Dynamic filepath in Java (writing and reading text files)
Solution 1
You can references files using relative paths like ../myfile.txt
. The base of these paths will be the directory that the Java process was started in for the command line. For Eclipse it's the root of your project, or what you've configured as the working directory under Run > Run Configurations > Arguments. If you want to see what the current directory is inside of Java, here's a trick to determine it:
File currentDir = new File("");
System.out.println(currentDir.getAbsolutePath());
Solution 2
You can use relative paths, by default they will be relative to the current directory where you are executing the Java app from.
But you can also get the user's home directory with:
String userHome = System.getProperty("user.home");
Solution 3
You can do it:
File currentDir = new File (".");
String basePath = currentDir.getCanonicalPath();
now basePath
is the path to your application folder, add it the exact dir / filename and you're good to go
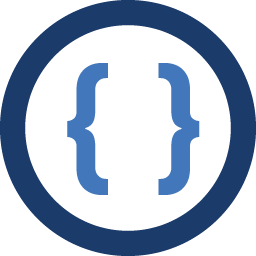
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
Okay, so I want to read a file name myfile.txt and let's say I'll be saving it under this directory:
home/myName/Documents/workspace/myProject/files myfile.txt
hmmm.. I want to know what I should pass on the
File(filePath)
as my parameter... Can I put something like "..\myfile.txt"? I don't want to hard code the file path, because, it will definitely change if say I open my project on another PC. How do i make sure that the filepath is as dynamic as possible? By the way, I'm using java.File teacherFile = new File(filePath);