Encrypting and decrypting xml
Solution 1
I have used DES algorith for encryption and decryption.
For encryption:Here after encryption, I am writing file to save. You can save it with other(temp) name and send it to server. After successful sending you can delete this encrypted file
FileOutputStream fos = null ;
CipherInputStream cis;
byte key[] = "abcdEFGH".getBytes();
SecretKeySpec secretKey = new SecretKeySpec(key,"DES");
Cipher encrypt = Cipher.getInstance("DES/ECB/PKCS5Padding");
encrypt.init(Cipher.ENCRYPT_MODE, secretKey);
InputStream fis = new ByteArrayInputStream(fileData);//Here I am getting file data as byte array. You can convert your file data to InputStream by other way too.
File dataFile = new File(dataDir,fileName); //dataDir is location where my file is stored
if(!dataFile.exists()){
cis = new CipherInputStream(fis,encrypt);
try {
fos = new FileOutputStream(dataFile);
byte[] b = new byte[8];
int i;
while ((i=cis.read(b)) != -1) {
fos.write(b, 0, i);
}
return fileName;
} finally{
try {
if(fos != null)
{
fos.flush();
fos.close();
}
cis.close();
fis.close();
} catch (IOException e) {
//IOException
}
}
}
return "";
For decryption:
CipherInputStream cis;
FileOutputStream fos = null;
FileInputStream fis = null;
File dataFile = new File(dataDir,fileName); // here I am getting encrypted file from server
File newDataFile = new File(dataDir,fileName+"_TEMP"); // I am creating temporary decrypted file
byte key[] = "abcdEFGH".getBytes();
SecretKeySpec secretKey = new SecretKeySpec(key,"DES");
Cipher decrypt = Cipher.getInstance("DES/ECB/PKCS5Padding");
decrypt.init(Cipher.DECRYPT_MODE, secretKey);
try {
fis = new FileInputStream(dataFile);
} catch(Exception e) {
//Exception
}
if(dataFile.exists()){
cis = new CipherInputStream(fis,decrypt);
try {
fos = new FileOutputStream(newDataFile);
byte[] b = new byte[8];
int i;
while ((i=cis.read(b)) != -1) {
fos.write(b, 0, i);
}
return newDataFile;
} finally{
try {
if(fos != null)
{
fos.flush();
fos.close(); }
cis.close();
fis.close();
} catch (IOException e) {
//IOException
}
}
}
Solution 2
What is the problem you're solving?
Maybe SSL is matching you? Encryption out of the box, standart solution.
Also you can take a look at JCA. But i think, it will be too heavy solution for your problem.
Solution 3
In my opinion, you should not try to implement a custom algorithm as first off, you're reinventing the wheel, and second off it will probably be no where near as secure as other more standard encryption routines. If I were you, I would take a look around for some good Java Encryption libraries. One I found is here, http://www.bouncycastle.org/latest_releases.html
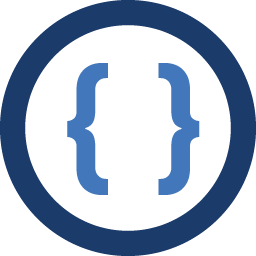
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am making an app in which I have to encrypt the xml from my side and send it to server and in response I will receive xml and I have to decrypt it. I have no idea to encrypt and decrypt. My code is as follows
<?xml version='1.0' encoding='utf-8'?><adm_auth_req><user_name>user.s7</user_name><password>gspcsmo</password></adm_auth_req>
I am using this code to encrypt and decrypt it
public string encryptData(string key, string data) { int keyLen = key.Length; int dataLen = Convert.ToInt16(data.Length); char chData; char chKey; char[] data1 = data.ToCharArray(); char[] key1 = key.ToCharArray(); StringBuilder encryptedData = new StringBuilder(); for (int i = 0; i < dataLen; i++) { chData = data1[i]; for (int j = 0; j < keyLen; j++) { chKey = key1[j]; chData = (char)(chData ^ chKey); } encryptedData.Append(chData); } return (encryptedData.ToString()); }
But still all in vain. Can anyone tell me how to encrypt it and decrypt the result?
-
Admin over 12 yearsi am using your code so now tell me how to encrypt the above xml and decrypt the response from server
-
Admin over 12 yearsSo what is DES and DES/ECB/PKCS5Padding ?
-
Balaji Khadake over 12 yearscheck URL 1. developer.android.com/reference/javax/crypto/spec/…, java.lang.String) and 2. docs.oracle.com/javase/1.4.2/docs/api/javax/crypto/…
-
Admin over 12 yearsbut still i am not clear that what is DES and DES/ECB/PKCS5Padding??
-
Admin over 12 yearsplease tell me about instance used in encryption and decryption : DES/ECB/PKCS5Padding ?
-
Admin over 12 years
-
Maarten Bodewes over 12 yearsThe proposed solution is not cryptographically correct and I would seriously advise you not to follow any other advise of Balaji K (sorry, but that's how it is). DES and ECB are incorrect algorithms for this kind of encryption, and for client/server you will also need integrity and authentication control. I'll try and get back on this, will flag it in the mean time.
-
Admin over 12 years@owlstead, Did you found any answer for my question?
-
Maarten Bodewes over 12 yearsThere are three to four ways of performing the encryption. You cannot just perform encryption without verification in a client/server model because of padding Oracle attacks. The first way is to use simple String encryption and Mac, but basically you would be creating your own proprietary protocol. You could use XML encrypt + XML signature (as included in e.g. WS-security, but those protocols are ridden with pitfalls. Finally, you could use CMS or PGP "containers" to encrypt, although the last one is probably not a good idea. I'll make a simple example for the first method.
-
Maarten Bodewes over 12 yearsBefore I go on, are you sure you cannot simply enable SSL? That would be by far the most logical path to follow. Please reply before I show you how to encrypt XML/Strings, or accept the answer of Artem.