error C2504: 'BASECLASS' : base class undefined
Solution 1
The problem is You need to have #include "GXRenderer.h"
at the top of both: GXGL.h and also GXDX.h.
The base type must be defined not just declared before defining a derived type.
By the way, the error is a compiling error not linking error.
Edit: About your class type redefinition:
at the top of every header file you should have #pragma once
.
The #pragma once
directive specifies that the file will be included at most once by the compiler in a build.
Solution 2
You included them all into GXRenderManager.h
, meaning that GXRenderManager.h
is OK.
But you forgot to include them all into GXGL.cpp
and GXDX.cpp
. In these .cpp files GXRenderer
class is completely unknown.
There are at least two "schools" of #include
strategies. One says that header file must include everything that is needed for its own compilation. That would mean that GXGL.h
and GXDX.h
must include GXRenderer.h
. If you followed that strategy, your GXGL.cpp
and GXDX.cpp
would be OK as they are now.
Another "school" says that header files must not include each other at all, i.e. all inclusions must be done through .cpp files. At first sight one could guess that your GXGL.h
and GXDX.h
follow that strategy (since you are not including anything into them), but then your GXRenderManager.h
looks completely different.
You need to decide which strategy you are trying to follow and follow it. I'd recommend the first one.
Solution 3
I got an error C2504: 'CView' : base class undefined where CView is not directly my base class from which I am inheriting.
I am inherting mYClass from MScrollView, "for this matter any class which is not actual Base Class is what the point is to be noted down here" but the error is the C2504. When I have included it in the header where this problem is arising, this problem is resolved.
#include "stdafx.h"
where stdafx.h has #include which contains all the basic class defined...hope this answer resolves everyone who are facing this issue.
Related videos on Youtube
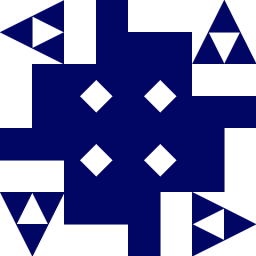
numerical25
Updated on June 04, 2022Comments
-
numerical25 7 months
I checked out a post similar to this but the linkage was different the issue was never resolved. The problem with mine is that for some reason the linker is expecting there to be a definition for the base class, but the base class is just a interface. Below is the error in it's entirety
c:\users\numerical25\desktop\intro todirectx\godfiles\gxrendermanager\gxrendermanager\gxrendermanager\gxdx.h(2) : error C2504: 'GXRenderer' : base class undefined
Below is the code that shows how the headers link with one another
GXRenderManager.h
#ifndef GXRM #define GXRM #include <windows.h> #include "GXRenderer.h" #include "GXDX.h" #include "GXGL.h" enum GXDEVICE { DIRECTX, OPENGL }; class GXRenderManager { public: static int Ignite(GXDEVICE); private: static GXRenderer *renderDevice; }; #endif
at the top of GxRenderManager, there is GXRenderer , windows, GXDX, GXGL headers. I am assuming by including them all in this document. they all link to one another as if they were all in the same document. correct me if I am wrong cause that's how a view headers. Moving on...
GXRenderer.h
class GXRenderer { public: virtual void Render() = 0; virtual void StartUp() = 0; };
GXGL.h
class GXGL: public GXRenderer { public: void Render(); void StartUp(); };
GXDX.h
class GXDX: public GXRenderer { public: void Render(); void StartUp(); };
GXGL.cpp and GXDX.cpp respectively
#include "GXGL.h" void GXGL::Render() { } void GXGL::StartUp() { } //...Next document #include "GXDX.h" void GXDX::Render() { } void GXDX::StartUp() { }
Not sure whats going on. I think its how I am linking the documents, I am not sure.
-
numerical25 over 12 yearsI tried that and I get "error C2011: 'GXRenderer' : 'class' type redefinition" I guess I am seeing this wrong but I thought by including all the headers in 1 document (GXRenderManager.h) that they would all link to each other that way
-
Brian R. Bondy over 12 years@numerical25: Each cpp is compiled into objects separate and then linked together so that's why you can't do like you think with including all somewhere in your project in one file.
-
numerical25 over 12 yearsso for a header to be linked with another header. I must directly put the include in one of them. Books say that the compiler puts all the headers declarations in the exact place where the include was implemented as if they were in the same document. I figure if the computer reads like this, one header would recognize the other and link to each other just by having 2 header includes side by side. But I guess not. Anyhow, that did resolve the problem. Thanks
-
numerical25 over 12 yearsPerhapes I don't understand headers completely. I was stuck in a situation along time ago when headers were not being recognized because they were included already somewhere else. I still never fully understand why I was having that issue. I tried to find sources that addressed this issue but could not. Still I sometimes get confused and think that including a header in multiple documents causes one of them to ignore it because a header can only get called once. Or so I was told.