does anyone have a working example of a fetched-property in core-data?
Solution 1
Here's my relevant bits of code (including bits you've already mentioned):
My example has a 'Card' object that has a 1->many relationship with a 'Stats' object. Each 'Stats' object has an 'outcome' that can be 1-4. My fetched property is a simple one to give my 'Card' object an array of 'Stats' objects that are of 'outcome'=1 only.
I wanted to use the fetched property so that I could easily get hold of 'Card' objects that had more than a certain number and kind of 'Stats' objects.
So, in the 'Card' object I put the Fetched Property 'statsOfTypeOne', with Destination set to 'Stats'.
In the predicate for this fetched property I put
(SELF.outcome=1) AND (SELF.card=$FETCH_SOURCE)
'SELF' is the 'stats' record, and $FETCH_SOURCE magically becomes the 'Card' object when executed.
As you did, I put the following in the .h and .m files for the 'Card' object:
@property (nonatomic, retain) NSArray *statsOfTypeOne;
@dynamic statsOfTypeOne;
Then in my code I used:
[self.managedObjectContext refreshObject:cardInstance mergeChanges:YES];
[cardInstance valueForKey:@"statsOfTypeOne"]
to get at the array (although cardInstance.statsOfTypeOne should be fine). Without the refresh object it wasn't updating the Fetched property (as per the manual).
I think that's everything that I did to make it work. Let me know if it works for you.
Peter
Solution 2
Adding to @Peter's answer. Here's how I got it working in Swift 2.0 and Xcode 7:
import Foundation
import CoreData
@objc(Card)
class Card: NSManagedObject {
@NSManaged var statsOfTypeOne: [Stat]
}
And then, to read the fetched property:
managedObjectContext.refreshObject(someCard, mergeChanges: true)
// This works and returns [Stat] type
someCard.statsOfTypeOne
// So does this
someCard.valueForkey("statsOfTypeOne") as! [Stat]
Solution 3
Have you taken a look at this previous question: Xcode 4 Core Data: How to use fetched property created in Data Model editor
Read through the accepted answer and all of the comments. It sounds like they have it sorted out.
Related videos on Youtube
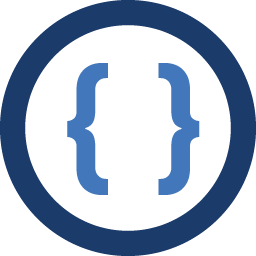
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have tried to use fetched properties a couple of times, and although it seems to be the right approach, it never works.
In my latest attempt I added the fetched-property to my entity, selected the other entity in the model as the 'destination', and set the predicate to a condition that I know is valid.
Problem 1: When the NSManagedObject-class for the entity is generated it does not include anything for the fetched-property. After some searching I added the declaration for it in the .h file and the @dynamic statement for it in the .m file (yes, I know it's an NSArray * type).
Problem 2: Even after that, when I access this property in code I get an exception being thrown that states something to the effect that the fetch-request does not have an entity. I am assuming that the 'entity' would be the one specified as the 'destination' and it is, in fact, there.
So, I'd like someone to provide a concrete working example (iOS platform) where a fetched-property is defined in the model, declared in a NSManagedObject-derived class, and actually used from code.
At this point I am giving up on this time-waster and simply implementing the fetch-request code myself.
-
Sixten Otto over 12 yearsHaving the same problem: the entity is set in the model (and I can see that in the
contents
XML) but the compiled model that's loaded into the application doesn't have target entities set. Frustrating!
-
-
Admin over 12 yearsThanks sosborn, but I've already been through that one -- that's where I found out the answer to Problem 1 in my post -- but using the property in the app throws the exception outlined in Problem 2.