Error Message: The method sort(List<T>) in the type Collections is not applicable for the arguments (ArrayList<Date>)
31,259
Because Collections.sort
expects java.lang.Comparable
and not your Comparable interface, change your Date
class to implement the java.lang.Comparable
.
public class Date implements java.lang.Comparable<Date>{
..
}
If you still want to define your own Comparable for some reasons and you still want to use Collections.sort then the your Comparable
has to be java.util.Comparable
interface Comparable<T> extends java.lang.Comparable<T> {
}
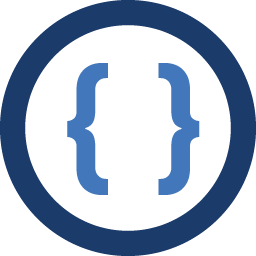
Author by
Admin
Updated on February 29, 2020Comments
-
Admin about 4 years
Keep getting error message, but don't know why. Can't get code to sort the list using Collections.sort()
Here is what I have. 3 java files.
The Interface file.
public interface Comparable<T> { public int compareTo(T other); }
The Class file.
public class Date implements Comparable<Date>{ private int year; private int month; private int day; public Date(int month, int day, int year){ this.month = month; this.day = day; this.year = year; } public int getYear(){ return this.year; } public int getMonth(){ return this.month; } public int getDay(){ return this.day; } public String toString(){ return month + "/" + day + "/" + year; } public int compareTo(Date other){ if (this.year!=other.year){ return this.year-other.year; } else if (this.month != other.month){ return this.month-other.month; } else { return this.day-other.day; } }
}
The Client class
import java.util.*; public class DateTest{ public static void main(String[] args){ ArrayList<Date> dates = new ArrayList<Date>(); dates.add(new Date(4, 13, 1743)); //Jefferson dates.add(new Date(2, 22, 1732)); //Washington dates.add(new Date(3, 16, 1751)); //Madison dates.add(new Date(10, 30, 1735)); //Adams dates.add(new Date(4, 28, 1758)); //Monroe System.out.println(dates); Collections.sort(dates); System.out.println("birthdays = "+dates); } }
The error message I get is "The method sort(List) in the type Collections is not applicable for the arguments (ArrayList)"
-
ever alian over 3 years
java.util.Comparable
?java.lang.comparable
right?