Elegant way to perform tuple arithmetic
Solution 1
If you're looking for fast, you can use numpy:
>>> import numpy
>>> numpy.subtract((10, 10), (4, 4))
array([6, 6])
and if you want to keep it in a tuple:
>>> tuple(numpy.subtract((10, 10), (4, 4)))
(6, 6)
Solution 2
One option would be,
>>> from operator import sub
>>> c = tuple(map(sub, a, b))
>>> c
(6, 6)
And itertools.imap
can serve as a replacement for map
.
Of course you can also use other functions from operator
to add
, mul
, div
, etc.
But I would seriously consider moving into another data structure since I don't think this type of problem is fit for tuple
s
Solution 3
Use zip
and a generator expression:
c = tuple(x-y for x, y in zip(a, b))
Demo:
>>> a = (10, 10)
>>> b = (4, 4)
>>> c = tuple(x-y for x, y in zip(a, b))
>>> c
(6, 6)
Use itertools.izip
for a memory efficient solution.
help on zip
:
>>> print zip.__doc__
zip(seq1 [, seq2 [...]]) -> [(seq1[0], seq2[0] ...), (...)]
Return a list of tuples, where each tuple contains the i-th element
from each of the argument sequences. The returned list is truncated
in length to the length of the shortest argument sequence.
Solution 4
JFYI, execution time in my laptop in 100000 times iteration
np.subtract(a, b)
: 0.18578505516052246
tuple(x - y for x, y in zip(a, b))
:
0.09348797798156738
tuple(map(lambda x, y: x - y, a, b))
: 0.07900381088256836
from operator import sub tuple(map(sub, a, b))
: 0.044342041015625
operator looks more elegant for me.
Solution 5
This can also be done just as nicely without an import at all, although lambda is often undesirable:
tuple(map(lambda x, y: x - y, a, b))
If you are looking to get the distance between two points on say a 2d coordinate plane you should use the absolute value of the subtraction of the pairs.
tuple(map(lambda x ,y: abs(x - y), a, b))
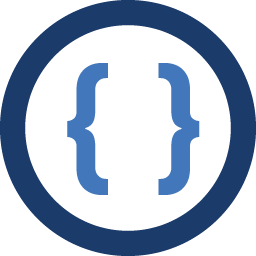
Admin
Updated on May 08, 2020Comments
-
Admin almost 4 years
What is the most elegant and concise way (without creating my own class with operator overloading) to perform tuple arithmetic in Python 2.7?
Lets say I have two tuples:
a = (10, 10) b = (4, 4)
My intended result is
c = a - b = (6, 6)
I currently use:
c = (a[0] - b[0], a[1] - b[1])
I also tried:
c = tuple([(i - j) for i in a for j in b])
but the result was
(6, 6, 6, 6)
. I believe the above works as a nested for loops resulting in 4 iterations and 4 values in the result. -
Admin almost 11 yearsThanks for the detailed answer!
-
Arthur Tacca over 6 yearsJust a note on
izip
: In Python 3, the regularzip
function works like Python 2'sizip
did, so you can just usezip
as the examples in this answer show. -
vroomfondel almost 5 yearsyou definitely shouldn't use np.subtract if you're converting to and from numpy arrays, but should if you're doing many of these and can keep them in the more reasonable data structure for this task!