Error: The argument type 'String Function(String)' can't be assigned to the parameter type 'String? Function(String?)?'
Solution 1
The body might complete normally, causing 'null' to be returned, but the return type is a potentially non-nullable type.
When you don't return anything inside a function/method, it implicitely returns null, so this
String validateFirstName(String value) {
if(value.length<2){
return 'İsim en az iki karakter olmalıdır';
}
}
is equivalent to this
String validateFirstName(String value) {
if(value.length<2){
return 'İsim en az iki karakter olmalıdır';
}
return null;
}
But the return type of this method is String
, a non-nullable type. To make it nullable (since you're returning null), add a question mark after it:
// v
String? validateFirstName(String value) {
if(value.length<2){
return 'İsim en az iki karakter olmalıdır';
}
}
The argument type 'String Function(String)' can't be assigned to the parameter type 'String? Function(String?)?'.
The validator
parameter of TextFormField
is a function that receives a nullable type and returns a nullable type (i.e. a String? Function(String?), so just add a question mark after the value
parameter:
// v
String? validateFirstName(String? value) {
if (value == null) {
// The user haven't typed anything
return "Type something, man!";
}
if (value.length<2){
return 'İsim en az iki karakter olmalıdır';
}
}
Solution 2
TextFormField validator accept a function which return String? that take a parameter String? so refactor your code like below.
class StudentValidationMixin {
String? validateFirstName(String? value) {
if(value == null)
return "İsim boş olamaz!";
if(value.length<2){
return 'İsim en az iki karakter olmalıdır';
}
return null;
}
}
Solution 3
TextFormField(
validator: (value) {
controller.isNumberValid(value);
So for something like the validator for the TextFormField, you need to approach it this way to avoid that error.
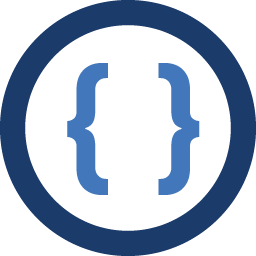
Admin
Updated on December 30, 2022Comments
-
Admin 2 minutes
class StudentValidationMixin { String validateFirstName(String value) { if(value.length<2){ return 'İsim en az iki karakter olmalıdır'; } } }
The body might complete normally, causing 'null' to be returned, but the return type is a potentially non-nullable type.
class _StudentAddState extends State with StudentValidationMixin { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Yeni öğrenci ekle"), ), body: Container( margin: EdgeInsets.all(20.0), child: Form( child: Column( children: <Widget>[ TextFormField( decoration: InputDecoration(labelText: "Öğrenci Adı", hintText: "Fatih"), validator: validateFirstName, ), ], ), ), ), ); } }
The argument type 'String Function(String)' can't be assigned to the parameter type 'String? Function(String?)?'.
These two separate bits of code are getting an error. What should I do?