Dropzone.js - Success message after Upload
Solution 1
Try with this, write your success code as follows,
success:function(file, response)
{
// Do what you want to do with your response
// This return statement is necessary to remove progress bar after uploading.
return file.previewElement.classList.add("dz-success");
}
You can refer the link, http://www.dropzonejs.com/#event-success for more details.
Solution 2
Here is how I do it, with a combination of the error
handler and queuecomplete
:
var errors = false;
var myDropzone = new Dropzone("#dropzone" , {
...
error: function(file, errorMessage) {
errors = true;
},
queuecomplete: function() {
if(errors) alert("There were errors!");
else alert("We're done!");
}
Solution 3
I had a problem similar to yours, so here is my code, i hope it can help you
Dropzone.options.UploadForm = {
method: "post",
uploadMultiple: true,
acceptedFiles: ".csv",
autoProcessQueue: false,
init: function () {
myDropzone = this;
var submitButton = document.querySelector("#submit-all");
var removeButton = document.querySelector("#remove-all");
submitButton.addEventListener("click", function () {
myDropzone.processQueue(); // Tell Dropzone to process all queued files.
});
removeButton.addEventListener("click", function () {
myDropzone.removeAllFiles();
submitButton.removeAttribute("disabled");
});
this.on("addedfile", function (file) {
});
this.on("sendingmultiple", function (file) {
// And disable the start button
submitButton.setAttribute("disabled", "disabled");
});
this.on("completemultiple", function (file) {
if (this.getUploadingFiles().length === 0 && this.getQueuedFiles().length === 0) {
submitButton.removeAttribute("disabled");
}
});
this.on("successmultiple", function (file, response) {
console.log(response);
$(response).each(function (index, element) {
if (element.status) {
$("body").prepend('<div class="alert alert-success alert-dismissable">' +
'<a href="#" class="close" data-dismiss="alert" aria-label="close">×</a>' +
'<strong>Success! </strong> ' + element.fileName + ' was uploaded successfully</div>');
}
else {
$("body").prepend('<div class="alert alert-danger alert-dismissable">' +
'<a href="#" class="close" data-dismiss="alert" aria-label="close">×</a>' +
'<strong>Error!</strong> ' + element.message + '</div >');
}
});
submitButton.removeAttribute("disabled");
});
this.on("error", function (file, errorMessage) {
$("body").prepend('<div class="alert alert-danger alert-dismissable">' +
'<a href="#" class="close" data-dismiss="alert" aria-label="close">×</a>' +
'<strong>Error!</strong> ' + errorMessage + '</div >');
submitButton.removeAttribute("disabled");
});
}
};
sendingmultiple,completemultiple and successmultiple are made for multiple file upload.
Solution 4
Does this help?
Dropzone.options.myDropzone = {
init: function() {
this.on("success", function(file, responseText) {
//
});
}
};
This link might be helpful as well: https://github.com/enyo/dropzone/issues/244
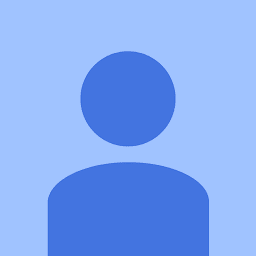
Christoph C.
Updated on February 21, 2020Comments
-
Christoph C. about 4 years
I am using Dropzone.js for my file uploading process. I already found a few topics here on stackoverflow regarding my problem but none of those solutions help me.
Right now a user can upload multiple files at once at as soon as one file is uploaded, there is a link called "Remove File". That´s all! What I want is the following:
If a user uploads let´s say 4 images than after all those images are uploaded there should be a success message. Right now a user does not understand if those files are uploaded for 100%. I am not good at jQuery/Ajax so I really do not know what my code should look like. Would be great if someone can tell me what my code should look like so that it works.
