Edit MYSQL Row in PHP form
Solution 1
edit.php - with some changes
<?php
mysql_connect('localhost', 'admin', 'passw0rd') or die(mysql_error());
mysql_select_db("students") or die(mysql_error());
$UID = (int)$_GET['ID'];
$query = mysql_query("SELECT * FROM stokesley_students WHERE id = '$UID'") or die(mysql_error());
if(mysql_num_rows($query)>=1){
while($row = mysql_fetch_array($query)) {
$firstname = $row['firstname'];
$surname = $row['surname'];
$FBID = $row['FBID'];
$IMGNU = $row['IMGNU'];
}
?>
<form action="update.php" method="post">
<input type="hidden" name="ID" value="<?=$UID;?>">
IMGNU: <input type="text" name="ud_img" value="<?=$IMGNU;?>"><br>
First Name: <input type="text" name="ud_firstname" value="<?=$firstname?>"><br>
Last Name: <input type="text" name="ud_surname" value="<?=$surname?>"><br>
FB: <input type="text" name="ud_FBID" value="<?=$FBID?>"><br>
<input type="Submit">
</form>
<?php
}else{
echo 'No entry found. <a href="javascript:history.back()">Go back</a>';
}
?>
update.php (Apart from the asterisk, Your query was also matching ID
with the $ud_IMG
variable)
<?php
mysql_connect('localhost', 'admin', 'passw0rd') or die(mysql_error());
mysql_select_db("students") or die(mysql_error());
$ud_ID = (int)$_POST["ID"];
$ud_firstname = mysql_real_escape_string($_POST["ud_firstname"]);
$ud_surname = mysql_real_escape_string($_POST["ud_surname"]);
$ud_FBID = mysql_real_escape_string($_POST["ud_FBID"]);
$ud_IMG = mysql_real_escape_string($_POST["ud_IMG"]);
$query="UPDATE stokesley_students
SET firstname = '$ud_firstname', surname = '$ud_surname', FBID = '$ud_FBID'
WHERE ID='$ud_ID'";
mysql_query($query)or die(mysql_error());
if(mysql_affected_rows()>=1){
echo "<p>($ud_ID) Record Updated<p>";
}else{
echo "<p>($ud_ID) Not Updated<p>";
}
?>
Solution 2
"UPDATE * stokesley_students SET firstname = '$ud_firstname', surname = '$ud_surname',
FBID = '$ud_FBID' WHERE ID ='$ud_IMG'"
That query is wrong, remove the asterisk. Also, you don't know if there is an error because you don't check the return type of mysql_query
or use mysql_error
.
Solution 3
The update query is wrong. You need to tell it which table then which fields specifically. The asterisk is only used on select statements.
Also it would be helpful if you checked for whether or not the query was successful. Take a look below. I have rewritten your code a bit. I also allowed for the ID to come from the POST or the GET by using REQUEST. And I removed the mysql_close() call since it is completely unneeded as it will be closed when the script stops running.
<?php
$ud_ID = $_REQUEST["ID"];
$ud_firstname = $_POST["ud_firstname"];
$ud_surname = $_POST["ud_surname"];
$ud_FBID = $_POST["ud_FBID"];
$ud_IMG = $_POST["ud_IMG"];
mysql_connect('localhost', 'admin', 'passw0rd') or die(mysql_error());
echo "MySQL Connection Established! <br>";
mysql_select_db("students") or die(mysql_error());
echo "Database Found! <br>";
$query = "UPDATE stokesley_students SET firstname = '$ud_firstname', surname = '$ud_surname',
FBID = '$ud_FBID' WHERE ID = '$ud_ID'";
$res = mysql_query($query);
if ($res)
echo "<p>Record Updated<p>";
else
echo "Problem updating record. MySQL Error: " . mysql_error();
?>
Quick little reference on the PHP mysql_query function: http://nl.php.net/manual/en/function.mysql-query.php
Also I bet you would like a few good tutorials to help you out learning PHP and MySQL. Check out this site: http://net.tutsplus.com/category/tutorials/php/
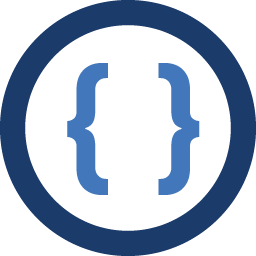
Admin
Updated on March 02, 2020Comments
-
Admin about 4 years
I have done this before and for some reason I just can't get it to work this time! I'm pulling my hair out over this! Because there is no errors, it just wont update the database.
Basically I Have a Table with student data in....
ID | IMGNU | Firstname | Surname | FBID
Let's use row 233 for an example.
I can view a specific row by going to view.php?ID=233
Then that works, but now i want to be able to go to edit.php?ID=233 and it should load a form, that already has the info from row 233. I should then be able to edit the data in the fields and submit the form, which would change the information in the database.
Here is what i have already.
edit.php
<?php mysql_connect('localhost', 'admin', 'passw0rd') or die(mysql_error()); echo "Tick <p>"; mysql_select_db("students") or die(mysql_error()); echo "Tick"; $UID = $_GET['ID']; $query = mysql_query("SELECT * FROM stokesley_students WHERE id = '$UID'") or die(mysql_error()); while($row = mysql_fetch_array($query)) { echo ""; $firstname = $row['firstname']; $surname = $row['surname']; $FBID = $row['FBID']; $IMGNU = $row['IMGNU']; }; ?> <form action="update.php?ID=<?php echo "$UID" ?>" method="post"> IMGNU: <input type="text" name="ud_img" value="<?php echo "$IMGNU" ?>"><br> First Name: <input type="text" name="ud_firstname" value="<?php echo "$firstname" ?>"><br> Last Name: <input type="text" name="ud_surname" value="<?php echo "$surname" ?>"><br> FB: <input type="text" name="ud_FBID" value="<?php echo "$FBID" ?>"><br> <input type="Submit"> </form>
And here is update.php
< ?php $ud_ID = $_GET["ID"]; $ud_firstname = $_POST["ud_firstname"]; $ud_surname = $_POST["ud_surname"]; $ud_FBID = $_POST["ud_FBID"]; $ud_IMG = $_POST["ud_IMG"]; mysql_connect('localhost', 'admin', 'passw0rd') or die(mysql_error()); echo "MySQL Connection Established! <br>"; mysql_select_db("students") or die(mysql_error()); echo "Database Found! <br>"; $query="UPDATE * stokesley_students SET firstname = '$ud_firstname', surname = '$ud_surname', FBID = '$ud_FBID' WHERE ID ='$ud_IMG'"; mysql_query($query); echo "<p>Record Updated<p>"; mysql_close(); ?>
Any ideas would be much appreciated, maby im just missing something stupid?
Thanks Alex