Embedding Java Applet into .html file
Solution 1
Making applets work across a wide range of browsers is surprisingly hard. The tags weren't properly standardized in the early days, so Internet Explorer and Mozilla went separate directions.
Sun developed a generic JavaScript to handle all the specific browser quirks, so that you don't have to worry about browser compatibility.
Add this to your <head>
section:
<script src="//www.java.com/js/deployJava.js"></script>
And this to <body>
section:
<script>
var attributes = {codebase: 'http://my.url/my/path/to/codebase',
code: 'my.main.Applet.class',
archive: 'my-archive.jar',
width: '800',
height: '600'};
var parameters = {java_arguments: '-Xmx256m'}; // customize per your needs
var version = '1.5'; // JDK version
deployJava.runApplet(attributes, parameters, version);
</script>
See Java™ Rich Internet Applications Deployment Advice for a detailed explanation of the script and all the possible options.
Solution 2
I agree that deployJava.js
is preferred approach nowadays.
Then follow several old multi-browser tricks for historical completeness.
https://www.ailis.de/~k/archives/63-How-to-use-Java-applets-in-modern-browsers.html:
<object id="testapplet-object"
classid="clsid:8AD9C840-044E-11D1-B3E9-00805F499D93"
width="256" height="256"
codebase="http://java.sun.com/update/1.6.0/jinstall-6u30-windows-i586.cab#Version=1,6,0,0">
<param name="archive" value="mytest.jar" />
<param name="code" value="my.package.MyClass" />
<param name="myParam" value="My Param Value" />
<embed id="testapplet-embed"
type="application/x-java-applet;version=1.6"
width="256" height="256"
archive="mytest.jar"
code="my.package.MyClass"
pluginspage="http://java.com/download/"
myParam="My Param Value" />
</embed>
</object>
http://joliclic.free.fr/html/object-tag/en/object-java.html (has several variations):
<object classid="clsid:8AD9C840-044E-11D1-B3E9-00805F499D93"
width="150" height="80">
<param name="codebase" value="data" >
<param name="code" value="JitterText">
<param name="BGCOLOR" value="000000">
<param name="TEXTCOLOR" value="FF0000">
<param name="TEXT" value="OJITesting!">
<param name="SPEED" value="250">
<param name="RANDOMCOLOR" value="1">
<!--[if gte IE 7]> <!-->
<object classid="java:JitterText.class"
codebase="data"
type="application/x-java-applet"
width="150" height="80">
<param name="code" value="JitterText">
<!-- Safari browser needs the following param -->
<param name="JAVA_CODEBASE" value="data">
<param name="BGCOLOR" value="000000">
<param name="TEXTCOLOR" value="FF0000">
<param name="TEXT" value="OJITesting!">
<param name="SPEED" value="250">
<param name="RANDOMCOLOR" value="1">
alt : <a href="data/JitterText.class">JitterText.class</a>
</object>
<!--<![endif]-->
<!--[if lt IE 7]>
alt : <a href="data/JitterText.class">JitterText.class</a>
<![endif]-->
</object>
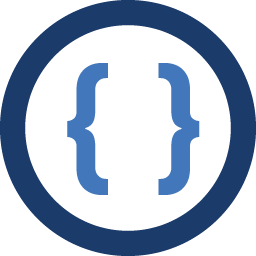
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am having trouble embedding my applet into a webpage. I don't think I'm doing it correctly.
* I have my html file in the same directory as my .class files
My main method is in CardApp class
This is my html code
<html> <head> <meta content="text/html; charset=ISO-8859-1" http-equiv="content-type"> <title>TestJCardBet.html</title> </head> <body> <applet codebase="" code="CardApp.class" height="400" width="500"></applet> </body> </html>
-
Admin over 13 yearsis code base the directory where I have my .class files? I have the .html file in the same directory as the .class files. What should I put for codebase?
-
Admin over 13 yearsAlso is archive a required attribute? I don't have a jar. Should I build one? or would it work without it?
-
Admin over 13 yearsAlso, the line var parameters. do I just leave it like that? or do I need to substitute java_arguments for my main arguments? Actually my program doesn't require any arguments to run it.
-
Admin over 13 yearsthe jdk version, is that my version on my computer? or the compliance version I used to build my project?
-
Kim Burgaard over 13 yearscodebase is the URL where either your jar file or individual class files are located. If you use a jar file, then you must use the archive property. Otherwise, omit the archive property. The JDK version is whatever version your code depends upon. So if you're developing with JDK 1.5, then specify 1.6. If you're coding with JDK 1.6, then specify 1.6.
-
Kim Burgaard over 13 yearsIf you aren't using a web server and your class files or .jar archive is placed locally on your machine, make sure you start your codebase URL with file:// instead of http://
-
Jackie Degl'Innocenti about 7 yearsIt doesn't work any more on Chrome, as it has no more support for NPAPI plugins. java.com/it/download/faq/chrome.xml