Entity Framework 6 + SQLite
Solution 1
if you used EF 6.1.3 + System.Data.SQLite v1.0.96.0, just adjust(add) the following declarations in the web.config. (you can find the difference with some text-compare tool, i have numbered them).
<entityFramework>
<defaultConnectionFactory type="System.Data.Entity.Infrastructure.LocalDbConnectionFactory, EntityFramework">
<parameters>
<parameter value="mssqllocaldb" />
</parameters>
</defaultConnectionFactory>
<providers>
<provider invariantName="System.Data.SqlClient" type="System.Data.Entity.SqlServer.SqlProviderServices, EntityFramework.SqlServer" />
<provider invariantName="System.Data.SQLite.EF6" type="System.Data.SQLite.EF6.SQLiteProviderServices, System.Data.SQLite.EF6" />
<!-- 1. Solves SQLite error of "Unable to find the requested .Net Framework Data Provider."-->
<provider invariantName="System.Data.SQLite" type="System.Data.SQLite.EF6.SQLiteProviderServices, System.Data.SQLite.EF6" />
</providers>
</entityFramework>
<system.data>
<DbProviderFactories>
<remove invariant="System.Data.SQLite.EF6" />
<add name="SQLite Data Provider (Entity Framework 6)" invariant="System.Data.SQLite.EF6" description=".NET Framework Data Provider for SQLite (Entity Framework 6)" type="System.Data.SQLite.EF6.SQLiteProviderFactory, System.Data.SQLite.EF6" />
<!-- 2. Solves SQLite error of "Unable to find the requested .Net Framework Data Provider."-->
<remove invariant="System.Data.SQLite"/>
<add name="SQLite Data Provider" invariant="System.Data.SQLite"
description=".Net Framework Data Provider for SQLite" type="System.Data.SQLite.SQLiteFactory, System.Data.SQLite" />
</DbProviderFactories>
</system.data>
It works for me.
Solution 2
The System.Data.SQLite Entity Framework provider will need to be updated to work with version 6 of the Entity Framework. (See Rebuilding EF providers for EF6)
For SQLite, this is a fairly trivial task:
- Download and open the System.Data.SQLite.Linq project
- Remove the reference to System.Data.Entity.dll
- Add a reference to EntityFramework.dll version 6
- Update the broken namespace references
- Rebuild the provider
Jun 21, 2013 Update: I've shared an updated version of the provider on my blog. See System.Data.SQLite on Entity Framework 6 for more information.
Solution 3
I put working solution of EF 6.0 with Sqlite on my Bitbucket account: https://[email protected]/zchpit/sqlitesamples.git
or git https://github.com/zchpit/SQLiteSamples
You can download working solution from that git repository. In my solution, I connect to Sqlite by:
- Data Reader
- Simple.Data
- EF 6.0
p.s. my App.config
<?xml version="1.0"?>
<configuration>
<configSections>
<!-- For more information on Entity Framework configuration, visit http://go.microsoft.com/fwlink/?LinkID=237468 -->
<section name="entityFramework" type="System.Data.Entity.Internal.ConfigFile.EntityFrameworkSection, EntityFramework, Version=6.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" requirePermission="true" />
</configSections>
<startup useLegacyV2RuntimeActivationPolicy="true">
<supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.0,Profile=Client" />
</startup>
<runtime>
<assemblyBinding xmlns="urn:schemas-microsoft-com:asm.v1">
<dependentAssembly>
<assemblyIdentity name="System.Data.SQLite" publicKeyToken="db937bc2d44ff139" culture="neutral" />
<bindingRedirect oldVersion="0.0.0.0-1.0.96.0" newVersion="1.0.96.0" />
</dependentAssembly>
</assemblyBinding>
</runtime>
<system.data>
<DbProviderFactories>
<remove invariant="System.Data.SQLite.EF6" />
<add name="SQLite Data Provider" invariant="System.Data.SQLite" description="Data Provider for SQLite" type="System.Data.SQLite.SQLiteFactory, System.Data.SQLite" />
</DbProviderFactories>
</system.data>
<connectionStrings>
<add name="SqlLiteContext" connectionString="Data Source=|DataDirectory|MyDatabase.sqlite" providerName="System.Data.SQLite" />
</connectionStrings>
<entityFramework>
<providers>
<provider invariantName="System.Data.SQLite" type="System.Data.SQLite.EF6.SQLiteProviderServices, System.Data.SQLite.EF6"/>
