.py" in currency_rates 36. date__exact=datetime.now().date()).exists(): File "/home/johan/sdp/currency-converter/lib/python3.4/site-packages/django/db/models/manager.py" in manager_method 127. return getattr(self.get_queryset(), name)(*args, **kwargs) File "/home/johan/sdp/currency-converter/lib/python3.4/site-packages/django/db/models/query.py" in get 334. self.model._meta.object_name Exception Type: DoesNotExist at /currencypairs/ Exception Value: CurrencyPairHistory matching query does not exist.
I hope someone will be able to help me out here. Thanks in advance.