ERROR in AppComponent cannot be used as an entry component
Solution 1
The @Component
decoration should be used on the AppComponent
not the Event
.
Also check that AppComponent
is declared on the AppModule
.
Solution 2
I just wanted to supply an example of the problem explained by @ibenjelloun.
@Component({ ... })
, while looking a bit like a normal function-call, is a actually a decorator, so it must precede the class that you want Angular to treat as a component. So make sure not to put classes between @Component({ ... })
and export class YourComponent
.
So move Event
to before/after AppComponent
:
class Event {
constructor(public title) { }
}
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
...
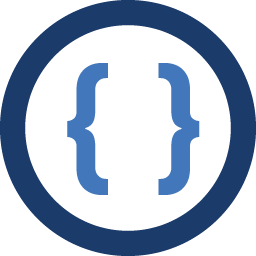
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
Hey I'm new to using Angular and web development, I've been walking through a few tutorials.
When trying to incorporate use of my Firebase Database, upon compilation locally (using ng serve) my application is failing. Returning: "AppComponent cannot be used as an entry component"
If you could offer any help that'd be great. Thanks in advance :)
//app.component.ts import { Component } from '@angular/core'; import {AuthService} from "./services/auth.service"; import {Router} from "@angular/router"; import { AngularFireDatabase } from 'angularfire2/database'; import { AngularFireList } from 'angularfire2/database'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) class Event { constructor(public title) { } } export class AppComponent { title = 'qoqa'; private eventCounter = 0; public events:AngularFireList<Event[]>; constructor(db: AngularFireDatabase, private authService: AuthService, private router: Router) { this.events= db.list('/events'); } public AddEvent(): void { let newEvent = new Event(`My event #${this.eventCounter++}`); this.events.push([newEvent]); } signInWithFacebook() { this.authService.FacebookSignIn() .then((res) => { //this.router.navigate(['dashboard']) }) .catch((err) => console.log(err)); }; signInWithGoogle() { this.authService.GoogleSignIn() .then((res) => { //this.router.navigate(['dashboard']) }) .catch((err) => console.log(err)); } } //app.module.ts import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AngularFireModule } from '@angular/fire'; import { AngularFirestoreModule } from '@angular/fire/firestore'; import { AngularFireAuthModule } from '@angular/fire/auth'; import { environment } from '../environments/environment'; import { LoginComponent } from './login.component'; import { AppRoutingModule } from './app-routing'; import { AngularFireDatabaseModule } from 'angularfire2/database'; import { AuthService } from './services/auth.service'; import { AppComponent } from './app.component'; import { HomeComponent } from './home/home.component'; import { CreateEventComponent } from './create-event/create-event.component'; import { EventsComponent } from './events/events.component'; import { ProfileComponent } from './profile/profile.component'; import { SavedEventsComponent } from './saved-events/saved-events.component'; import { InvitationsComponent } from './invitations/invitations.component'; import { CreateQoqaComponent } from './create-qoqa/create-qoqa.component'; @NgModule({ declarations: [ AppComponent, LoginComponent, HomeComponent, CreateEventComponent, EventsComponent, ProfileComponent, SavedEventsComponent, InvitationsComponent, CreateQoqaComponent ], imports: [ AngularFireModule.initializeApp(environment.firebase), AngularFirestoreModule, AngularFireAuthModule, AngularFireDatabaseModule, AppRoutingModule, BrowserModule ], providers: [AuthService], bootstrap: [AppComponent] }) export class AppModule { }