Django Select query with specific column
16,273
Thing that stands out is that:
mperson = GData.objects.get(id=g_id).values('person')
should probably be:
mperson = GData.objects.get(id=g_id).person
You should also probably rethink your view logic, just looking quickly something like this is slightly better, but can probably be improved:
from django.shortcuts import get_object_or_404
def message(request, g_id):
mperson = get_objects_or_404(GData, id=g_id).person
if request.method == 'POST':
n = request.POST.get('bname')
p = request.POST.get('bphone')
e = request.POST.get('bemail')
m = request.POST.get('bmsg')
msg_job = MessagePerson(person=mperson, mname=n, mPhone=p, memail=e, message=m)
msg_job.save()
return render_to_response('ivent.html', context_instance=RequestContext(request))
return HttpResponse('Not a post request')
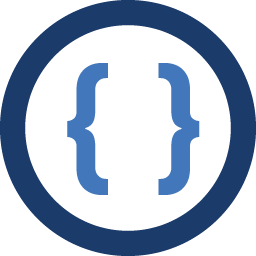
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have a model called
MessagePerson
which has the fields of details which depict the messages for a particular person, who's details are inPersonal
models.I even have separate model which has a foreign key reference to
Personal
class.class Personal(models.Model): name = models.CharField(max_length=20,primary_key=True) email = models.EmailField(blank=True,null=True) address = models.CharField(max_length=50,blank=True,null=True) contact = models.CharField(max_length=20) pic = models.FileField(upload_to='image/',blank=True,null=True) def __unicode__(self): return self.name class MessagePerson(models.Model): person = models.ForeignKey(Personal, related_name='msg') mname = models.CharField(max_length=30) mPhone = models.CharField(max_length=20,blank=True,null=True) memail = models.EmailField(blank=True,null=True) message = models.CharField(max_length=200,blank=True,null=True) def __unicode__(self): return self.person.name class GData(models.Model): person = models.ForeignKey(Personal, related_name='everyperson') place = models.CharField(max_length=40) typeOfProperty = models.CharField(max_length=30) typeOfPlace = models.CharField(max_length=20) price = models.IntegerField() def __unicode__(self): return self.person.name
Now the thing I require is, I have the
id
ofGData
using which i should save a message for the particular 'MessagePersonusing name of
Personal`The query I used is : Views.py
def message(request, g_id): n = request.POST['bname'] p = request.POST['bphone'] e = request.POST['bemail'] m = request.POST['bmsg'] mperson = GData.objects.get(id=g_id).values('person') msg_job = MessagePerson(person=mperson, mname=n, mPhone=p, memail=e, message=m) msg_job.save() return render_to_response('ivent.html', context_instance=RequestContext(request))
Now the thing is, without any error, it is not even storing the message :(
Please help me to get the result :(
-
JamesO almost 13 yearsDTing, your right - my answer wouldn't have worked - that will teach me for not checking an answer :-)
-
Admin almost 13 years@DTing : Ur solution worked when i used it in
python manage.py shell
, but through the website it is not saving the object. :( Should i provide any further details? The site when submits just gives back the same page without even the success notice, which i have created . -
dting almost 13 yearsHave you checked the database to see if the instance is saved?
-
dting almost 13 yearsAlso, after your save it seems like you would want to redirect to another view not render_to_response.
-
Admin almost 13 years@DTing : I did check the database. I checked them through the admin site of django. If i put the data from
shell
it gets saved. Before this there was another same insert type situation. That works perfectly. But this doesn't. And Yea I am rendering them to same page not redirecting :(