Drawing in a Win32 Console on C++?
Solution 1
No you can't just do that because Win32 console doesn't support those methods. You can however use GDI to draw on the console window.
This is a great example of drawing a bitmap on a console by creating a child window on it: http://www.daniweb.com/code/snippet216431.html
And this tells you how to draw lines and circles:
http://www.daniweb.com/code/snippet216430.html
This isn't really drawing in the console though. This is sort of drawing "over" the console but it still does the trick pretty well.
Solution 2
Yes, it is possible.
Get the HWND
of the console window using GetConsoleWindow and then draw in it.
#define _WIN32_WINNT 0x601
#include <windows.h>
#include <stdio.h>
int main() {
// Get window handle to console, and device context
HWND console_handle = GetConsoleWindow();
HDC device_context = GetDC(console_handle);
//Here's a 5 pixels wide RED line [from initial 0,0] to 300,300
HPEN pen = CreatePen(PS_SOLID, 5, RGB(255, 0, 0));
SelectObject(device_context, pen);
LineTo(device_context, 300, 300);
ReleaseDC(console_handle, device_context);
getchar();
return 0;
}
Note: GetConsoleWindow
was introduced in Windows 2000. It's available when _WIN32_WINNT
is set to 0x500 or greater.
Solution 3
It is possible, albeit totally undocumented, to create a console screen buffer that uses an HBITMAP
that is shared between the console window process and the calling process. This is the approach that NTVDM takes to display graphics once a DOS application switches to graphics mode.
Solution 4
As Nick Brooks has pointed out, you can use GDI calls in console apps, but the graphics cannot appear in the same window as the text console I/O. This may not matter since you can draw text elements in GDI.
A simplified interface to GDI calls in console apps is provided by WinBGIm. It is a clone of Borland's DOS BGI API, but with extensions to handle resizable windows, mouse input, and 24bit colour models. Since it is available as source code, it also serves a good demonstration of using GDI in this way.
It is possible to either have both a console and the GDI window, or you can suppress the console window by specifying that the application is a GUI app (the -mwindows linker option in GNU toolchain) - note that specifying a GUI app really only suppresses the console, it is only really a GUI app if it has a message loop. Having the console is good for debugging, since it is where stdout and stderr are output to by default.
Related videos on Youtube
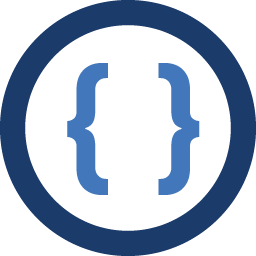
Admin
Updated on May 13, 2021Comments
-
Admin about 3 years
What is the best way to draw things in the Console Window on the Win 32 platform using C++?
I know that you can draw simple art using symbols but is there a way of doing something more complex like circles or even bitmaps?
-
Admin over 14 yearsthen how did they make those old DOS games in console?
-
Admin over 14 yearsThe console back then was not the same console as it is now.
-
littlebroccoli over 14 yearsDOS games did not use the Win32 Console, they wrote graphics directly to video memory.
-
Goz over 14 yearsPS: REad up about Mode 13h ... you'll probably find most info on that :)
-
Kristina Brooks over 14 yearsyou're stuck with GDI buddy :)
-
Dmytro almost 7 yearsactually, now i'm curious if it is possible to generate client sized frames, jigsaw each block into its own font, and fill screens of those jigsaw blocks. i'm not sure if its possible to register/unregister fonts with cmd on the fly though. Would be quite amusing if it's possible though(although it's probably more efficient and easy to just make a new window and hook the console window for resize/move to mimic it and just draw with gdi/direct3d/opengl)