Email validation using jQuery
Solution 1
You can use regular old javascript for that:
function isEmail(email) {
var regex = /^([a-zA-Z0-9_.+-])+\@(([a-zA-Z0-9-])+\.)+([a-zA-Z0-9]{2,4})+$/;
return regex.test(email);
}
Solution 2
jQuery Function to Validate Email
I really don’t like to use plugins, especially when my form only has one field that needs to be validated. I use this function and call it whenever I need to validate an email form field.
function validateEmail($email) {
var emailReg = /^([\w-\.]+@([\w-]+\.)+[\w-]{2,4})?$/;
return emailReg.test( $email );
}
and now to use this
if( !validateEmail(emailaddress)) { /* do stuff here */ }
Cheers!
Solution 3
I would use the jQuery validation plugin for a few reasons.
You validated, ok great, now what? You need to display the error, handle erasing it when it is valid, displaying how many errors total perhaps? There are lots of things it can handle for you, no need to re-invent the wheel.
Also, another huge benefit is it's hosted on a CDN, the current version at the time of this answer can be found here: http://www.asp.net/ajaxLibrary/CDNjQueryValidate16.ashx This means faster load times for the client.
Solution 4
Look at http: //bassistance.de/jquery-plugins/jquery-plugin-validation/. It is nice jQuery plugin, which allow to build powerfull validation system for forms.
There are some usefull samples here. So, email field validation in form will look so:
$("#myform").validate({
rules: {
field: {
required: true,
email: true
}
}
});
See Email method documentation for details and samples.
Solution 5
<script type="text/javascript">
$(document).ready(function() {
$('.form_error').hide();
$('#submit').click(function(){
var name = $('#name').val();
var email = $('#email').val();
var phone = $('#phone').val();
var message = $('#message').val();
if(name== ''){
$('#name').next().show();
return false;
}
if(email== ''){
$('#email').next().show();
return false;
}
if(IsEmail(email)==false){
$('#invalid_email').show();
return false;
}
if(phone== ''){
$('#phone').next().show();
return false;
}
if(message== ''){
$('#message').next().show();
return false;
}
//ajax call php page
$.post("send.php", $("#contactform").serialize(), function(response) {
$('#contactform').fadeOut('slow',function(){
$('#success').html(response);
$('#success').fadeIn('slow');
});
});
return false;
});
});
function IsEmail(email) {
var regex = /^([a-zA-Z0-9_\.\-\+])+\@(([a-zA-Z0-9\-])+\.)+([a-zA-Z0-9]{2,4})+$/;
if(!regex.test(email)) {
return false;
}else{
return true;
}
}
</script>
<form action="" method="post" id="contactform">
<table class="contact-table">
<tr>
<td><label for="name">Name :</label></td>
<td class="name"> <input name="name" id="name" type="text" placeholder="Please enter your name" class="contact-input"><span class="form_error">Please enter your name</span></td>
</tr>
<tr>
<td><label for="email">Email :</label></td>
<td class="email"><input name="email" id="email" type="text" placeholder="Please enter your email" class="contact-input"><span class="form_error">Please enter your email</span>
<span class="form_error" id="invalid_email">This email is not valid</span></td>
</tr>
<tr>
<td><label for="phone">Phone :</label></td>
<td class="phone"><input name="phone" id="phone" type="text" placeholder="Please enter your phone" class="contact-input"><span class="form_error">Please enter your phone</span></td>
</tr>
<tr>
<td><label for="message">Message :</label></td>
<td class="message"><textarea name="message" id="message" class="contact-input"></textarea><span class="form_error">Please enter your message</span></td>
</tr>
<tr>
<td></td>
<td>
<input type="submit" class="contactform-buttons" id="submit"value="Send" />
<input type="reset" class="contactform-buttons" id="" value="Clear" />
</td>
</tr>
</table>
</form>
<div id="success" style="color:red;"></div>
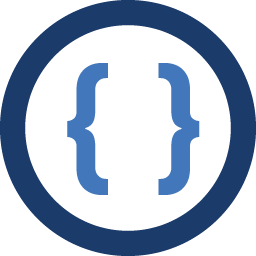
Admin
Updated on July 29, 2021Comments
-
Admin almost 3 years
I'm new to jQuery and was wondering how to use it to validate email addresses.