</providers>
<defaultConnectionFactory type="System.Data.SQLite.SQLiteFactory, EntityFramework">
<parameters>
<!---parameter value="v11.0" />-->
</parameters>
</defaultConnectionFactory>
</entityFramework>
</configuration>
Solution 4
System.Data.SQLite 1.0.91.0 has been updated to support EF6. Many thanks for Brice's EF SQLite excellent tutorial and update. You need to update your app.config for the new changes if you want it to work with the tutorial. I can confirm that this has worked for me on VS 2010:
<?xml version="1.0"?>
<configuration>
<configSections>
<!-- For more information on Entity Framework configuration, visit http://go.microsoft.com/fwlink/?LinkID=237468 -->
<section name="entityFramework" type="System.Data.Entity.Internal.ConfigFile.EntityFrameworkSection, EntityFramework, Version=6.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" requirePermission="false"/>
</configSections>
<entityFramework>
<defaultConnectionFactory type="System.Data.Entity.Infrastructure.LocalDbConnectionFactory, EntityFramework">
<parameters>
<parameter value="v11.0"/>
</parameters>
</defaultConnectionFactory>
<providers>
<provider invariantName="System.Data.SQLite" type="System.Data.SQLite.EF6.SQLiteProviderServices, System.Data.SQLite.EF6, Version=1.0.91.0, Culture=neutral, PublicKeyToken=db937bc2d44ff139" />
</providers>
</entityFramework>
<system.data>
<DbProviderFactories>
<remove invariant="System.Data.SQLite" />
<add name="SQLite Data Provider" invariant="System.Data.SQLite" description=".Net Framework Data Provider for SQLite" type="System.Data.SQLite.SQLiteFactory, System.Data.SQLite, Version=1.0.91.0, Culture=neutral, PublicKeyToken=db937bc2d44ff139" />
</DbProviderFactories>
</system.data>
<connectionStrings>
<add name="ChinookContext" connectionString="Data Source=|DataDirectory|Chinook_Sqlite_AutoIncrementPKs.sqlite" providerName="System.Data.SQLite"/>
</connectionStrings>
</configuration>
Solution 5
The exception disappeared when reinstalled the NuGet package (System.Data.SQLite version 1.0.94.1) with
Update-Package –reinstall System.Data.SQLite
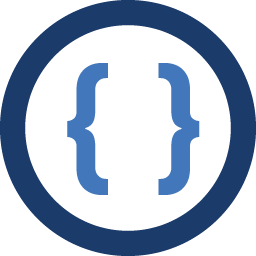
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I'm trying to use EF6 alpha and SQLite 1.0.66.0
My .config file:
<connectionStrings> <add connectionString="data source=:memory:;" name="TestDbContext" providerName="System.Data.SQLite" /> </connectionStrings> <entityFramework> <providers> <provider invariantName="System.Data.SQLite" type="System.Data.SQLite.SQLiteFactory, System.Data.SQLite, Version=1.0.66.0, Culture=neutral, PublicKeyToken=db937bc2d44ff139" /> </providers> </entityFramework> <runtime> <assemblyBinding xmlns="urn:schemas-microsoft-com:asm.v1"> <dependentAssembly> <assemblyIdentity name="EntityFramework" publicKeyToken="b77a5c561934e089" culture="neutral" /> <bindingRedirect oldVersion="0.0.0.0-5.0.0.0" newVersion="5.0.0.0" /> </dependentAssembly> </assemblyBinding> </runtime> <system.data> <DbProviderFactories> <remove invariant="System.Data.SQLite"/> <add name="SQLite Data Provider" invariant="System.Data.SQLite" description=".Net Framework Data Provider for SQLite" type="System.Data.SQLite.SQLiteFactory, System.Data.SQLite, Version=1.0.66.0, Culture=neutral, PublicKeyToken=db937bc2d44ff139" /> </DbProviderFactories> </system.data>
When I run
using (var dbContext = new TestDbContext()) { if (dbContext.Database.Exists()) { dbContext.Database.Delete(); } dbContext.Database.Create(); }
I get an error:
System.InvalidOperationException: System.InvalidOperationException: The 'Instance' member of the Entity Framework provider type 'System.Data.SQLite.SQLiteFactory, System.Data.SQLite, Version=1.0.66.0, Culture=neutral, PublicKeyToken=db937bc2d44ff139' did not return an object that inherits from 'System.Data.Entity.Core.Common.DbProviderServices'. Entity Framework providers must extend from this class and the 'Instance' member must return the Singleton instance of the provider..
What am I doing wrong?