Do I have to initialize simple class member variables?
Solution 1
Do I have to initialize simple class member variables,
No, you don't have to initialize the member variables. If you do not initialize them though, do not assume they will have any value.
If you make a test program and check in debugger how the values are initialized you may see them initialized as zeros, but that is not guaranteed. The values get initialized to whatever is in memory on the stack at that location.
or are they guaranteed to get assigned their default values in any case?
They are not guaranteed to get assigned any value. If you have member objects the default constructors will be called for them, but for POD types there is no default initialization.
Even though you don't have to do it, it is good practice to initialize all members of your class (to avoid hard to find errors and to have an explicit representation of the initializations / constructor calls).
Solution 2
Yes, that is necessary. Your object will be allocated either on the stack or on the heap. You have no way of knowing what values that memory contains, and the runtime does not guarantee zeroing this for you, so you must initialise the variable.
Solution 3
Read this. This page is also helpful for many other questions you may have down the stone road of learning C++.
In general, you don't have to, but you should. Relying on default values can really come back hard on you. Also this makes the code better to understand for others. Keep in mind that the initialization list does not affect the order in which members are initialized, that is determined by the order they are declared in the class.
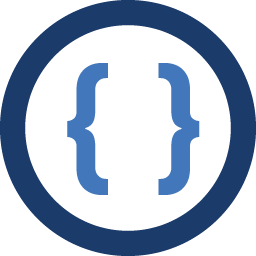
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
Quick beginner's question:
Do I have to initialize simple class member variables, or are they guaranteed to get assigned their default values in any case?
Example:
class Foo { int i; // is i==0 or do I need the following line for that? Foo() : i(0) {} };
Thanks!
-
fredoverflow almost 14 yearsThey can also be allocated in static storage. I believe value-initialization is performed in that context, so i would be 0 automatically, but I'm not sure.
-
Björn Pollex about 8 years@vlad_tepesch, thanks for notice, it got moved here.
-
Darioush almost 8 yearsThis answer is so much better and simpler than the top one.
-
Melebius about 7 years@Darioush Yes, it is simpler as it covers the usual case only while the top one covers all cases.
-
Admin about 6 yearsYou don't have to, so saying it is necessary is just wrong.
-
AJ. about 6 yearsThe original questioner gives the alternative "or do the variables get assigned their default values". They do not. Therefore in the context that the op wants to know what initial values are present, it is necessary and saves a lot of pain.
-
AJ. about 6 yearsPS I quite agree with @melebius that the accepted answer is fuller and more explicit than mine :)