Error: A navigator can only contain 'Screen' components as its direct children
Solution 1
Please check your code whenever writing check the tags and spaces and keep your tags in the same line as much as possible if you are using Visual studio use formatters and autocomplete tags which will help you in solving problems. your code should be as follows:
import React from 'react';
import { Text, View } from 'react-native'
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
function HomeScreen() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }} >
<Text> Home Screen </Text>
</View>
);
}
const Stack = createStackNavigator();
export default function App() {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
Solution 2
I faced similar issue. Turned out that I was using small-case like this : <Stack.screen>
instead of <Stack.Screen>
Solution 3
Just build again. npx react-native run-android
Solution 4
There is one extra space at the end of some of your components. Putting your code in my IDE and using ESLint for formatting it, this is what I got:
import React from 'react'
import { Text, View } from 'react-native'
import { NavigationContainer } from '@react-navigation/native'
import { createStackNavigator } from '@react-navigation/stack'
function HomeScreen() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text> Home Screen </Text>{' '} // <--- right here
</View>
)
}
const Stack = createStackNavigator()
export default function App() {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />{' '} // <--- right here
</Stack.Navigator>{' '} // <--- right here
</NavigationContainer>
)
}
You can see some {' '}
in there. These are extra blank spaces that shouldn't be there. This is what your error is saying, a ' '
was found after the Stack.Screen
. Removing these empty spaces should solve your problem.
By the way, I would highly recommend you to use a JS IDE (if you are not using any) and a linter, like ESLint
. indentation is a very crucial thing to either avoid this kind of bugs and to let your code more legible.
Solution 5
import 'react-native-gesture-handler';
add
import 'react-native-gesture-handler';
at the top (make sure it's at the top and there's nothing else before it) of your entry file, such as App.js or App.jsx or App.ts or App.tsx. I prefer App insted index
if not add in index too
enjoy
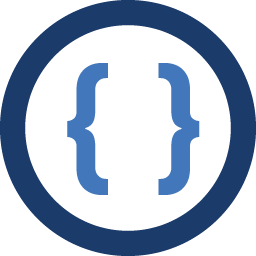
Admin
Updated on July 22, 2022Comments
-
Admin almost 2 years
I am new to React Native and I am getting this error, but I am not able to resolve it.
I am following the tutorial from the main react-navigation page, but I am not able to complete it.
I will appreciate any help. Thanks!
import * as React from 'react'; import { Text, View } from 'react-native' import { NavigationContainer } from '@react-navigation/native'; import { createStackNavigator } from '@react-navigation/stack'; function HomeScreen() { return ( < View style = { { flex: 1, alignItems: 'center', justifyContent: 'center' } } > < Text > Home Screen < /Text> < / View > ); } const Stack = createStackNavigator(); export default function App() { return ( < NavigationContainer > < Stack.Navigator > < Stack.Screen name = "Home" component = { HomeScreen } /> < / Stack.Navigator > < /NavigationContainer > ); }
-
Mote Zart over 2 yearsFor some reason mine only works with this syntax, and your barebones HomeScreen component is great for debugging. I ended up pasting in your code and filling in with my own.
-
sami ullah over 2 yearsnpx react-native run-android -- a typo i think.
-
SeanMC over 2 yearsThis was kind of it for me. I had pasted the Name of a component at the end of my JSX, that I forgot to comment-out or delete after.