Error: Cannot match any routes. URL Segment:
Solution 1
Add pathMatch: 'full'
{path: '', component: AppComponent, pathMatch: 'full'},
to routes with empty path ''
and no child routes.
Solution 2
I am integrating Angular 2/4 into an existing MVC application, and I had this problem too.
Using your example, if I had path: 'Clients'
in the routing config instead of path: 'clients'
and tried to go to the URL such as http://localhost/clients
, I would get this error.
The route path names are case sensitive (as of right now anyway). They won't match what's in your URL otherwise, and with typical MVC controller/action naming, they are "InitialCaps-style."
Solution 3
Check the imports session at the app.module.ts and look you did't miss the routing:
imports: [
BrowserModule,
APP_ROUTES,
AppRoutingModule
],
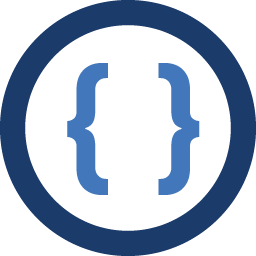
Admin
Updated on October 13, 2020Comments
-
Admin over 3 years
I am trying to follow this tutorial http://onehungrymind.com/named-router-outlets-in-angular-2/ but I am getting the error.
EXCEPTION: Uncaught (in promise): Error: Cannot match any routes. URL Segment: 'clients' Error: Cannot match any routes. URL Segment: 'clients'
Here is my
Router module
import {NgModule} from "@angular/core"; import {Routes, RouterModule} from "@angular/router"; import {AppComponent} from "./app.component"; import {ClientComponent} from "./clients/client.component"; import {ClientDetailsComponent} from "./clients/client-details/client-details.component"; import {ClientListComponent} from "./clients/client-list/client-list.component"; const routes: Routes = [ {path: '', component: AppComponent}, {path: 'clients', component: ClientComponent, children: [ {path: 'list', component: ClientListComponent, outlet: 'client-list'}, {path: ':id', component: ClientDetailsComponent, outlet: 'client-details'} ] }, ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule], providers: [] }) export class RoutingModule { }
App.component
export class AppComponent { clientsLoaded = false; constructor(private clientService: ClientService, private router: Router) { } loadClientsComponent() { this.router.navigate(['/clients', {outlets: {'client-list': ['clients'], 'client-details': ['none'], 'client-accounts-info': ['none']}}]); this.clientsLoaded = true; } }
Client.component
import {Component} from "@angular/core"; import {ClientService} from "../services/client.service"; import {Router} from "@angular/router"; import {Client} from "./client-details/model/client.model"; @Component({ selector: 'clients', templateUrl: 'app/clients/client.component.html' }) export class ClientComponent { clients: Array<Client> = []; constructor(private clientService: ClientService, private router: Router) { } ngOnInit() { this.getClients().subscribe((clients) => { this.clients = clients; }); } getClients() { return this.clientService.getClients(); } }
What can be wrong ? I would be grateful for any help
UPDATE
Thanks for the advice, I have tried something like that
const routes: Routes = [ {path: '', component: AppComponent, pathMatch: 'full'}, {path: 'clients', component: ClientComponent, children: [ {path: '', component: ClientComponent}, {path: 'list', component: ClientListComponent, outlet: 'client-list'}, {path: ':id', component: ClientDetailsComponent, outlet: 'client-details'} ] }, ];
But it still doesn't work.
Maybe I am doing this wrong but I have the following template for my
ClientComponent
<div id="sidebar" class="col-sm-3 client-sidebar"> <h2 class="sidebar__header"> Client list </h2> <div class="sidebar__list"> <router-outlet name="client-list"></router-outlet> </div> </div> <div id="client-info" class="col-sm-4 client-info"> <div class="client-info__section client-info__section--general"> <router-outlet name="client-details"></router-outlet> </div> </div>
So I want my client component to keep all client related components. And if user clicks for example a button then a client list is loaded.