Does std::vector call the destructor of pointers to objects?
Solution 1
No.
How is std::vector
supposed to know how to destroy the pointed-to object? Should it use delete
? delete[]
? free
? Some other function? How is it supposed to know the pointed-to objects are actually dynamically allocated or that it is the One True Owner and is responsible for destroying them?
If the std::vector
is the One True Owner of the pointed-to objects, use std::unique_ptr
, potentially with a custom deleter to handle the cleanup of the objects.
Solution 2
As you said correctly yourself, vector does call destructors for its elements. So, in your example the vector does call "destructors of pointers". However, you have to keep in mind that pointer types have no destructors. Only class types can have destructors. And pointers are not classes. So, it is more correct to say that std::vector
applies the pseudo-destructor call syntax to the pointer objects stored in the vector. For pointer types that results in no-operation, i.e it does nothing.
This also answers the second part of your question: whether the myclass
objects pointed by the pointers get destroyed. No, they don't get destroyed.
Also, it seems that you somehow believe that "calling destructors on pointers" (the first part of your question) is the same thing as "destroying the pointed objects" (the second part of your question). In reality these are two completely different unrelated things.
In order to create a link from the former to the latter, i.e. to make the vector destroy the pointed objects, you need to build your vector from some sort of "smart pointers", as opposed to ordinary raw myclass *
pointers. The vector will automatically call the destructors of the "smart pointers" and these destructors, in turn, will destroy the pointed objects. This "link" can only be implemented explicitly, inside the "smart pointer's" destructor, which is why ordinary raw pointers can't help you here.
Solution 3
No; what if you stored a pointer to an automatic object?
vector<T*> v;
T tinst;
v.push_back(&tinst);
If the vector calls the destructors of the objects the pointers point to, the automatic object would be destructed twice - once when it went out of scope, and once when the vector went out of scope. Also, what if they are not supposed to be deallocated with delete
? There's no way it could behave appropriately in every situation.
If your objects are all allocated dynamically, you have to manually iterate the vector and delete
each pointer if it was allocated with new
. Alternatively, you can create a vector of smart pointers which will deallocate the objects pointed to by the pointers:
vector<shared_ptr<T>> v;
v.push_back(new T);
Related videos on Youtube
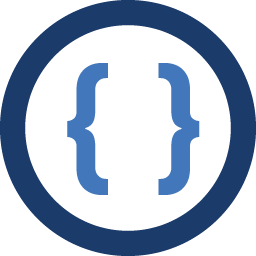
Admin
Updated on July 10, 2022Comments
-
Admin almost 2 years
Possible Duplicate:
Deleting pointers in a vectorI know when an
std::vector
is destructed, it will call the destructor of each of its items. Does it call the destructor of pointers to objects?vector<myclass*> stuff;
When stuff is destroyed, do the individual objects pointed to by the pointers inside stuff get destructed?
-
Martin York about 12 yearsCheck out boost::ptr_vector<my_class>
-
-
Seth Carnegie about 12 yearsActually if you want to be precise,
std::vector
does not destruct anything, the allocator does -
Kerrek SB about 12 yearsHm. I think the second word, "because", is misleading. The onus to justify not deleting pointees is not on the vector; rather, the OP must explain why a container should treat pointer-typed elements differently. As it stands, the answer could be a simple, "No.".
-
Dean Burge about 12 yearsIf
std::vector
would have a constructor that takes a destruction function for the elements that would be dandy. It would be useful for legacy or C API. But anyhow the better way of doing things is wrapping this sort of resources with a RAII class. -
Seth Carnegie about 12 years@wilhelmtell you can write a custom allocator for that :) Or give
unique_ptr
a custom deleter function. -
Dean Burge about 12 years@SethCarnegie yeah, it hit me seconds after posting the comment. happens to me all the time, talking before thinking. :-S
-
Jo So over 9 yearsThis should be the accepted answer as it shows why pointers are no special case.