Does String's toString() method have any practical purpose?
Solution 1
Besides filling conventions or using computing resources, what does a .toString() in Java do on a String that using the String itself wouldn't?
It means it gives an appropriate result when called polymorphically.
Why doesn't it simply inherit the .toString() from class java.lang.Object?
Because that wouldn't give the same result, and almost certainly not the desired result.
Object.toString()
is meant to give a reasonably useful string representation of the object. The Object
implementation gives information about the type and a value which can be used for crude identity hints (diagnostically useful, but that's all). That's clearly not the most useful string representation for a string - the string itself is.
While I would say that it's a pity that toString
is defined in quite a woolly way (it's not clear whether the result is meant for machine, developer or user consumption), it feels obvious to me that a String
would return itself in the implementation.
Solution 2
Practical example:
public abstract class MetadataProcessor {
protected void processMetadata() {
Map<String, Object> metadata = getMetadata();
for(String key : metadata.keySet()) {
if(metadata.get(key) instanceof Date) {
processDate(metadata.get(key));
} else { //String or any other object type
processString(metadata.get(key).toString());
}
}
private void processDate(Date date) {
(...)
}
private void processString(String string) {
(...)
}
/**
* contains document's metadata, values must be String or Date
**/
protected abstract Map<String, Object> getMetadata();
}
If String's .toString() wouldn't return the String itself, an additional if(foo instanceof String)
would be needed in above code.
Solution 3
Obviously old, but another answer that avoids the need of (foo instanceof String) is when dealing with generics.
It'd be annoying to get a string representation of every object, but then string is the odd man out requiring extra code to get the same result.
Solution 4
public final class String
extends Object
implements Serializable, Comparable<String>, CharSequence
see: https://docs.oracle.com/javase/10/docs/api/java/lang/String.html
All object has toString()
method (see https://docs.oracle.com/javase/10/docs/api/java/lang/Object.html#toString() ). String
is a type of object, therefore, it also need a method toString()
for implementing method from its base class.
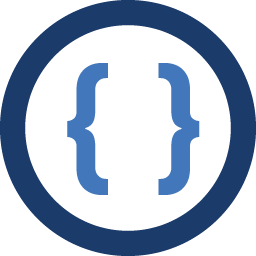
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I was reading the docs and noticed it. Never imaginated it. The description:
This object (which is already a string!) is itself returned.
Besides filling conventions or using computing resources, what does a
.toString()
in Java do on aString
that using theString
itself wouldn't? Why doesn't it simply inherit the.toString()
from classjava.lang.Object
?EDIT:
I understand that in situations of polymorphism an own
toString()
method has to exist since it overrode its parent'stoString()
. What I want to know in the first question is if there is any situation where you'll get something different between usingstringVariable
/"String value"
and usingstringVariable.toString()
/"String value".toString()
.Ex. gr.: An output operation like
System.out.println(stringVariable.toString());
or a value assignment likestringVariable = "String value".toString();
. -
Louis Wasserman almost 12 yearsEffective Java item 10 discusses some reasons to avoid fully specifying
toString()
: because someone will inevitably decide it's a good idea to parse it. -
Jon Skeet almost 12 years@TrevorTrovalds: Doesn't the documentation already answer that? It returns "this object" - so no, there's no situation where using
foo
andfoo.toString()
will give a different result wherefoo
is a non-null reference to aString
. -
Jon Skeet almost 12 years@TrevorTrovalds: Um, ish - although I wouldn't use it for that purpose.
-
ryvantage over 10 years@Trevor... I'm scratching my head trying to envision a scenario where throwing a NPE with
str.toString()
would be better than aif str == null
check... -
JohnDoDo almost 10 years@user985358: Trevor, you recently unaccepted this answer as well as others. Is it something wrong with this answer or the others or had you other reasons to unnaccept so many answers?
-
halfer almost 10 years@JohnDoDo: the OP may not see your comment there, since they have no comments in this thread (they may have deleted them since you posted). I'll put a note on the question.