Django - No module named _sqlite3
Solution 1
At the django.db.backends.sqlite3, it tries to
try:
try:
from pysqlite2 import dbapi2 as Database
except ImportError:
from sqlite3 import dbapi2 as Database
except ImportError as exc:
from django.core.exceptions import ImproperlyConfigured
raise ImproperlyConfigured("Error loading either pysqlite2 or sqlite3 modules (tried in that order): %s" % exc)
So one of the modules named sqlite3 or pysqlite2 is not installed. Try to install them
$ pip install sqlite3 # or pysqlite2
Update
sqlite3
and pysqlite2
are part of Python, therefore these two packages are not in PyPi anymore.
Solution 2
You may also have compiled python by hand with no sqlite development library installed
So, do
sudo apt-get install libsqlite3-dev libsqlite3
Then, re-install python
test by entering
python
>>> import sqlite3
Solution 3
I got very similar error message when I tried to run the Django development server:
ImproperlyConfigured: Error loading either pysqlite2 or sqlite3 modules (tried in that order): dlopen(/Users/Rubinous/Projects/Jiiri2/jiiri_venv/lib/python2.7/lib-dynload/_sqlite3.so, 2): Library not loaded: /usr/local/opt/sqlite/lib/libsqlite3.0.8.6.dylib
Referenced from: /Users/Rubinous/Projects/Jiiri2/jiiri_venv/lib/python2.7/lib-dynload/_sqlite3.so
Reason: image not found
I solved this by installing pysqlite
Python module with pip install pysqlite
.
Solution 4
On MacOSX 10.9 (Mavericks), I had to run this command:
CPPFLAGS=-I/usr/local/opt/sqlite/include; LDFLAGS=-L/usr/local/opt/sqlite/lib; pip install pysqlite
I used homebrew to install sqlite3.
brew update; brew install sqlite
Solution 5
This is what I did to get it to work.
I am using pythonbrew(which is using pip) with python 2.7.5 installed.
I first did what Zubair(above) said and ran this command:
sudo apt-get install libsqlite3-dev
Then I ran this command:
pip install pysqlite
This fixed the database problem and I got confirmation of this when I ran:
python manager.py syncdb
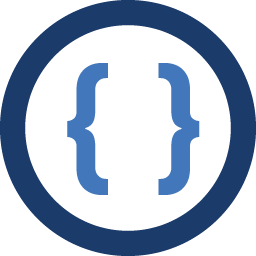
Satyam Garg
Updated on March 11, 2020Comments
-
Satyam Garg about 4 years
I have django 1.4 installed on my rhel 5. By default rhel 5 has python 2.4 in it but to use django 1.4 I manually installed python 2.7.3 The development server is running fine but when I create a new project and after changing the settings.py file as :
'ENGINE': 'django.db.backends.sqlite3', 'NAME': '/home/oracle/Desktop/test1/my.db'
Now when I give python2.7 manage.py syncdb command, I get the error as:
Traceback (most recent call last): File "manage.py", line 10, in <module> execute_from_command_line(sys.argv) File "/usr/local/lib/python2.7/site-packages/django/core/management/__init__.py", line 443, in execute_from_command_line utility.execute() File "/usr/local/lib/python2.7/site-packages/django/core/management/__init__.py", line 382, in execute self.fetch_command(subcommand).run_from_argv(self.argv) File "/usr/local/lib/python2.7/site-packages/django/core/management/__init__.py", line 261, in fetch_command klass = load_command_class(app_name, subcommand) File "/usr/local/lib/python2.7/site-packages/django/core/management/__init__.py", line 69, in load_command_class module = import_module('%s.management.commands.%s' % (app_name, name)) File "/usr/local/lib/python2.7/site-packages/django/utils/importlib.py", line 35, in import_module __import__(name) File "/usr/local/lib/python2.7/site-packages/django/core/management/commands/syncdb.py", line 8, in <module> from django.core.management.sql import custom_sql_for_model, emit_post_sync_signal File "/usr/local/lib/python2.7/site-packages/django/core/management/sql.py", line 6, in <module> from django.db import models File "/usr/local/lib/python2.7/site-packages/django/db/__init__.py", line 40, in <module> backend = load_backend(connection.settings_dict['ENGINE']) File "/usr/local/lib/python2.7/site-packages/django/db/__init__.py", line 34, in __getattr__ return getattr(connections[DEFAULT_DB_ALIAS], item) File "/usr/local/lib/python2.7/site-packages/django/db/utils.py", line 92, in __getitem__ backend = load_backend(db['ENGINE']) File "/usr/local/lib/python2.7/site-packages/django/db/utils.py", line 24, in load_backend return import_module('.base', backend_name) File "/usr/local/lib/python2.7/site-packages/django/utils/importlib.py", line 35, in import_module __import__(name) File "/usr/local/lib/python2.7/site-packages/django/db/backends/sqlite3/base.py", line 31, in <module> raise ImproperlyConfigured("Error loading either pysqlite2 or sqlite3 modules (tried in that order): %s" % exc) django.core.exceptions.ImproperlyConfigured: Error loading either pysqlite2 or sqlite3 modules (tried in that order): No module named _sqlite3
Please suggest the way out?
-
simar about 10 yearsin case of python 2.6 and earlier version need to install pysqlite as a separate lib.
-
Dereckson about 9 yearsThis is now a part of Python (if compiled with this option), so there isn't any sqlite3 package anymore in pip repository: pypi.python.org/pypi/sqlite3 and pypi.python.org/pypi/pysqlite2
-
Alireza Savand about 9 years@Dereckson I've updated the answer regarding to your comment. Thanks
-
Kimmo Hintikka almost 8 yearsThanks this fixed it for me
-
TauPan almost 8 yearsNote that pyenv install <version> will (as of today) warn about missing libzip-devel and readline, but not about missing sqlite3-devel.
-
Jayesh over 7 years@AlirezaSavand I am using django 1.10.3 with python 3.5.2 but am still facing this error. Any solution?
-
Sarkhan about 7 years@AlirezaSavand Same here
-
MikeyE over 6 yearsIf you plan to do any debugging, you might also want to install the debug libraries using
apt-get install libsqlite3-0-dbg
. -
sunsetjunks over 5 yearsFaced a missing sqlite3 for common django commands on a fresh centos7 installation. We had python2.7 altinstall to have the latest python on-bpard. Installing sqlite3 via yum and recompiling python from source saved us!