Error: System.NullReferenceException: Object reference not set to an instance of an object
18,625
you are not instantiating your MainViewModel
with the new
keyword
private static MainViewModel mainviewmodel;
public static MainViewModel MainViewModel
{
get
{
mainviewmodel = new MainViewModel();
return mainviewmodel;
}
}
Update
this line should be like this
private ObservableCollection<Member> _data = new ObservableCollection<Member>();
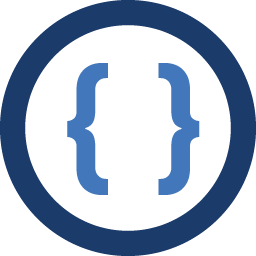
Author by
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
Work with Windows-phone app C#:
I get this error when add my info "System.NullReferenceException: Object reference not set to an instance of an object." tại "Data.Add(newInfo)" I use MVVM pattern.
In MainViewModel.cs, my Add Function is
public class MainViewModel : INotifyPropertyChanged { private MemberDataContext MemberDB; public MainViewModel(string MemberDBConnectionString) { MemberDB = new MemberDataContext(MemberDBConnectionString); } private ObservableCollection<Member> _data; public ObservableCollection<Member> Data { get { return _data; } set { _data = value; NotifyPropertyChanged("Data"); } } public void LoadCollectionsFromDatabase() { var infoInDB = from Member ss in MemberDB.Members select ss; Data = new ObservableCollection<Member>(infoInDB); } public void Addinfo(Member newInfo) { MemberDB.Members.InsertOnSubmit(newInfo); MemberDB.SubmitChanges(); Data.Add(newInfo); **<~~~~~~~ Error in this line** } public void Update(Member currentmember, string fullname, string address) { currentmember.Address = address; currentmember.FullName = fullname; } public void Delete(Member currentmember) { MemberDB.Members.DeleteOnSubmit(currentmember); Data.Remove(currentmember); } public void SaveData() { MemberDB.SubmitChanges(); } public void LoadData() { if (Data == null) { Data = new ObservableCollection<Member>(MemberDB.Members); var oderedFullName = from Member b in MemberDB.Members orderby b.FullName select b; } } public MainViewModel() { MemberDB = new MemberDataContext("Data source=isostore:/Member.sdf"); if (!MemberDB.DatabaseExists()) { MemberDB.CreateDatabase(); } } #region INotifyPropertyChanged Members public event PropertyChangedEventHandler PropertyChanged; // Used to notify the app that a property has changed. private void NotifyPropertyChanged(string propertyName) { if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(propertyName)); } } #endregion } public class ViewDetails : INotifyPropertyChanged { private string _fullname; public string FullName { get { return _fullname; } set { _fullname = value; if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs("Fullname")); } } } private string _address; public string Address { get { return _address; } set { _address = value; if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs("Address")); } } } public void LoadDetails(Member abc) { FullName = abc.FullName; Address = abc.Address; } public void UpdateDetails(Member abc) { abc.FullName = FullName; abc.Address = Address; } #region INotifyPropertyChanged Members public event PropertyChangedEventHandler PropertyChanged; // Used to notify the app that a property has changed. private void NotifyPropertyChanged(string propertyName) { if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(propertyName)); } } #endregion
My Add.xaml:
<StackPanel x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0"> <TextBlock Text="FullName"/> <TextBox x:Name="addfullname"/> <TextBlock Text="Address"/> <TextBox x:Name="addaddress" /> </StackPanel>
My Add Button:
private void Add_Click(object sender, EventArgs e) { if ((addaddress.Text.Length > 0) && (addfullname.Text.Length > 0)) { Member newInfo = new Member { FullName = addfullname.Text, Address = addaddress.Text }; App.MainViewModel.Add(newInfo); if (NavigationService.CanGoBack) { NavigationService.GoBack(); } } //else MessageBox.Show("Data cannot be null!"); }
In my App.xaml
public partial class App : Application { private static object _lockObject = new object(); private static MainViewModel mainviewmodel; public static MainViewModel MainViewModel { get { if (mainviewmodel == null) { lock (_lockObject) { mainviewmodel = new MainViewModel(); } } return mainviewmodel; } }