-
Fabian about 14 yearsYes it will, remember jQuery is still javascript :)
-
Dave Hogan over 11 yearsCan you elaborate your answer... for example you should mention that getVerimailStatus is an additional plugin.
-
Arseny over 11 yearsEven though this regexp considers most real world addresses valid, it still has a lot of false positives and false negatives. For instance, see examples of valid and invalid email addresses on Wikipedia.
-
Benjamin Eckstein almost 11 yearswith this regex ... would [email protected] be a valid email?
-
tomis almost 11 years@Umingo [email protected] is still valid e-mail, however, it still could be written in better way. None part of domain can start with other char than [a-z0-9] (case insensitive). Also, valid e-mail (and domain) has some len limit, which is also not tested.
-
nffdiogosilva over 10 yearsYou should just return
emailReg.test($email);
-
Diziet over 10 yearsJust FYI this returns true for a blank email address. e.g.
emailReg.text("")
true
. I'd simply the function down to the declaration of the emailReg var then this:return ( $email.length > 0 && emailReg.test($email))
-
Theo about 10 yearsThe regex for checking the emailaddress validity is outdated since we now have domainname extensions with 6 characters like .museum, therefor you would want to change
var emailReg = /^([\w-\.]+@([\w-]+\.)+[\w-]{2,4})?$/;
tovar emailReg = /^([\w-\.]+@([\w-]+\.)+[\w-]{2,6})?$/;
-
kraftb about 10 yearsOk ... no need to reinvent the wheel. But why do I have to install dozens of KByte of Javascript for validating a field. It's like building a car factory if all you need is a new wheel :)
-
Nick Craver about 10 years@kraftb As stated in my answer, it's the handling and display around the validation, not just validating the text itself.
-
iGanja about 10 yearsright you are @h.coates! I came to this thread hoping to find that jQuery actually had a built in email validation. Move along, these aren't the droids you are looking for...
-
Andrew Bashtannik about 10 yearsThe last one is still alive)
-
gkanak about 10 years
.getVerimailStatus()
doesn't return numeric status codes, just a string value ofsuccess
,error
or possiblypending
(didn't verify the last one). -
Imdad almost 10 yearsThis would pass [email protected] as a valid email but the tld should be 2-4 characters. A better suggestion is /^([a-zA-Z0-9_.+-])+\@(([a-zA-Z0-9-])+\.)+([a-zA-Z0-9]){2,4}$/ But of coursr this can be further improved...
-
jfgrissom almost 10 yearsThanks for this @NickCraver: This really looks to be a "best practice" approach to the problem of handling validation for an email. This most certainly is NOT like building a factory (writing up the libs to do all the work) to get a wheel. It's like following the instructions from the factory to install the wheel on a modern vehicle (jack the car up, place the wheel - put on the lug nuts) instead of trying to figure out how to get a wagon wheel on your car. This plugin is super simple to use. To do the form validation it's literally an include, some annotations, and a single method call.
-
Liath over 9 yearsWith new top level domains becoming more common this regex may need modifying .systems and .poker etc are all valid TLDs now but would fail the regex check
-
SnowInferno over 9 yearsThe regexp method usually prevents clearly silly emails like [email protected] (which your linked JSFiddle example allows using latest Chrome), so the HTML5 solution is clearly an inadequate solution.
-
isaac weathers over 9 yearscool. So how about just using the pattern match like HTML5 is "supposed" to do? Why don't you try this in your chromebook: jsfiddle.net/du676/8
-
icktoofay over 9 yearsBut are successive dots actually invalid? On the contrary I think you’d be excluding valid email addresses by doing that.
-
Jeroenv3 almost 9 years@Theo's point is vital, but the actual length of the TLD should be more than 6, the theoretical upper limit for the extension is 63 characters. currently the longest one is over 20 see data.iana.org/TLD/tlds-alpha-by-domain.txt
-
Adam Knights almost 9 yearsThis one was the best answer for me - it returned true on
[email protected]
but would like that to have been false -
Dom Vinyard almost 9 yearsNow you're reinventing the 'reinventing the wheel' metaphor!
