error: Undefined name 'context' in flutter
6,814
It is because you are trying to access context outside of its scope. Move list1 and list2 to the method build
like this:-
import 'package:flutter/material.dart';
import 'package:origitive_app/main.dart';
import 'package:origitive_app/Productpage.dart';
void main() {
runApp(chooseCategory());
}
class chooseCategory extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new MaterialApp(
debugShowCheckedModeBanner: false,
home: new DefaultTabController(
length: 3,
child: new Scaffold(
appBar: new AppBar(
title: const Text('Collections'),
backgroundColor: Colors.grey[400],
actions: [
//list if widget in appbar actions
PopupMenuButton(
icon: Icon(Icons.menu),
//don't specify icon if you want 3 dot menu
color: Colors.blue,
itemBuilder: (context) =>
[
PopupMenuItem<int>(
value: 0,
child: Text(
"Home", style: TextStyle(color: Colors.white),),
),
PopupMenuItem<int>(
value: 0,
child: Text(
"About", style: TextStyle(color: Colors.white),),
),
PopupMenuItem<int>(
value: 0,
child: Text(
"Settings", style: TextStyle(color: Colors.white),),
),
],
onSelected: (item) => {print(item)},
),
],
bottom: new TabBar(isScrollable: true, tabs: [
new Tab(text: 'MEN',),
new Tab(text: 'WOMEN',),
new Tab(text: 'KIDS',),
]),
),
body: new TabBarView(
children: [
new ListView(
children: list1,
),
new ListView(
children: list2,
),
new ListView(
children: list3,
),
],
),
bottomNavigationBar: BottomNavigationBar(
type: BottomNavigationBarType.fixed,
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(Icons.home),
title: Text('Home'),
),
BottomNavigationBarItem(
icon: Icon(Icons.business),
title: Text('Business'),
),
BottomNavigationBarItem(
icon: Icon(Icons.computer),
title: Text('Technology'),
),
BottomNavigationBarItem(
icon: Icon(Icons.book),
title: Text('Education'),
),
],
),
),
));
List<Widget> list1 = <Widget>[
new ListTile(
title: new Text('Shoes',
style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)),
leading: new Image.asset("assets/Images/men1.png"),
trailing: Icon(Icons.arrow_forward),
onTap: (){
Navigator.push(
context,//error
MaterialPageRoute(builder: (context) => Productpage()));
},
),
new ListTile(
title: new Text('Clothing',
style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)),
subtitle: new Text('429 Castro St'),
leading: new Image.asset("assets/Images/men2.png"),
trailing: Icon(Icons.arrow_forward),
onTap: (){
Navigator.push(
context,//error
MaterialPageRoute(builder: (context) => Productpage()));
},
),
new ListTile(
title: new Text('Accessories',
style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)),
leading: new Image.asset("assets/Images/men3.png"),
trailing: Icon(Icons.arrow_forward),
onTap: (){
Navigator.push(
context,//error
MaterialPageRoute(builder: (context) => Productpage()));
},
),
];
List<Widget> list2 = <Widget>[
new ListTile(
title: new Text('Shoes',
style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)),
subtitle: new Text('85 W Portal Ave'),
leading: new Image.asset("assets/Images/women1.png"),
trailing: Icon(Icons.arrow_forward),
onTap: (){
Navigator.push(
context,//error
MaterialPageRoute(builder: (context) => Productpage()));
},
),
new ListTile(
title: new Text('Clothing',
style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)),
leading: new Image.asset("assets/Images/women2.png"),
trailing: Icon(Icons.arrow_forward),
onTap: (){
Navigator.push(
context,//error
MaterialPageRoute(builder: (context) => Productpage()));
},
),
new ListTile(
title: new Text('Accessories',
style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)),
leading: new Image.asset("assets/Images/women3.png"),
trailing: Icon(Icons.arrow_forward),
onTap: (){
Navigator.push(
context,//error
MaterialPageRoute(builder: (context) => Productpage()));
},
),
];
List<Widget> list3 = <Widget>[
new ListTile(
title: new Text('Boys Shoes',
style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)),
leading: new Image.asset("assets/Images/boy1.png"),
trailing: Icon(Icons.arrow_forward),
onTap: (){
Navigator.push(
context,//error
MaterialPageRoute(builder: (context) => Productpage());
);
},
),
new ListTile(
title: new Text('Girls Shoes',
style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)),
leading: new Image.asset("assets/Images/girl1.png"),
trailing: Icon(Icons.arrow_forward),
onTap: (){
Navigator.push(
context,//error
MaterialPageRoute(builder: (context) => Productpage()));
},
),
new ListTile(
title: new Text('Boys Clothing',
style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)),
leading: new Image.asset("assets/Images/boy1.png"),
trailing: Icon(Icons.arrow_forward),
onTap: () {
Navigator.push(
context,//error
MaterialPageRoute(builder: (context) => Productpage()));
);
},
),
new ListTile(
title: new Text('Girls Clothing',
style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)),
leading: new Image.asset("assets/Images/girl2.png"),
onTap: (){
Navigator.push(
context,//error
MaterialPageRoute(builder: (context) => Productpage()));
},trailing: Icon(Icons.arrow_forward),
),
];
}
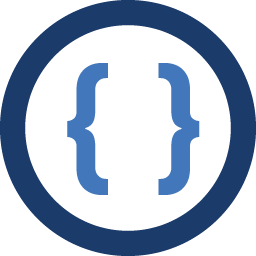
Author by
Admin
Updated on December 31, 2022Comments
-
Admin over 1 year
I am a beginner with flutter, I had just started a few days ago. I want to go from one page to another page . But when I use the navigator it shows an error.
