Error java.lang.ClassNotFoundException: com.google.android.gms.maps.MapFragment in Google Map V2
Solution 1
You problem is the way you added google-play-services
to your project:
I have copied the google-play-services.jar in libs folder and set in the build path of eclipse.
This is wrong! Read the first 3 steps of this blog post I wrote to get an idea of how to do it correctly:
In short, you should import google-play-services
as a project in your workspace.
and then reference it from your project.
this should be the result:
Solution 2
Use support Map fragment in place of Map fragment. Make sure you have added the support libray
<fragment
android:id="@+id/map"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:name="com.google.android.gms.maps.SupportMapFragment "
android:layout_marginBottom="60dp"/>
Second you should not copy the jar file to your build path.
You should refer the google play services library project in your map project.
Import your library project to your worksace. import the same to eclipse.
Right click on your project. goto properties. Choose android. click android. browse and add the library project.
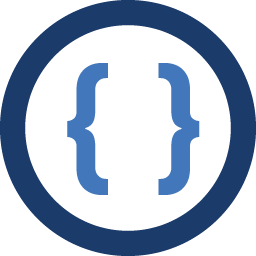
Admin
Updated on January 10, 2020Comments
-
Admin over 4 years
I am using Google Map V2 API for the map. I have copied the google-play-services.jar in libs folder and set in the build path of eclipse.
I am getting exception as I added logcat.
Please help to get solve this issue.
home_map_view.xml
<fragment android:id="@+id/map" android:layout_width="fill_parent" android:layout_height="fill_parent" android:name="com.google.android.gms.maps.MapFragment" android:layout_marginBottom="60dp"/>
AndroidManifest.xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.app" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="17" /> <permission android:name="com.example.app.permission.MAPS_RECEIVE" android:protectionLevel="signature"/> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> <uses-permission android:name="android.permission.CALL_PHONE" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/> <uses-permission android:name="com.example.app.permission.MAPS_RECEIVE"/> <uses-feature android:glEsVersion="0x00020000" android:required="true"/> <application android:icon="@drawable/ic_launcher" android:label="@string/app_name"> <meta-data android:name="com.google.android.maps.v2.API_KEY" android:value="KEY"/> <activity android:name=".MainActivity" android:label="@string/app_name" android:clearTaskOnLaunch="true" android:configChanges="orientation" android:screenOrientation="portrait"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".HomeMapView" android:label="@string/title_home" android:configChanges="orientation" android:launchMode="singleTop" /> </application> </manifest>
HomeMapView.java
public class HomeMapView extends FragmentActivity implements OnTabChangeListener { private GoogleMap mapView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.home_map_view); // Getting reference to SupportMapFragment of the activity_main SupportMapFragment fragment = new SupportMapFragment(); getSupportFragmentManager().beginTransaction() .add(R.id.map, fragment).commit(); // Getting Map for the SupportMapFragment mapView = fragment.getMap(); mapView.setMyLocationEnabled(true); } }
Logcat:
05-15 23:17:52.843: E/AndroidRuntime(19782): FATAL EXCEPTION: main 05-15 23:17:52.843: E/AndroidRuntime(19782): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.app/com.example.app.HomeMapView}: android.view.InflateException: Binary XML file line #13: Error inflating class fragment 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:1651) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:1667) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.app.ActivityThread.access$1500(ActivityThread.java:117) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:935) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.os.Handler.dispatchMessage(Handler.java:99) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.os.Looper.loop(Looper.java:130) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.app.ActivityThread.main(ActivityThread.java:3687) 05-15 23:17:52.843: E/AndroidRuntime(19782): at java.lang.reflect.Method.invokeNative(Native Method) 05-15 23:17:52.843: E/AndroidRuntime(19782): at java.lang.reflect.Method.invoke(Method.java:507) 05-15 23:17:52.843: E/AndroidRuntime(19782): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867) 05-15 23:17:52.843: E/AndroidRuntime(19782): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:625) 05-15 23:17:52.843: E/AndroidRuntime(19782): at dalvik.system.NativeStart.main(Native Method) 05-15 23:17:52.843: E/AndroidRuntime(19782): Caused by: android.view.InflateException: Binary XML file line #13: Error inflating class fragment 