Encryption and Descyption issue in Flutter and C#
1,315
This works for me: Keys:
String keySTR = "16 characters"; //16 byte
String ivSTR = "16 characters"; //16 byte
Flutter
final plainText = 'Works!';
final key = encrypt.Key.fromUtf8(keySTR);
final iv = encrypt.IV.fromUtf8(ivSTR);
final encrypter = encrypt.Encrypter(encrypt.AES(key,mode: encrypt.AESMode.cbc,padding: 'PKCS7'));
final encrypted = encrypter.encrypt(plainText, iv: iv);
final decrypted = encrypter.decrypt(encrypted, iv: iv);
print('decrypted:'+decrypted);
print('encrypted.base64:'+encrypted.base64);
C#
using (System.Security.Cryptography.RijndaelManaged rjm =
new System.Security.Cryptography.RijndaelManaged
{
KeySize = 128,
BlockSize = 128,
Key = ASCIIEncoding.ASCII.GetBytes(keySTR),
IV = ASCIIEncoding.ASCII.GetBytes(ivSTR)
}
)
{
Byte[] input = Encoding.UTF8.GetBytes(stringToEncrypt);
Byte[] output = rjm.CreateEncryptor().TransformFinalBlock(input, 0, input.Length);
return Convert.ToBase64String(output);
}
}
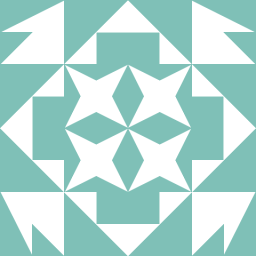
Author by
user2775222
Updated on December 16, 2022Comments
-
user2775222 5 months
I am using encryption in Flutter using the link: https://pub.dev/packages/encrypt
Code is
final plainText = 'some plain text here'; final key = encrypt.Key.fromUtf8('16 characters key'); final iv = IV.fromLength(16); final encrypter = Encrypter(AES(key,mode: AESMode.cbc,padding: 'PKCS7')); final encrypted = encrypter.encrypt(plainText, iv: iv); final decrypted = encrypter.decrypt(encrypted, iv: iv); print(decrypted); print(encrypted.base64);
C# code (for decryption)
public static String TestDecrypt(String encryptedText) { var encryptedBytes = Convert.FromBase64String(encryptedText); return Encoding.UTF8.GetString(Decrypt(encryptedBytes, GetRijndaelManaged("16 characters key"))); } public static RijndaelManaged GetRijndaelManaged(String secretKey) { var keyBytes = new byte[16]; var secretKeyBytes = Encoding.UTF8.GetBytes(secretKey); Array.Copy(secretKeyBytes, keyBytes, Math.Min(keyBytes.Length, secretKeyBytes.Length)); return new RijndaelManaged { Mode = CipherMode.CBC, Padding = PaddingMode.PKCS7, KeySize = 128, BlockSize = 128, Key = keyBytes, IV = keyBytes }; }
I encrypt some plaintext in flutter dart code, But I didn't get proper plain text after decrypting the encrypted text using c# code.
I'm getting a message
Padding is invalid and cannot be removed
-
500 - Internal Server Error over 3 yearsA key is not 16 bytes long just because it says it is :)
-
Maarten Bodewes over 3 years
IV = keyBytes
is suspicious and ` final iv = IV.fromLength(16);` is as well. Whatever you're doing, it is not secure. To find out what is happening, print all the input / output to AES out in hexadecimals - mainly key and IV - and check that they are identical on both sides. The key is the most likely culprit if your plaintext is indeed over 16 characters / bytes in size. -
bartonjs over 3 yearsDon’t set both Key and KeySize. Each updates/resets the other.
-