I've tried to solve it using some answers to similar problems on stack overflow, but I can't solve it. Also, I am not able to understand those properly.
These are some of them:
Undefined name 'context'
Undefined name 'context' in flutter navigation
import 'package:flutter/material.dart'; import 'package:origitive_app/main.dart'; import 'package:origitive_app/Productpage.dart'; void main() { runApp(chooseCategory()); } class chooseCategory extends StatelessWidget { @override Widget build(BuildContext context) { return new MaterialApp( debugShowCheckedModeBanner: false, home: new DefaultTabController( length: 3, child: new Scaffold( appBar: new AppBar( title: const Text('Collections'), backgroundColor: Colors.grey[400], actions: [ //list if widget in appbar actions PopupMenuButton( icon: Icon(Icons.menu), //don't specify icon if you want 3 dot menu color: Colors.blue, itemBuilder: (context) => [ PopupMenuItem<int>( value: 0, child: Text( "Home", style: TextStyle(color: Colors.white),), ), PopupMenuItem<int>( value: 0, child: Text( "About", style: TextStyle(color: Colors.white),), ), PopupMenuItem<int>( value: 0, child: Text( "Settings", style: TextStyle(color: Colors.white),), ), ], onSelected: (item) => {print(item)}, ), ], bottom: new TabBar(isScrollable: true, tabs: [ new Tab(text: 'MEN',), new Tab(text: 'WOMEN',), new Tab(text: 'KIDS',), ]), ), body: new TabBarView( children: [ new ListView( children: list1, ), new ListView( children: list2, ), new ListView( children: list3, ), ], ), bottomNavigationBar: BottomNavigationBar( type: BottomNavigationBarType.fixed, items: const <BottomNavigationBarItem>[ BottomNavigationBarItem( icon: Icon(Icons.home), title: Text('Home'), ), BottomNavigationBarItem( icon: Icon(Icons.business), title: Text('Business'), ), BottomNavigationBarItem( icon: Icon(Icons.computer), title: Text('Technology'), ), BottomNavigationBarItem( icon: Icon(Icons.book), title: Text('Education'), ), ], ), ), )); } List<Widget> list1 = <Widget>[ new ListTile( title: new Text('Shoes', style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)), leading: new Image.asset("assets/Images/men1.png"), trailing: Icon(Icons.arrow_forward), onTap: (){ Navigator.push( context,//error MaterialPageRoute(builder: (context) => Productpage())); }, ), new ListTile( title: new Text('Clothing', style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)), subtitle: new Text('429 Castro St'), leading: new Image.asset("assets/Images/men2.png"), trailing: Icon(Icons.arrow_forward), onTap: (){ Navigator.push( context,//error MaterialPageRoute(builder: (context) => Productpage())); }, ), new ListTile( title: new Text('Accessories', style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)), leading: new Image.asset("assets/Images/men3.png"), trailing: Icon(Icons.arrow_forward), onTap: (){ Navigator.push( context,//error MaterialPageRoute(builder: (context) => Productpage())); }, ), ]; List<Widget> list2 = <Widget>[ new ListTile( title: new Text('Shoes', style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)), subtitle: new Text('85 W Portal Ave'), leading: new Image.asset("assets/Images/women1.png"), trailing: Icon(Icons.arrow_forward), onTap: (){ Navigator.push( context,//error MaterialPageRoute(builder: (context) => Productpage())); }, ), new ListTile( title: new Text('Clothing', style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)), leading: new Image.asset("assets/Images/women2.png"), trailing: Icon(Icons.arrow_forward), onTap: (){ Navigator.push( context,//error MaterialPageRoute(builder: (context) => Productpage())); }, ), new ListTile( title: new Text('Accessories', style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)), leading: new Image.asset("assets/Images/women3.png"), trailing: Icon(Icons.arrow_forward), onTap: (){ Navigator.push( context,//error MaterialPageRoute(builder: (context) => Productpage())); }, ), ]; List<Widget> list3 = <Widget>[ new ListTile( title: new Text('Boys Shoes', style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)), leading: new Image.asset("assets/Images/boy1.png"), trailing: Icon(Icons.arrow_forward), onTap: (){ Navigator.push( context,//error MaterialPageRoute(builder: (context) => Productpage()); ); }, ), new ListTile( title: new Text('Girls Shoes', style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)), leading: new Image.asset("assets/Images/girl1.png"), trailing: Icon(Icons.arrow_forward), onTap: (){ Navigator.push( context,//error MaterialPageRoute(builder: (context) => Productpage())); }, ), new ListTile( title: new Text('Boys Clothing', style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)), leading: new Image.asset("assets/Images/boy1.png"), trailing: Icon(Icons.arrow_forward), onTap: () { Navigator.push( context,//error MaterialPageRoute(builder: (context) => Productpage())); ); }, ), new ListTile( title: new Text('Girls Clothing', style: new TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0)), leading: new Image.asset("assets/Images/girl2.png"), onTap: (){ Navigator.push( context,//error MaterialPageRoute(builder: (context) => Productpage())); },trailing: Icon(Icons.arrow_forward), ), ]; } }
tried adding a static build context but failed.it gave a null error.
-
Admin almost 3 yearsI tried doing that but it comments out the whole three lists, but i cant find why. no commenters have been applied to them .
-
Zia over 2 yearsNo need to use statefull widget, instead of creating a simple list, create a function to return your list, and then wherever you call the function inside build pass a context.