Embedding YouTube videos on
Solution 1
Just tested your code, it works fine on an iPhone, Youtube videos are not supported on the iOS simulator though, so you'll need a real device for testing.
How can I position this box?
You are already passing the X (20), Y(20), width(100) and height(100) of the box at this line:
[self embedYouTube:@"http://..." frame:CGRectMake(20, 20, 100, 100)];
To change the position of the view afterwards, you modify its center property:
videoView.center = CGPointMake(200, 100 );
Solution 2
Here is the sample code, its working fine on iOS 5 and later, this is the new implementation which Youtube API provided, myWeb
is a UIWebView here.
showinfo=0
in URL will remove the top header in the videoView.
NSString *html = [NSString stringWithFormat:@"<html><body><iframe class=\"youtube-player\" type=\"text/html\" width=\"%f\" height=\"%f\" src=\"http://www.youtube.com/embed/q1091sWVCMI?HD=1;rel=0;showinfo=0\" allowfullscreen frameborder=\"0\" rel=nofollow></iframe></body></html>",frame.size.width,frame.size.height];
[myWeb loadHTMLString:html baseURL:nil];
Hope this helps you :)
Solution 3
Here's a version that works in iOS 6 and with the new YouTube embed code:
- (void)embedYouTube:(NSString *)urlString frame:(CGRect)frame {
NSString *html = [NSString stringWithFormat:@"<html><head><style type='text/css'>body {background-color: transparent;color: white;}</style></head><body style='margin:0'><iframe width='%f' height='%f' src='%@' frameborder='0' allowfullscreen></iframe></body></html>", frame.size.width, frame.size.height, urlString];
UIWebView *videoView = [[UIWebView alloc] initWithFrame:frame];
[videoView loadHTMLString:html baseURL:nil];
[self.view addSubview:videoView];
}
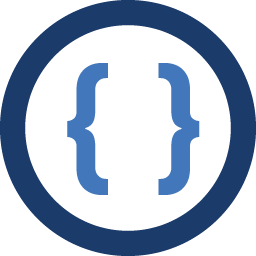
Admin
Updated on February 19, 2020Comments
-
Admin about 4 years
I've embedded a video from YouTube via a snippet I've found on the Internet, here is the code that I've used:
@interface FirstViewController (Private) - (void)embedYouTube:(NSString *)urlString frame:(CGRect)frame; @end @implementation FirstViewController - (void)viewDidLoad { [super viewDidLoad]; [self embedYouTube:@"http://www.youtube.com/watch?v=l3Iwh5hqbyE" frame:CGRectMake(20, 20, 100, 100)]; } - (void)embedYouTube:(NSString *)urlString frame:(CGRect)frame { NSString *embedHTML = @"\ <html><head>\ <style type=\"text/css\">\ body {\ background-color: transparent;\ color: white;\ }\ </style>\ </head><body style=\"margin:0\">\ <embed id=\"yt\" src=\"%@\" type=\"application/x-shockwave-flash\" \ width=\"%0.0f\" height=\"%0.0f\"></embed>\ </body></html>"; NSString *html = [NSString stringWithFormat:embedHTML, urlString, frame.size.width, frame.size.height]; UIWebView *videoView = [[UIWebView alloc] initWithFrame:frame]; [videoView loadHTMLString:html baseURL:nil]; [self.view addSubview:videoView]; [videoView release]; } - (void)didReceiveMemoryWarning { // Releases the view if it doesn't have a superview. [super didReceiveMemoryWarning]; // Release any cached data, images, etc that aren't in use. } - (void)viewDidUnload { // Release any retained subviews of the main view. // e.g. self.myOutlet = nil; } - (void)dealloc { [super dealloc]; } @end
It compiles correctly and when I open it, I see a white box positioned incorrectly, which the code created. I have two questions regarding it:
How do I know if the video will play, it is just a plain white box, does the simulator play videos from YouTube?
How can I position this box?