Embedded Jetty Server - NO JSP Support for /, did not find org.apache.jasper.servlet.JspServlet
Solution 1
It seems like you're missing an JAR-file that includes the class org.apache.jasper.servlet.JspServlet
. Download a JAR file containing it (look here) and add it to your classpath. Also, on a side note, you should replace com/test/ServerMain
with the real class name, com.test.ServerMain
. You java statement should look like this:
java -cp ".:lib/servlet-api.jar:lib/jetty-all.jar:lib/apache-jasper.jar" com.test.ServerMain
Solution 2
Haven't tried for embedded Jetty but when running Jetty 9.3 as a service you need to add JSP support.
cd $JETTY_BASE
$JAVA_HOME/bin/java -jar $JETTY_HOME/start.jar --add-to-startd=jsp
Where JETTY_BASE
is your folder where you deploy application which is separate from JETTY_HOME
. So I'm guessing embedded Jetty would need similar configuration.
Solution 3
Incidentally, there's a github project, maintained by the Jetty Project, demonstrating JSP support in Jetty Embedded.
https://github.com/jetty-project/embedded-jetty-jsp
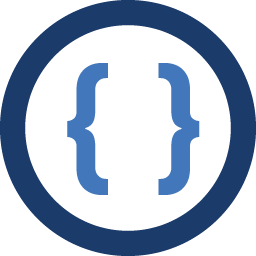
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I've have the following code to use an embedded Jetty server alongside a simple servlet and .jsp webpage. However, after compiling and running the code:
javac -cp lib/servlet-api.jar:lib/jetty-all.jar com/test/MyServlet.java javac -cp lib/servlet-api.jar:lib/jetty-all.jar com/test/ServerMain.java java -cp .:lib/servlet-api.jar:lib/jetty-all.jar com/test/ServerMain
I get an error:
INFO:oejw.StandardDescriptorProcessor:main: NO JSP Support for /, did not find org.apache.jasper.servlet.JspServlet
And navigating to /index.jsp gives a 500 error.
HTTP ERROR 500 Problem accessing /index.jsp. Reason: JSP support not configured
I've read this post but I don't think the solution applies here because I'm running Jetty embedded rather than using the start.jar.
How can I resolve this error so that the server will run and serve .jsp pages successfully?
ServerMain.java
package com.test; import org.eclipse.jetty.server.Server; import org.eclipse.jetty.webapp.WebAppContext; public class ServerMain { public static void main(String[] args) throws InterruptedException { Server server = new Server(8080); WebAppContext webApp = new WebAppContext(); webApp.setDescriptor("web.xml"); webApp.setResourceBase(""); webApp.setParentLoaderPriority(true); server.setHandler(webApp); try { server.start(); } catch (Exception e) { e.printStackTrace(); System.exit(-1); } server.join(); } }
MyServlet.java
package com.test; import java.io.IOException; import java.util.Properties; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class MyServlet extends HttpServlet { @Override public void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException { resp.setContentType("text/plain"); resp.getWriter().println("Hello, this is a testing servlet. \n\n"); Properties p = System.getProperties(); p.list(resp.getWriter()); } }
web.xml
<?xml version="1.0" encoding="utf-8"?> <!DOCTYPE web-app PUBLIC "-//Oracle Corporation//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd"> <web-app xmlns="http://java.sun.com/xml/ns/javaee" version="2.5"> <servlet> <servlet-name>MyServlet</servlet-name> <servlet-class>com.test.MyServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>MyServlet</servlet-name> <url-pattern>/test</url-pattern> </servlet-mapping> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
This is my project structure:
webapp ----com ----test ----MyServlet.java ----ServerMain.java ----index.jsp ----web.xml ----lib ----jetty-all.jar ----servlet-api.jar