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.view.LayoutInflater.createViewFromTag(LayoutInflater.java:587) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.view.LayoutInflater.rInflate(LayoutInflater.java:623) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.view.LayoutInflater.inflate(LayoutInflater.java:408) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.view.LayoutInflater.inflate(LayoutInflater.java:320) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.view.LayoutInflater.inflate(LayoutInflater.java:276) 05-15 23:17:52.843: E/AndroidRuntime(19782): at com.android.internal.policy.impl.PhoneWindow.setContentView(PhoneWindow.java:216) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.app.Activity.setContentView(Activity.java:1660) 05-15 23:17:52.843: E/AndroidRuntime(19782): at com.example.app.HomeMapView.onCreate(HomeMapView.java:61) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1047) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:1615) 05-15 23:17:52.843: E/AndroidRuntime(19782): ... 11 more 05-15 23:17:52.843: E/AndroidRuntime(19782): Caused by: android.support.v4.app.Fragment$InstantiationException: Unable to instantiate fragment com.google.android.gms.maps.MapFragment: make sure class name exists, is public, and has an empty constructor that is public 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.support.v4.app.Fragment.instantiate(Fragment.java:395) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.support.v4.app.Fragment.instantiate(Fragment.java:363) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.support.v4.app.FragmentActivity.onCreateView(FragmentActivity.java:264) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.view.LayoutInflater.createViewFromTag(LayoutInflater.java:563) 05-15 23:17:52.843: E/AndroidRuntime(19782): ... 20 more 05-15 23:17:52.843: E/AndroidRuntime(19782): Caused by: java.lang.ClassNotFoundException: com.google.android.gms.maps.MapFragment in loader dalvik.system.PathClassLoader[/system/framework/com.google.android.maps.jar:/data/app/com.example.app-2.apk] 05-15 23:17:52.843: E/AndroidRuntime(19782): at dalvik.system.PathClassLoader.findClass(PathClassLoader.java:240) 05-15 23:17:52.843: E/AndroidRuntime(19782): at java.lang.ClassLoader.loadClass(ClassLoader.java:551) 05-15 23:17:52.843: E/AndroidRuntime(19782): at java.lang.ClassLoader.loadClass(ClassLoader.java:511) 05-15 23:17:52.843: E/AndroidRuntime(19782): at android.support.v4.app.Fragment.instantiate(Fragment.java:385) 05-15 23:17:52.843: E/AndroidRuntime(19782): ... 23 more
-
Raghunandan almost 11 years@EmilAdz i din't see your post and edit my answer. I am sorry if you feel that way. I already started editing even before you posted the answer. since you could not see my edit you thought otherwise
-
Raghunandan almost 11 years@EmilAdz i posted the answer and then started to edit. could you see while i was editing. in mean time you posted the answer. By the time you could see my update you thought i copied form your answer. I am sorry its not that way. If you feel that way i will upvote your answer. you take the credit
-
Raghunandan almost 11 yearsi can't help if you feel it that way. I din't edit my answer seeing your post.
-
Emil Adz almost 11 yearsWell, never mind. @Raghunandan. I got the feeling that this is the way it was.
-
Admin almost 11 years@Emil Adz I am getting this exception java.lang.NoClassDefFoundError: com.google.android.gms.R$styleable.
-
Emil Adz almost 11 yearshave you committed all the steps described in the Guide?
-
Raghunandan almost 11 years@user1996510 i guess you have not referred to google play services library properly still.
-
Admin almost 11 years+1 Well Done!!!Solved my problem..Getting this issue from last two days.Now solved.It would be helpful for others.
-
Admin almost 11 years@Emil Adz Can you help me in how can i draw route between two location in Google Map V2.
-
Emil Adz almost 11 yearstake a look at this post: stackoverflow.com/questions/16125868/…
-
Admin almost 11 years@Emil Adz Can I set the margin of fragment programmatically at runtime. Please suggest me to do this. I need this.
-
Emil Adz almost 11 yearsI never done that programmatically, open a new question for this. I'm sure you will be helped.
-
mutkan almost 11 yearsToday i updated my google play service lib to rev 7, but there is a problem. It builds google play service normally but there is no jar file in bin folder.
-
Raghunandan over 10 years@EmilAdz could you delete the comment coz someone downvoted the answer probably seeing at your comment. even i don't get a upvote from now on i don't want any more downvotes
-
Abandoned Cart almost 8 years@EmilAdz Your blog is outdated and no longer relevant for current Google Play Services. It now includes individual libraries (in aar format) for each of the desired services, which need to be extracted and converted to individual library projects. The new manifest meta-data is
com.google.android.geo.API_KEY
but uses the same key. This could be why others would think you gave an incorrect answer.