Error: CUICatalog: Invalid asset name supplied: (null), or invalid scale factor : 2.000000
Solution 1
This one appears when someone is trying to put nil
in [UIImage imageNamed:]
Add symbolic breakpoint for [UIImage imageNamed:]
Add $arg3 == nil
condition on Simulator, $r0 == nil
condition on 32-bit iPhone, or $x2 == nil
on 64-bit iPhone.
Run your application and see where debugger will stop.
P.S. Keep in mind this also happens if image name is empty string. You can check this by adding [(NSString*)$x2 length] == 0
to the condition.
Solution 2
This error (usually) happens when you try to load an image with [UIImage imageNamed:myImage]
but iOS is not sure if myImage
is really a NSString
and then you have this warning.
You can fix this using:
[UIImage imageNamed:[NSString stringWithFormat:@"%@", myImage]]
Or you can simply check for the length
of the name of the UIImage
:
if (myImage && [myImage length]) {
[UIImage imageNamed:myImage];
}
Solution 3
Since the error is complaining that the name you gave is (null)
, this is most likely caused by calling [UIImage imageNamed:nil]
. Or more specifically, passing in a variable hasn't been set, so it's equal to nil
. While using stringWithFormat:
would get rid of the error, I think there's a good chance it's not actually doing what you want. If the name you supply is a nil
value, then using stringWithFormat: would result in it looking for an image that is literally named "(null)", as if you were calling [UIImage imageNamed:@"(null)"]
.
Something like this is probably a better option:
if (name) {
UIImage *image = [UIImage imageNamed:name];
} else {
// Do something else
}
You might want to set a breakpoint in Xcode on that "Do something else" line, to help you figure out why this code is getting called with a nil value in the first place.
Solution 4
In Xcode 6.4, this seems to occur when using "Selected Image" for a tab bar item in the storyboard, even if it's a valid image.
This doesn't actually seem to set the selected state image anyway, so it needs to be defined in User Defined Runtime Attributes, and removed from the SelectedImage attribute of the Tab Bar Item
Solution 5
In my case i was passing [UIImage imageNamed:@""] which caused me to show the warning. Just add breakpoints to all the lines where you have used imageNamed and the debug the line where you find the warning.
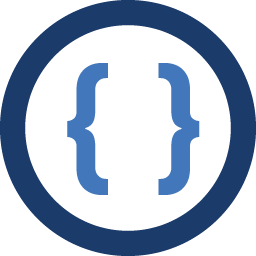
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
TableViewApplication[1458:70b] CUICatalog: Invalid asset name supplied: (null), or invalid scale factor: 2.000000
Getting this warning while working with TableViewController. How to rectify this error and which block is affected?
-
diegomen over 9 yearsI got the same problem and the error was that the image name was nil like robotspacer says.
-
mAu about 9 yearsVery nice approach! I always forget about symbolic breakpoints.
-
cbowns about 9 yearsThis is a clever approach. It looks like my nil image is somewhere in my nib, so it's not going through
UIImage imageNamed:
, but I'm saving this breakpoint nonetheless. -
Zev Eisenberg about 9 yearsI didn't know about the
$arg3
syntax for conditional breakpoints. Where can I learn more? -
Dave G almost 9 yearsIn case anyone made the same mistake I did, I was trying to update a UIButton's background image to be removed (no image at all) and so I changed my UIImage name from a string to just "", which caused the error. By removing the entire UIImage(named: "") and replacing it with nil, the error disappeared.
-
wcochran almost 9 yearsThank you for that! These error/warning messages were driving me nuts! I can't figure out why Apple doesn't either get rid of the Tab Bar Item "Selected Image" pulldown in IB or fix it so it works correctly.
-
derpoliuk over 8 yearsGreat solution, thank you. Is it possible to add condition for result, not for the argument?
-
Ben over 8 yearsNot sure to understand why it doesn't work for me, always returning
error: use of undeclared identifier '$arg3'
. Did I miss something? -
sudoExclaimationExclaimation over 8 yearsi keep getting: Stopped due to an error evaluating condition of breakpoint 23.1: "$r0 == nil" Couldn't parse conditional expression: error: use of undeclared identifier '$r0' error: 1 errors parsing expression
-
hasan over 8 yearsThen what? nothing happen to tell me what asset is that!
-
CHiP-love-NY over 8 yearsI set condition: (int)[(id)($esp+12) length] == 0
-
Jeff almost 8 years@DaveG You sir, saved me hours. Thankyou for the comment!
-
Artem Illarionov over 7 years$arg3 == nil (not $r0 == nil) work for me for iPhone device run
-
Matthew Korporaal over 7 yearsIn my case the image name string was empty, so checking the arg3 length worked for me.
(int)[$arg3 length] == 0
-
Julfikar over 6 yearsI am also using
[UIImage imageNamed:@""]
. How did you fix it? -
Hahnemann over 6 years[aButton setImage:nil forState:UIControlStateNormal]
-
Moshe Gottlieb over 5 yearsThis solved it for me, been looking for this problem for a while now, it drove me nuts. The breakpoint schtick did not work for me.
-
Josh Bernfeld over 5 yearsSame issue here, but the breakpoint did help me find the xib it was in. The image name was Unknown Image in IB.
-
Josh Bernfeld over 5 yearsIf you are having difficulty finding the right register create the breakpoint without the condition, then in lldb run the following
register read -f d
. Look through the printed registers to see which one contains an image name, then use that register in your breakpoint condition. -
gone about 4 yearsOther than using an
if
to test if the image name is not an empty string, or using a default "noImage" image, how do you display no image when no applicable name applies and the Assets do not contain a "noImage" image?