Error 405 (Method Not Allowed) Laravel 5
Solution 1
The methodNotAllowed
exception indicates that a route doesn't exist for the HTTP method you are requesting.
Your form is set up to make a DELETE
request, so your route needs to use Route::delete()
to receive this.
Route::delete('empresas/eliminar/{id}', [
'as' => 'companiesDelete',
'uses' => 'CompaniesController@delete'
]);
Solution 2
Your routes.php file needs to be setup correctly.
What I am assuming your current setup is like:
Route::post('/empresas/eliminar/{id}','CompanyController@companiesDelete');
or something. Define a route for the delete method instead.
Route::delete('/empresas/eliminar/{id}','CompanyController@companiesDelete');
Now if you are using a Route resource, the default route name to be used for the 'DELETE' method is .destroy. Define your delete logic in that function instead.
Solution 3
In my case the route in my router was:
Route::post('/new-order', 'Api\OrderController@initiateOrder')->name('newOrder');
and from the client app I was posting the request to:
https://my-domain/api/new-order/
So, because of the trailing slash I got a 405. Hope it helps someone
Solution 4
If you didn't have such an error during development and it props up only in production try
php artisan route:list
to see if the route exists.
If it doesn't try
php artisan route:clear
to clear your cache.
That worked for me.
Solution 5
This might help someone so I'll put my inputs here as well.
I've encountered the same (or similar) problem. Apparently, the problem was the POST request was blocked by Modsec by the following rules: 350147, 340147, 340148, 350148
After blocking the request, I was redirected to the same endpoint but as a GET request of course and thus the 405.
I whitelisted those rules and voila, the 405 error was gone.
Hope this helps someone.
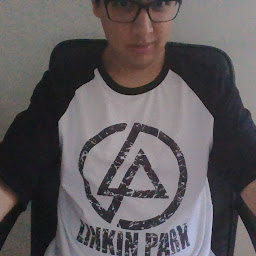
German Ortiz
Updated on July 09, 2022Comments
-
German Ortiz almost 2 years
Im trying to do a POST request with jQuery but im getting a error 405 (Method Not Allowed), Im working with Laravel 5
THis is my code:
jQuery
<script type="text/javascript"> $(document).ready(function () { $('.delete').click(function (e){ e.preventDefault(); var row = $(this).parents('tr'); var id = row.data('id'); var form = $('#formDelete'); var url = form.attr('action').replace(':USER_ID', id); var data = form.serialize(); $.post(url, data, function (result){ alert(result); }); }); }); </script>
HTML
{!! Form::open(['route' => ['companiesDelete', ':USER_ID'], 'method' =>'DELETE', 'id' => 'formDelete']) !!} {!!Form::close() !!}
Controller
public function delete($id, \Request $request){ return $id; }
The Jquery error is http://localhost/laravel5.1/public/empresas/eliminar/5 405 (Method Not Allowed).
The url value is
http://localhost/laravel5.1/public/empresas/eliminar/5
and the data value is
_method=DELETE&_token=pCETpf1jDT1rY615o62W0UK7hs3UnTNm1t0vmIRZ.
If i change to
$.get
request it works fine, but i want to do a post request.Anyone could help me?
Thanks.
EDIT!!
Route
Route::post('empresas/eliminar/{id}', ['as' => 'companiesDelete', 'uses' => 'CompaniesController@delete']);
-
jeremyj11 about 4 yearsThank you!! In my case it was rule 300016 causing the block.
-
Čamo over 3 yearsBut he make an ajax post request. How is it related with form method?
-
Jeemusu over 3 yearsIt is a post request, but op serializes the form data which includes the
method="delete"
attribute and posts it via ajax. -
Čamo over 3 yearsI dont understand. Serialize is only a string.
-
Jeemusu over 3 yearsWhen you use
{!! Form::open( 'method' =>'DELETE' ) !!}
in Laravel, it automatically adds a hidden input called_method
with the designated value, in this case it isDELETE
. Laravel automatically looks for this parameter in every request to determine if it is a DELETE, POST, PATCH, or GET request. I suggest reading the Laravel documentation on method spoofing. laravel.com/docs/5.0/routing#method-spoofing -
Čamo over 3 yearsAh yes I understand. You are right. I cant remember all the documentation.
-
Victor Okech almost 3 yearsIn my case it was the REQUEST-911-METHOD-ENFORCEMENT.conf rule
-
Stas Sorokin about 2 yearsThis solved my issue, thank you! Clearing cache with
php artisan cache:clear
did not help, whilephp artisan route:clear
did the job!