Does AJAX loaded content get a "document.ready"?
Solution 1
To answer your question: No, document.ready will not fire again once a ajax request is completed. (The content in the ajax is loaded into your document, so there isn't a second document for the ajax content).
To solve your problem just add the event listener to the Element where you load the ajax content into it. For example:
$( "div.ajaxcontent-container" ).on( "click", "#id-of-the-element-in-the-ajax-content", function() {
console.log($( this ));
});
For #id-of-the-element-in-the-ajax-content
you can use any selector you would use in $("selector")
. The only difference is, only elements under div.ajaxcontent-container
will be selected.
How it works:
As long as div.ajaxcontent-container
exists all elements (if they exist now or only in the future) that match the selector #id-of-the-element-in-the-ajax-content
will trigger this click-event.
Solution 2
Javascript in the resulting ajax call will not be excecuted (by default) due to safety. Also, you can't directly bind event to non-existing elements.
You can bind an event to some parent that does exist, and tell it to check it's children:
$(document).ready(function(){
$(document).on('eventName', '#nonExistingElement', function(){ alert(1); }
// or:
$('#existingParent').on('eventName', '#nonExistingElement', function(){ alert(1); }
});
Always try to get as close to the triggering element as you can, this will prevent unnessesary bubbling through the DOM
If you have some weird functions going on, you could do something like this:
function bindAllDocReadyThings(){
$('#nonExistingElement').off().on('eventName', function(){ alert(1); }
// Note the .off() this time, it removes all other events to set them again
}
$(document).ready(function(){
bindAllDocReadyThings();
});
$.ajaxComplete(function(){
bindAllDocReadyThings();
});
Solution 3
try this, that is not working because your control is not yet created and you are trying to attach a event, if you use on event it will work fine. let me know if you face any issues.
$(document).ready(function(){
$(document).on('click', '#element', function (evt) {
alert($(this).val());
});
});
Solution 4
The answer here is a delegated event:
jQuery
$(document).ready(function(){
// Listen for a button within .container to get clicked because .container is not dynamic
$('.container').on('click', 'input[type="button"]', function(){
alert($(this).val());
});
// we bound the click listener to .container child elements so any buttons inside of it get noticed
$('.container').append('<input type="button" class="dynamically_added" value="button2">');
$('.container').append('<input type="button" class="dynamically_added" value="button3">');
$('.container').append('<input type="button" class="dynamically_added" value="button4">');
$('.container').append('<input type="button" class="dynamically_added" value="button5">');
});
HTML
<div class="container">
<input type="button" class="dynamically_added" value="button1">
</div>
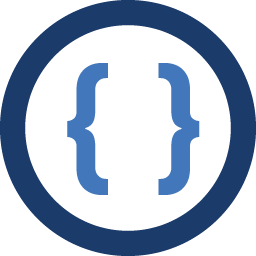
Admin
Updated on January 30, 2020Comments
-
Admin about 4 years
Yesterday I had an issue where a
.on('click')
event handler I was assigning wasn't working right. Turns out it's because I was was trying to apply that.on('click')
before that element existed in the DOM, because it was being loaded via AJAX, and therefore didn't exist yet when thedocument.ready()
got to that point.I solved it with an awkward workaround, but my question is, if I were to put a
<script>
tag IN the ajax loaded content and anotherdocument.ready()
within that, would that seconddocument.ready()
be parsed ONLY once that ajax content is done being loaded? In other words, does it consider that separately loaded ajax content to be anotherdocument
, and if so, does having anotherdocument.ready()
within that ajax-loaded HTML work the way I think it does?Alternatively; what would be a better way to handle this situation? (needing to attach an event listener to a DOM element that doesn't yet exist on
document.ready()
) -
Admin over 10 yearsThanks! Most answers were the same but yours specifically answered my question and then went on to explain event delegation. Cheers!
-
Smamatti almost 10 yearsThis doesn't work without errors if you don't have the functions on all loaded pages. Therefore I suggest to add something like
$(document).ajaxStart(function() { delete window.bindAllDocReadyThings; });
and rather define the functions within the documents asbindAllDocReadyThings = function () { ... }
-
Vitaliy Markitanov over 8 yearsActually document.ready will be called in ajax-loaded HTML. Try to add into ajax-loaded page <script> $(function(){console.log('message from ajax-loaded page!!')}) </script> and you will see that message logged. But function.ready on main page is not fired after ajax.. just to be clear.
-
user1451111 over 5 years@VitaliyMarkitanov Can you please explain this function.ready on main page is not fired after ajax
-
Vitaliy Markitanov over 5 yearslook at this psedo page <parentPage> $(readyParentWhenParenLoaded) <ChildPage> $(readyChild) </ChildPage> </parentPage> So when you load child page from parent via ajax call readyParentWhenParenLoaded will not be invoked, but readyChild will
-
paulokunev over 2 yearsGreat answer, saved my time, because I didn't understand event dumping and bubbling at that time. The key feature of this approach is to add event handler to unremovable parent of dynamically loaded part of the DOM.