Editing 8bpp indexed Bitmaps
Solution 1
There is no need to move into C++ land or use P/Invoke at all; C# supports pointers and unsafe code perfectly fine; I may even hazard a guess that this is causing your problems.
The colors are likely coming from the default Palette. You'll find that changing the Palette shouldn't be slow at all. This is what I do to create a greyscale palette:
image = new Bitmap( _size.Width, _size.Height, PixelFormat.Format8bppIndexed);
ColorPalette pal = image.Palette;
for(int i=0;i<=255;i++) {
// create greyscale color table
pal.Entries[i] = Color.FromArgb(i, i, i);
}
image.Palette = pal; // you need to re-set this property to force the new ColorPalette
Solution 2
Imagine that your indexed 8ppp graysacle are stored in a linear array dataB (for speed)
Try this code:
//Create 8bpp bitmap and look bitmap data
bmp = new Bitmap(width, height, PixelFormat.Format8bppIndexed);
bmp.SetResolution(horizontalResolution, verticalResolution);
bmpData = bmp.LockBits(new Rectangle(0, 0, width, height), System.Drawing.Imaging.ImageLockMode.WriteOnly, PixelFormat.Format8bppIndexed);
//Create grayscale color table
ColorPalette palette = bmp.Palette;
for (int i = 0; i < 256; i++)
palette.Entries[i] = Color.FromArgb(i, i, i);
bmp.Palette = palette;
//write data to bitmap
int dataCount = 0;
int stride = bmpData.Stride < 0 ? -bmpData.Stride : bmpData.Stride;
unsafe
{
byte* row = (byte*)bmpData.Scan0;
for (int f = 0; f < height; f++)
{
for (int w = 0; w < width; w++)
{
row[w] = (byte)Math.Min(255, Math.Max(0, dataB[dataCount]));
dataCount++;
}
row += stride;
}
}
//Unlock bitmap data
bmp.UnlockBits(bmpData);
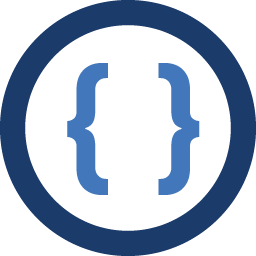
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
i'm trying to edit the pixels of a 8bpp. Since this PixelFormat is indexed i'm aware that it uses a Color Table to map the pixel values. Even though I can edit the bitmap by converting it to 24bpp, 8bpp editing is much faster (13ms vs 3ms). But, changing each value when accessing the 8bpp bitmap results in some random rgb colors even though the PixelFormat remains 8bpp.
I'm currently developing in c# and the algorithm is as follows:
(C#)
1- Load original Bitmap at 8bpp
2- Create Empty temp Bitmap with 8bpp with the same size as the original
3-LockBits of both bitmaps and, using P/Invoke, calling c++ method where I pass the Scan0 of each BitmapData object. (I used a c++ method as it offers better performance when iterating through the Bitmap's pixels)
(C++)
4- Create a int[256] palette according to some parameters and edit the temp bitmap bytes by passing the original's pixel values through the palette.
(C#)
5- UnlockBits.
My question is how can I edit the pixel values without having the strange rgb colors, or even better, edit the 8bpp bitmap's Color Table?
-
Admin about 14 yearsThanks for your answer. I know I can modify the bitmap's palette by changing the Bitmap's Palette property. But doing this is too slow. I was hoping that someone could tell me how to access the Color Table pointer so that I could modify it directly. SetPixel does not work and is also very sluggish. what i don't understand is why the pixel is assigned with a rgb color when i change it's value from 0 (black) to 100 (should be gray but is red).
-
Leon almost 12 yearsHow do I apply the new palette to the old image? How about changing the size of the old image as well?
-
Dai almost 11 yearsJust set the
Palette
property back. Resizing an image is different, useGraphics.DrawImage
on a new image of the right dumensions for that. -
marknuzz about 8 yearsGraphics.DrawImage is not supported on indexed pixel formats, and converting to non-indexed negates the speed benefit of using indexed formats.
-
Nyerguds over 6 yearsUm.
Bitmap
inherits fromImage
... and thus has that same Palette. And you can copy palettes simply withbm2.Palette = bm1.Palette
. -
Nyerguds over 4 yearsIf you want resizing on indexed images in c#, you're just going to have to write algorithms yourself based on handling raw byte arrays, and import the result into a new bitmap using LockBits. Or resize in hi-colour and then match the pixels back to their closest palette match.
-
Dai over 4 years@nyerguds oh hai from C&C :3