django quiz app model for multiple choice questions
For a properly normalized relational database schema, you want a distinct Choice
model with a foreign key on Question
:
class Question(models.Model):
question = models.CharField(...)
class Choice(models.Model):
question = models.ForeignKey("Question", related_name="choices")
choice = modelsCharField("Choice", max_length=50)
position = models.IntegerField("position")
class Meta:
unique_together = [
# no duplicated choice per question
("question", "choice"),
# no duplicated position per question
("question", "position")
]
ordering = ("position",)
And then you can get at a Question
's choices with myquestion.choices.all()
(and get the question from a Choice
with mychoice.question
).
Note that this won't impose any limitation on the number of choices for a Question, not even mandates that a Question has at least one related Choice.
Unless you have a very compelling reason to do otherwise, a properly normalized schema is what you want when using a relational database (rdbms are much more than mere bitbuckets, they offer a lot of useful features - as long as you do have a proper schema, that is).
Related videos on Youtube
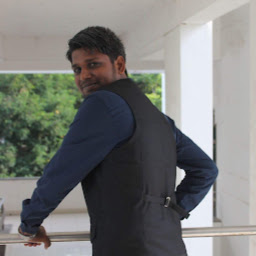
Comments
-
Sakthi Panneerselvam almost 2 years
I am creating quiz app in django, my django model for questions is like this,
class Question(models.Model): questions = models.CharField(max_length=50, unique=True) choice1 = models.CharField(max_length=50, unique=True) choice2 = models.CharField(max_length=50, unique=True) choice3 = models.CharField(max_length=50, unique=True) choice4 = models.CharField(max_length=50, unique=True) correct_answer = models.CharField(max_length=50, unique=True)
is this fine or save the four options in postgres array or save the choices in separate table.
-
Sakthi Panneerselvam over 6 yearshow will save multiple choice in single field like postgres array and i need to save correct answer also
-
bruno desthuilliers over 6 yearsYou asked for recommandations on the best way to design your model. My recommandation is to use a properly normalized schema. If you want to use some denormalized schema instead please do, but I won't answer on this because I don't think this would really help. If you wonder why, try both solutions, and see which one makes your life easier when it comes to handling answers, scores, and just any stats on your questions/choices/answers.
-
Sakthi Panneerselvam over 6 yearsThank you. now only i get it what you're trying to say.