error LNK2019: unresolved external symbol main referenced in function __tmainCRTStartup MSVCRTD.lib(crtexe.obj)
Solution 1
In C++ main function is declared as int main()
or int main(int argc, char *argv[])
(see C++ Standard, paragraph 3.6.1 Main function).
In your case linker can't find the body of main
, so it throws an error.
Solution 2
Project -> Properties -> Configuration Properties -> Linker -> System
and changing SubSystem to Console.
Solution 3
I am not completely sure, but generally these kind of errors are mostly dependent on the kind of the project you choose when creating the project. If you choose Console, you generally need to follow
int _tmain(int argc, _TCHAR* argv[])
but if you have choosen Windows type of project, then you can follow
int WinMain(HINSTANCE hInst,HINSTANCE hPrevInst,LPSTR lpCmdLine,int nShowCmd)
But if you are modifying _tmain to WinMain or vice-versa in an already existing project, you might want to do Arivazhagan has been suggesting.
You can change the settings in Properties -> Configuration Properties -> Linker -> System to Windows if you want WinMain(HINSTANCE hInst,HINSTANCE hPrevInst,LPSTR lpCmdLine,int nShowCmd)
format
and
Console if you want _tmain(int argc, _TCHAR* argv[])
format.
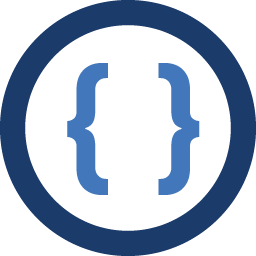
Admin
Updated on August 01, 2022Comments
-
Admin over 1 year
I keep receiving the following two errors and I can't figure out why.
error LNK2019: unresolved external symbol _main referenced in function ___tmainCRTStartup MSVCRTD.lib(crtexe.obj) error LNK1120: 1 unresolved externals
Ensuring that I run it as Console [even in Linker Properties it says Console] I am using VC++ 2012 Ultimate. I'm not insanely technically oriented with jargon and advanced concepts so please try not to overwhelm me Dx.
//Main.cpp #include <conio.h> #include "XArray.h" int Main() { XArray<int> Test; Test.Add(3); getch(); return 1; }
Followed by.
#ifndef XARRAY_H #define XARRAY_H template <class X> class XIndex { public: X Value; XIndex<X> *Next; //Construct XIndex(X ArrayValue) { Value = ArrayValue; Next = nullptr; } }; template <class X> class XArray { XIndex<X> *First; public: //Construct/Destruct; XArray() { First = nullptr; } ~XArray(); //Operators that Alter the structure; void Add(X); //Add X to the end. void AddX(X, int); //Create Int amount of X values void Insert(int, X); //Insert X at Index int bool Remove(int); //Remove Index int. Return true if deleted, false if failed. //void Sort(); //Todo -- Sorts by value. //Operators that deal with the values. X Get(int); void Set(int, X); }; template <class X> void XArray<X>::AddX(X NewVal, int Quantity) { for (int i = 0; i < Quantity; i++) Add(NewVal); } template <class X> void XArray<X>::Add(X NewVal) { XIndex<X> *CurrentIndex; XIndex<X> *NewNode; //Where we store NewVal NewNode = new XIndex<X>(NewVal); //List doesn't exist. if (!First) { First = NewNode; } else { //Start at beginning. CurrentIndex = First; while ((*CurrentIndex).Next){ CurrentIndex = (*CurrentIndex).Next; } (*CurrentIndex).Next = NewNode; } } template <class X> void XArray<X>::Insert(int Index, X NewVal) { XIndex<X> *CurrentIndex; XIndex<X> *PrevIndex; XIndex<X> *NewNode; //Where we store NewVal iCounter = 0; NewNode = new XIndex<X>(NewVal); //List doesn't exist. if (!First) { First = NewNode; } else { //Start at beginning. CurrentIndex = First; while ((*CurrentIndex).Next != nullptr && iCounter < Index){ iCounter += 1 PrevIndex = CurrentIndex; CurrentIndex = (*CurrentIndex).Next; } if (PreviousNode == nullptr) { First = NewNode; (*NewNode).Next = CurrentIndex; } else { (*PrevIndex).Next = NewNode; (*NewNode).Next = CurrentIndex; } } } template <class X> bool XArray<X>::Remove(int Index) { XIndex<X> *CurrentIndex; XIndex<X> *PrevIndex; int iCounter = 0; if (First == nullptr) return false; //We never had a list. //It's the first one; if (Index == 0) { CurrentIndex = (*First).Next; delete First; First = CurrentIndex; return true; } //Starting as normal. CurrentIndex = First; //Loop until we reach the index. while (CurrentIndex != nullptr && iCounter < Index) { iCounter += 1 PrevIndex = CurrentIndex; CurrentIndex = (*CurrentIndex).Next; } if (CurrentIndex != nullptr) { (*PrevIndex).Next = (*CurrentIndex).Next; delete CurrentIndex; return true; } return false; } //Destructor; template <class X> XArray<X>::~XArray() { XIndex<X> *NextIndex; XIndex<X> *CurrentIndex; //Start at beginning. CurrentIndex = First; //So long as we aren't at the end [when Next = nullptr]; while (CurrentIndex != nullptr) { NextIndex = (*CurrentIndex).Next; delete CurrentIndex; CurrentIndex = NextIndex; } } #endif