error TS1259: Module '"./node_modules/@types/express/index"' can only be default-imported using the 'esModuleInterop' flag
Solution 1
According to your GitHub repo, you have the following structure (some files/directories have been omitted):
Bad:
- server
- src
- tsconfig.json
- src
- server2
- src
- tsconfig.json
You will need to move /server/src/tsconfig.json
to /server/tsconfig.json
- please note that you will need to MOVE, not COPY the tsconfig file.
Good:
- server
- src
- tsconfig.json
- server2
- src
- tsconfig.json
Solution 2
In my case, I was facing this error for react. I fixed it by changing my import style from
import React from 'react';
to
import * as React from 'react';
Solution 3
As the error states, you need the set
"esModuleInterop": true,
in your tsconfig.json
file. This allows default imports from modules with no default export.
Solution 4
tsconfig.json
{
"compilerOptions": {
"esModuleInterop": true,
}
}
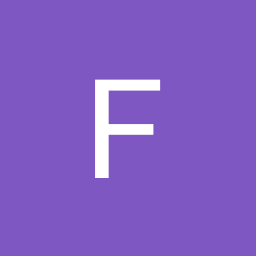
Francisco Pena
Updated on July 05, 2022Comments
-
Francisco Pena almost 2 years
I'm trying to import express into the project and in the middle of the development, it just stopped working, i've reseted configurations, etc. Can't make it work, can't import any modules.
npx tsc src/server.ts src/server.ts:1:8 - error TS1259: Module '"/home/fpc-ubut/Git/nlw2/server/node_modules/@types/express/index"' can only be default-imported using the 'esModuleInterop' flag 1 import express from 'express';
src/database/migrations/00_create_users.ts:1:8 - error TS1259: Module '"/home/fpc-ubut/Git/nlw2/server/node_modules/knex/types/index"' can only be default-imported using the 'esModuleInterop' flag 1 import Knex from 'knex'
src/server.ts:1:8 - error TS1259: Module '"path"' can only be default-imported using the 'esModuleInterop' flag 1 import path from 'path'
Enviroment OS: Ubuntu 20.04.1 LTS
node: v12.18.1
packages:"@types/express": "^4.17.6", "@types/node": "^14.0.27", "ts-node": "^8.10.2", "ts-node-dev": "^1.0.0-pre.44", "typescript": "^3.9.3" "express": "^4.17.1", "knex": "^0.21.1", "pg": "^8.2.1"
EDIT1: as asked, here is my tsconfig.json. But the configuration ("esModuleInterop": true), is already as aspected
<tsconfig.json>
{ "compilerOptions": { /* Visit https://aka.ms/tsconfig.json to read more about this file */ /* Basic Options */ // "incremental": true, /* Enable incremental compilation */ "target": "es5", /* Specify ECMAScript target version: 'ES3' (default), 'ES5', 'ES2015', 'ES2016', 'ES2017', 'ES2018', 'ES2019', 'ES2020', or 'ESNEXT'. */ "module": "commonjs", /* Specify module code generation: 'none', 'commonjs', 'amd', 'system', 'umd', 'es2015', 'es2020', or 'ESNext'. */ // "lib": [], /* Specify library files to be included in the compilation. */ // "allowJs": true, /* Allow javascript files to be compiled. */ // "checkJs": true, /* Report errors in .js files. */ // "jsx": "preserve", /* Specify JSX code generation: 'preserve', 'react-native', or 'react'. */ // "declaration": true, /* Generates corresponding '.d.ts' file. */ // "declarationMap": true, /* Generates a sourcemap for each corresponding '.d.ts' file. */ // "sourceMap": true, /* Generates corresponding '.map' file. */ // "outFile": "./", /* Concatenate and emit output to single file. */ // "outDir": "./", /* Redirect output structure to the directory. */ // "rootDir": "./", /* Specify the root directory of input files. Use to control the output directory structure with --outDir. */ // "composite": true, /* Enable project compilation */ // "tsBuildInfoFile": "./", /* Specify file to store incremental compilation information */ // "removeComments": true, /* Do not emit comments to output. */ // "noEmit": true, /* Do not emit outputs. */ // "importHelpers": true, /* Import emit helpers from 'tslib'. */ // "downlevelIteration": true, /* Provide full support for iterables in 'for-of', spread, and destructuring when targeting 'ES5' or 'ES3'. */ // "isolatedModules": true, /* Transpile each file as a separate module (similar to 'ts.transpileModule'). */ /* Strict Type-Checking Options */ "strict": true, /* Enable all strict type-checking options. */ // "noImplicitAny": true, /* Raise error on expressions and declarations with an implied 'any' type. */ // "strictNullChecks": true, /* Enable strict null checks. */ // "strictFunctionTypes": true, /* Enable strict checking of function types. */ // "strictBindCallApply": true, /* Enable strict 'bind', 'call', and 'apply' methods on functions. */ // "strictPropertyInitialization": true, /* Enable strict checking of property initialization in classes. */ // "noImplicitThis": true, /* Raise error on 'this' expressions with an implied 'any' type. */ // "alwaysStrict": true, /* Parse in strict mode and emit "use strict" for each source file. */ /* Additional Checks */ // "noUnusedLocals": true, /* Report errors on unused locals. */ // "noUnusedParameters": true, /* Report errors on unused parameters. */ // "noImplicitReturns": true, /* Report error when not all code paths in function return a value. */ // "noFallthroughCasesInSwitch": true, /* Report errors for fallthrough cases in switch statement. */ /* Module Resolution Options */ // "moduleResolution": "node", /* Specify module resolution strategy: 'node' (Node.js) or 'classic' (TypeScript pre-1.6). */ // "baseUrl": "./", /* Base directory to resolve non-absolute module names. */ // "paths": {}, /* A series of entries which re-map imports to lookup locations relative to the 