Dynamically Load a user control (ascx) in a asp.net website
Solution 1
Assuming the control exists in the same assembly as your web project, you need to add a reference directive in your .aspx file,
e.g:
<%@ Reference Control="~/Controls/WebControl1.ascx">
Keep in mind it often takes a few minutes (or sometimes a build) for IntelliSense to pick this up.
Solution 2
It can easily be done using namespaces. Here's an example:
WebControl1.ascx:
<%@ Control Language="C#" AutoEventWireup="true" CodeFile="WebControl1.ascx.cs" Inherits="MyUserControls.WebControl1" %>
Notice that Inherits references the namespace (MyUserControls), and not just the class name (WebControl1)
WebControl1.ascx.cs:
namespace MyUserControls
{
public partial class WebControl1 : System.Web.UI.UserControl
{
protected void Page_Load(object sender, EventArgs e)
{
}
}
}
Notice that the class have been included in the namespace MyUserControls
Default.aspx.cs:
using MyUserControls;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
var control = (WebControl1) Page.LoadControl("~/WebControl1.ascx");
}
}
This approach potentially allow you to redistribute your user controls (or keep them in a separate project) without the hassle of referencing them in your .aspx files.
Solution 3
Namespaces are not supported under the website model. As such, I could not get any of the solutions proposed to work. However, there is a solution. Create an interface and place it into app code and then implement the interface in the user control. You can cast to the interface and it works.
Solution 4
The subject of this post is a bit misleading. If you just want to add a control dynamically, you will not have to reference the control and therefore you can just add it with something simple like:
protected void Page_Load(object sender, EventArgs e)
{
Page.Controls.Add(Page.LoadControl("~/MyControl.ascx"));
}
without any namespace hassel.
Solution 5
Casting the user control this way may create many problems .my approach is to create a class (say control Class) put all the properties and method you need for casting in it and inherit this class from System.Web.UI.UserControl .Then in your user cotrol code file instead of System.Web.UI.UserControl user this control class .
now when ever you need casting, cast with this class only . it will be light casting as well.
Related videos on Youtube
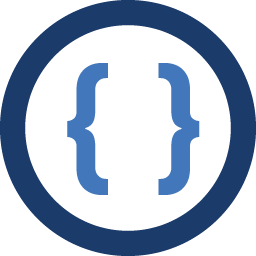
Admin
Updated on June 18, 2020Comments
-
Admin about 4 years
I am trying to dynamically load a user control in an asp.web site. However due to how asp.net websites projects are setup (I think), I am not able to access reach the type definition of the user control.
I get a message saying that my class HE_ContentTop_WebControl1 is: he type or namespace name 'HE_ContentTop_WebControl1' could not be found (are you missing a using directive or an assembly reference?)
Any idea how this could be made to work ? I have attempted using namespace but it seems to me that asp.net websites are not designed to work with namespaces by default. I would be interested in a non namespace approach.
TIA
public partial class HE_Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { var control = (HE_ContentTop_WebControl1)Page.LoadControl("~/ContentTop/WebControl1.ascx"); } }
-
JB King almost 15 yearsDon't forget that you also have to put that loaded control somewhere on the page,e.g. a placeholder.
-
-
Admin almost 15 yearsThanks very much. I had assumed the reference was not needed if I pointed to the location of the code file. It seemed a little redundant to add the file but again I made an assumption...
-
Tchami almost 15 yearsIf you're dealing with a lot of usercontrols I really wouldn't recommend this approach. In my experience the solution to add the usercontrols to a namespace and then load them dynamically is a lot more versatile.
-
jscharf almost 15 yearsYeah, it's a bit redundant but basically the reference directive is for ASP.NET so it can actually resolve the cast on Page.LoadControl at the appropriate time. Glad I could help!
-
Inderpal Singh almost 11 yearsWhere to put this ascx file? If i put it any where i can't import a name space unless i put in app_code directory. If i put in app_code directory it then gives me a error you can't put it there
-
Tchami almost 11 yearsWhat do you mean you can't import a namespace?
-
Inderpal Singh almost 11 yearsname space MyUserControls .. Where to put ascx file(in appcode or bin). I put it in controls folder but it doesn't recognize name space MyUserControls.
-
Kyle almost 10 yearsUpvoted because this solves the problem in Visual Studio AND MSBuild.
-
Shivam Srivastava over 9 yearsAdd enclosed tag <%@ Reference Control="~/Controls/WebControl1.ascx" %>
-
Magnus over 6 yearsThanks, this helped me implement Tchami's solution in a "website" I work on. As you said, I could not reference the class first, but with your explanation and suggestion of an interface, it now worked perfectly!