Environment variable with dotenv and TypeScript
Solution 1
Actually you have define the path of the .env file
Try like this
import * as dotenv from "dotenv";
dotenv.config({ path: __dirname+'/.env' });
Try this also
require('dotenv').config({ path: __dirname+'/.env' });
Change the path of .env file as required
reference : https://www.npmjs.com/package/dotenv
Solution 2
If you get undefined value and if you use ES6, you need to import it as follows (.env file must be in project root directory) :
How do I use dotenv with import?
- Preload dotenv: node --require dotenv/config index.js (Note: you do not need to import dotenv with this approach)
- Import dotenv/config instead of dotenv (Note: you do not need to call dotenv.config() and must pass options via the command line or environment variables with this approach)
- Create a separate file that will execute config first as outlined in this comment on #133
You have to import in your project's app.ts file (first) Example with express:
app.ts
//here
import 'dotenv/config'
import express from 'express'
import { userRouter } from './routes/user'
const app = express()
app.use(`/users`, userRouter)
app.listen(process.env.PORT, () => {
console.log(`running`)
})
Now use it anywhere in your project
It is always good to read the documentation
Solution 3
My project has eslint
setup, so I have to disable the import/first
rule
/* eslint-disable import/first */
require('dotenv').config();
import Koa from 'koa';
import { Logger } from './utils/loggers';
import { app } from './app';
const LOGGER = Logger();
const port = parseInt(process.env.PORT as string, 10) || 8081;
const server = (server: Koa) => {
server.listen(port, () => {
LOGGER.info(`> Ready on http://localhost:${port}`);
});
};
server(app());
We can also use:
import 'dotenv/config'
but
require('dotenv').config({path:path_to_dotenv});
is more flexiable.
Solution 4
If this is a React app and you are using react-script you need to prefix the key with REACT_APP_ otherwise they will be ignored.
REACT_APP_TYPE=xxx
REACT_APP_HOST=xxx
REACT_APP_PORT=xxx
REACT_APP_USERNAME=xxx
REACT_APP_PASSWORD=xxx
REACT_APP_DATABASE=xxx
Ref -> https://create-react-app.dev/docs/adding-custom-environment-variables/
Solution 5
You could use built-in NestJs way to handle this (ConfigModule).
// main.ts
import { Module } from '@nestjs/common';
import { ConfigModule } from '@nestjs/config';
import { InventoriesModule } from './inventories/inventories.module';
import typeORMConfig from './config/database.config';
import { TypeOrmModule } from '@nestjs/typeorm';
import { PrismaModule } from './prisma/prisma.module';
@Module({
imports: [
ConfigModule.forRoot(), // load .env file
TypeOrmModule.forRoot(typeORMConfig()),
InventoriesModule,
PrismaModule
],
})
export class AppModule { }
// ./config/database.config
import { TypeOrmModuleOptions } from '@nestjs/typeorm';
export default function (): TypeOrmModuleOptions {
return {
'type': 'mysql',
'host': process.env.DB_HOST,
'port': 3306,
'username': process.env.DB_USERNAME,
'password': process.env.DB_PASSWORD,
'database': process.env.DB_DATABASE,
'entities': ['dist/**/*.entity{.ts,.js}'],
'synchronize': false
}
};
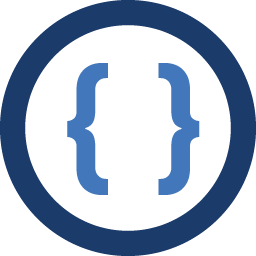
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I create this
.env
file:TYPE=xxx HOST=xxx, PORT=xxx, USERNAME=xxx, PASSWORD=xxx, DATABASE=xxx,
in my file I use in this way:
import * as dotenv from "dotenv"; dotenv.config(); export const typeOrmConfig: TypeOrmModuleOptions = { port: process.env.PORT }
but i can use only my
port
variable from.env
file and i cannot use rest of the variables, can someone tell me why i can't use rest of my vars?