downloading file from Spring Rest Controller
11,545
The below works for me:
@RequestMapping("/")
public void index(HttpServletRequest request, HttpServletResponse response) throws IOException {
FileInputStream inputStream = new FileInputStream(new File("C:\\work\\boot\\pom.xml"));
response.setHeader("Content-Disposition", "attachment; filename=\"testExcel.xlsx\"");
response.setContentType(MediaType.APPLICATION_OCTET_STREAM_VALUE);
ServletOutputStream outputStream = response.getOutputStream();
IOUtils.copy(inputStream, outputStream);
outputStream.close();
inputStream.close();
}
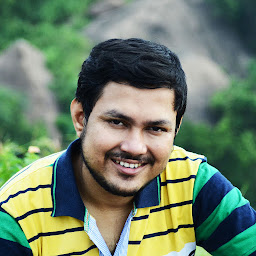
Author by
pradipta gure
Updated on June 04, 2022Comments
-
pradipta gure almost 2 years
I am trying to download a file through Spring REST Controller. Below is my code -
@RequestMapping(value="/aaa",method=RequestMethod.GET,produces=MediaType.APPLICATION_OCTATE_STREAM_VALUE) public ResponseEntity<byte[]> testMethod(@RequestParam("test") String test) { HttpHeaders responseHeaders = new HttpHeaders(); responseHeaders.setContentDispositionFromData("attachment","testExcel.xlsx"); responseHeaders.setContentType(MediaType.APPLICATION_OCTET_STREAM); File file = new File("C:\\testExcel.xlsx"); Path path = Paths.get(file.getAbsolutePath()); ByteArrayResource resource = new ByteArrayResource(Files.readAllbytes(path)); return new ResposeEntity<byte[]>(resource.getByteArray(),responseHeaders,HttpStatus.OK); }
This is called in a button click. After I click the button nothing happens. While debugging this java, I could see the bytestream. In developer tools of Mozilla, I could see successful HTTP response(response in bytestream of that excel file). As per resources available on internet, browser should automatically download the file, but that's not happening.
Why downloading is not happening in browser? What's more I need to do to make it work?
NOTE : This is just for POC purpose. In actual time, I have to generate the excel file from database details, through Apache POI or some other APIs.
-
pradipta gure about 7 yearsWithout using HttpServletRequest and HttpServletResponse, isn't it possible? I am using ResponseEntity here.
-
Essex Boy about 7 yearsYou just need to write to the response output stream this is the key. You don't need the request in this example. The response entity it is not needed. This code works :)
-
pradipta gure about 7 yearsStill I am getting bytestream in the response. File is not automatically in downloaded in the browser. I am calling this controller from angular js. Is there anything needs to be done from angularjs?