'baseUrl'. */ // "rootDirs": [], /* List of root folders whose combined content represents the structure of the project at runtime. */ // "typeRoots": [], /* List of folders to include type definitions from. */ // "types": [], /* Type declaration files to be included in compilation. */ // "allowSyntheticDefaultImports": true, /* Allow default imports from modules with no default export. This does not affect code emit, just typechecking. */ "esModuleInterop": true, /* Enables emit interoperability between CommonJS and ES Modules via creation of namespace objects for all imports. Implies 'allowSyntheticDefaultImports'. */ // "preserveSymlinks": true, /* Do not resolve the real path of symlinks. */ // "allowUmdGlobalAccess": true, /* Allow accessing UMD globals from modules. */ /* Source Map Options */ // "sourceRoot": "", /* Specify the location where debugger should locate TypeScript files instead of source locations. */ // "mapRoot": "", /* Specify the location where debugger should locate map files instead of generated locations. */ // "inlineSourceMap": true, /* Emit a single file with source maps instead of having a separate file. */ // "inlineSources": true, /* Emit the source alongside the sourcemaps within a single file; requires '--inlineSourceMap' or '--sourceMap' to be set. */ /* Experimental Options */ // "experimentalDecorators": true, /* Enables experimental support for ES7 decorators. */ // "emitDecoratorMetadata": true, /* Enables experimental support for emitting type metadata for decorators. */ /* Advanced Options */ "skipLibCheck": true, /* Skip type checking of declaration files. */ "forceConsistentCasingInFileNames": true /* Disallow inconsistently-cased references to the same file. */ } }
EDIT 2: Changed tsconfig.json
"allowSyntheticDefaultImports": true
Still showing the same errorEDIT 3: Github link: [REMOVED]
the problem is on
./server
./server2
is working just fine. Same configs.EDIT4: RESOLVED
According to your GitHub repo, ./server does NOT have a tsconfig file while .server2 does have one.. If I need to be more clear: they do not have the same config, because server does not even have a config. You should move ./server/src/tsconfig.json to ./server/tsconfig.json, just like your tsconfig file is in ./server2 (notice I said MOVE not COPY the tsconfig).. – Matt Oestreich
-
Matt Oestreich almost 4 yearsShowing your
tsconfig.json
file is going to be more important than yourpackage.json
... Perhaps, this may help? -
Matt Oestreich almost 4 yearsSo what changed then? Was your tsconfig.json file recently modified? Did you change the import statements? Have you tried basic troubleshooting? PLEASE help people help you.
-
Matt Oestreich almost 4 yearsAccording to your GitHub repo,
./server
does NOT have a tsconfig file while.server2
does have one.. If I need to be more clear: they do not have the same config, becauseserver
does not even have a config. You should move./server/src/tsconfig.json
to./server/tsconfig.json
, just like your tsconfig file is in./server2
(notice I said MOVE not COPY the tsconfig).. -
Francisco Pena almost 4 yearsI just added on the project some database modules, and everything crashed. I use the same one on every project. And since i'm not explicitly using it yest, It should not be breaking the project. Like i said, i'm using the same versions as another project. I did not change import statements, I'm trying to import the node native modules. And removed unused packages
-
Matt Oestreich almost 4 yearsFranciscoPena, please try this: You should move
./server/src/tsconfig.json
to./server/tsconfig.json
, just like yourtsconfig
file is in./server2
(notice I said MOVE not COPY the tsconfig).. -
Francisco Pena almost 4 years
./src/tsconfig.json
bad tsconfig.json position just like u said. I did check that once, :/ Moved it to root directory, worked just fine. Thanks for the help, gonna take a break in the coding haha -.-'. Again, thanks. -
Matt Oestreich almost 4 yearsFranciscoPena haha no worries - we all make mistakes and overlook the little things sometimes. I went ahead and added an official answer, so please make sure to mark it as the accepted answer if my solution resolved your issue.
-
-
Francisco Pena almost 4 yearsMy tsconfig.json already shows the config <tsconfig.json> "esModuleInterop": true
-
frozen almost 4 yearshow about setting
allowSyntheticDefaultImports
to true? -
Francisco Pena almost 4 yearsChanged it: "allowSyntheticDefaultImports": true, still showing the error
-
Francisco Pena almost 4 yearsYeah, it's on the comment, should i file an issue for typescript? I just copied the tsconfig file from another project that it's working as expected, same modules, rollbacked the modules for the same versions, etc. I'll post the github link, it's from "bootcamp"
-
frazras over 3 yearsput this under "compilerOptions": {"esModuleInterop": true,)
-
mikemaccana over 2 yearsSame here (not using React). The reason this is the best solution is that JSON files don't have a default export, so you need to specify a name during import.
-
yun over 2 yearsalso using
tsc -p .
package.json { scripts: { "compile": "tsc -p ."} } -
Lioness about 2 years@FranciscoPena I had to use the command line flag --esModuleInterop to get it to work
-
Tiramonium about 2 yearsMine threw a hissy fit and demanded the exact opposite. Was importing Express with the * anonymous identifier and Typescript demanded it to be named instead. At least it all worked out in the end.