EEPROM emulation on stm32 using HAL drivers
ST Microelectronics did provide sample code using HAL drivers. The problem is their documentation is all over the place and googling may not land you the right page.
This is the right document for you.
Search for "EEPROM_Emulation". You will find that the sample code is provided under NUCLEO -F091RC firmware examples. The sample code should answer your questions.
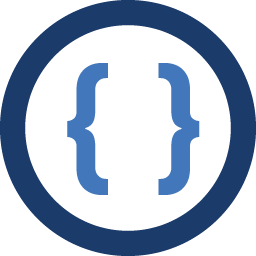
Admin
Updated on July 06, 2020Comments
-
Admin almost 4 years
I am trying to emulate EEPROM on stm32f0. There is an application note provided by STM.
In the sample
main.c
,int main(void) { /*!< At this stage the microcontroller clock setting is already configured, this is done through SystemInit() function which is called from startup file (startup_stm32f0xx.s) before to branch to application main. To reconfigure the default setting of SystemInit() function, refer to system_stm32f0xx.c file */ /* Unlock the Flash Program Erase controller */ FLASH_Unlock(); /* EEPROM Init */ EE_Init(); /* --- Store successively many values of the three variables in the EEPROM ---*/ /* Store 0x1000 values of Variable1 in EEPROM */ for (VarValue = 1; VarValue <= 0x64; VarValue++) { EE_WriteVariable(VirtAddVarTab[0], VarValue); } /* read the last stored variables data*/ EE_ReadVariable(VirtAddVarTab[0], &VarDataTab[0]); /* Store 0x2000 values of Variable2 in EEPROM */ for (VarValue = 1; VarValue <= 0xC8; VarValue++) { EE_WriteVariable(VirtAddVarTab[1], VarValue); } /* read the last stored variables data*/ EE_ReadVariable(VirtAddVarTab[0], &VarDataTab[0]); EE_ReadVariable(VirtAddVarTab[1], &VarDataTab[1]); /* Store 0x3000 values of Variable3 in EEPROM */ for (VarValue = 1; VarValue <= 0x1C2; VarValue++) { EE_WriteVariable(VirtAddVarTab[2], VarValue); } /* read the last stored variables data*/ EE_ReadVariable(VirtAddVarTab[0], &VarDataTab[0]); EE_ReadVariable(VirtAddVarTab[1], &VarDataTab[1]); EE_ReadVariable(VirtAddVarTab[2], &VarDataTab[2]); while (1); }
Flash_Unlock()
is a function used in STM standard peripheral library. However, I am using CubeMX that auto-generates code that uses HAL drivers. IsFlash_Unlock()
necessary to be called before one can use the EEPROM_emulation APIs? If yes, what is the HAL equivalent for callingFlash_Unlock()
? Any special configuration settings to make on CubeMX to use EEPROM emulation?