-
Anders over 8 yearsStack Overflow is a site in english. Please do not post content in other languages.
-
Jerreck over 8 yearsFor people stuck working on webforms apps encosia.com/using-jquery-validation-with-asp-net-webforms
-
Basheer AL-MOMANI over 7 yearsbut the accepted format is
x@x
(thats weird) it must[email protected]
How can i fix that? -
Bill Gillingham over 7 years@BasheerAL-MOMANI [jqueryvalidation.org/jQuery.validator.methods/]
$.validator.methods.email = function( value, element ) { return this.optional( element ) || //^.+@.+\..+$/.test( value ); }
-
Trevor Nestman over 7 years@nbrogi What do you mean this doesn't work? I just checked this again and this produces the following js
var regex = /^([a-zA-Z0-9_.+-])+\@(([a-zA-Z0-9-])+\.)+([a-zA-Z0-9]{2,4})+$/;
What's happening to your code? -
nkkollaw over 7 yearsSorry, I'm not sure at the moment, I completely changed it.
-
Trevor Nestman over 7 yearsPlease let me know when you can. If this is bad information, then I'll take it down. I try to contribute helpful information when possible and this helped me when writing a regex in an MVC view.
-
Trevor Nestman over 7 yearsAgain, I'd like to know why this was downvoted. It does exactly what I'm wanting it to which is produce the
@
symbol in a regex in.cshtml
. Normally it would try to treat everything after the@
symbol as razor code, but the double@@
prevents that. -
Rosdi Kasim almost 7 yearsIf you using this in ASP.NET MVC Razor page, don't forget to escape the
@
character with another@
character. Like so@@
, otherwise you will get build error. -
retrovertigo over 6 yearsjqvalidate changed the pattern in 1.12 based on html.spec.whatwg.org/multipage/input.html#valid-e-mail-address
-
Ira Herman almost 6 yearsPer Theo's comment on another answer, you should change
var regex = /^([a-zA-Z0-9_.+-])+\@(([a-zA-Z0-9-])+\.)+([a-zA-Z0-9]{2,4})+$/;
tovar regex = /^([a-zA-Z0-9_.+-])+\@(([a-zA-Z0-9-])+\.)+([a-zA-Z0-9]{2,6})+$/;
to support the newer TLD's like .museum, etc -
phlare almost 6 yearsmain issue i see is in the second codeblock your regex is set to a variable named regex, but second line uses a variable named re
-
Trevor Nestman almost 6 years@phlare Nice catch. Thanks.
-
Sandeep Patel about 5 yearsIt returns true for blank input.
-
Paras Korat about 5 yearsadd description of you answer.
-
DrCJones over 4 yearsTo keep up to date with the regx that will work for email addresses, you can look at emailregex.com . Currently it's
/^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/
-
Vikas Lalwani over 3 yearsgood example, I think adding jQuery validate for email validation would have made it much easier, also we can do HTML5 regex, check for possible ways: qawithexperts.com/article/jquery/…
-
mickmackusa over 3 yearsThis answer is missing its educational explanation.
-
ReverseEMF about 3 yearsThe simple distinctions is: Use the jQueryValidate plugin if want: (A) The full suite of validation functionality; (B) Something that will likely keep pace with standards, and browser quirks. Use the local regex function, if: (A) all you want to do is validate an email address, and don't want to down load a bulldozer to merely dig a 6" hole; (B) You don't mind keeping your own code up-to-date.
-
Stocki almost 2 yearsUnicode support e.g. for accented characters:
var emailReg = /^([\p{L}\p{N}\.-]+@([\p{L}\p{N}-]+\.)+[\p{L}\p{N}-]{2,6})?$/u;
. So thefőnök@árvíztűrő-tükörfúrógép.ευ
(boss@flood-resistant-mirror-drill.ευ – note the greek.ευ
TLD) will be valid. To reject empty/blank inputs use:var emailReg = /^[\p{L}\p{N}\.-]+@([\p{L}\p{N}-]+\.)+[\p{L}\p{N}-]{2,6}$/u;
(removed wrapping(...)?
).