Here is my form:
print "<div class='col-sm-12'><br /><br />"; print "<form method='post' action='index.php' id='dropzone' class='form-horizontal dropzone'>"; print "<input type='hidden' name='func' value='supportticket'>"; print "<input type='hidden' name='id' value='".$id."'>"; print "<input type='hidden' name='sid' value='".$sid."'>"; print "<input type='hidden' name='attach_images' value='".$attach_images."'>"; print "<div class='form-group'>"; print "<div class='col-sm-8'>"; print "<div class='fallback'>"; print "<input name='file' type='file' multiple='' />"; print "</div>"; print "</div>"; print "</div>"; print "</form>"; print "</div>";
Here is my script:
<script type="text/javascript"> jQuery(function($){ try { Dropzone.autoDiscover = false; var myDropzone = new Dropzone("#dropzone" , { paramName: "file", // The name that will be used to transfer the file maxFilesize: 2.0, // MB addRemoveLinks : true, dictDefaultMessage : '<span class="bigger-150 bolder"><i class="ace-icon fa fa-caret-right red"></i> Drop files</span> to upload \ <span class="smaller-80 grey">(or click)</span> <br /> \ <i class="upload-icon ace-icon fa fa-cloud-upload blue fa-3x"></i>', dictResponseError: 'Error while uploading file!', //change the previewTemplate to use Bootstrap progress bars previewTemplate: "<div class=\"col-sm-4\"><div class=\"dz-preview dz-file-preview\">\n <div class=\"dz-details\">\n <div class=\"dz-filename\"><span data-dz-name></span></div>\n <div class=\"dz-size\" data-dz-size></div>\n <img data-dz-thumbnail />\n </div>\n <div class=\"progress progress-small progress-striped active\"><div class=\"progress-bar progress-bar-success\" data-dz-uploadprogress><br /></div></div>\n</div>\n <div class=\"dz-error-message\"><span data-dz-errormessage></span></div>\n</div></div>" }); $(document).one('ajaxloadstart.page', function(e) { try { myDropzone.destroy(); } catch(e) {} }); } catch(e) { alert('Dropzone.js does not support older browsers!'); } }); </script>
NOTICE: Upload is working fine currently! All images are uploaded to my server and I also wrote a script so that the name of my file will be saved inside my database. The only thing I need is to extend my script so that I get a success message if an upload was successfull.
Hope that someone can help me out!
EDIT: Here is my current code. Upload is still working fine, but no success message after all files are uploaded.
<script type="text/javascript"> jQuery(function($){ try { Dropzone.autoDiscover = false; var errors = false; var myDropzone = new Dropzone("#dropzone" , { paramName: "file", // The name that will be used to transfer the file maxFilesize: 2.0, // MB error: function(file, errorMessage) { errors = true; }, queuecomplete: function() { if(errors) alert("There were errors!"); else alert("We're done!"); }, addRemoveLinks : true, dictDefaultMessage : '<span class="bigger-150 bolder"><i class="ace-icon fa fa-caret-right red"></i> Drop files</span> to upload \ <span class="smaller-80 grey">(or click)</span> <br /> \ <i class="upload-icon ace-icon fa fa-cloud-upload blue fa-3x"></i>', dictResponseError: 'Error while uploading file!', //change the previewTemplate to use Bootstrap progress bars previewTemplate: "<div class=\"col-sm-4\"><div class=\"dz-preview dz-file-preview\">\n <div class=\"dz-details\">\n <div class=\"dz-filename\"><span data-dz-name></span></div>\n <div class=\"dz-size\" data-dz-size></div>\n <img data-dz-thumbnail />\n </div>\n <div class=\"progress progress-small progress-striped active\"><div class=\"progress-bar progress-bar-success\" data-dz-uploadprogress><br /></div></div>\n</div>\n <div class=\"dz-error-message\"><span data-dz-errormessage></span></div>\n<span data-dz-suc>successfull</span></div></div>" }); $(document).one('ajaxloadstart.page', function(e) { try { myDropzone.destroy(); } catch(e) {} }); } catch(e) { alert('Dropzone.js does not support older browsers!'); } }); </script>
Thanks, Chris