Error when creating an enum in C# - "identifier expected"
Solution 1
Sure can add attributes:
enum e
{
[Description("10:30 a.m.")]
AM1030,
[Description("10:31 a.m.")]
AM1031,
[Description("10:32 a.m.")]
AM1032,
}
var type = e.GetType();
var memInfo = type.GetMember(e.AM1030.ToString());
var attributes = memInfo[0].GetCustomAttributes(typeof(DescriptionAttribute), false);
var description = ((DescriptionAttribute)attributes[0]).Description;
Solution 2
You could do
enum e
{
time1,
time2,
time3,
}
You cannot have values like 10.30 a.m. as enum types.
Solution 3
I would suggest looking at DateTime: http://msdn.microsoft.com/en-us/library/system.datetime.aspx
Creating an enumeration for possible times of the day is going to be prone to code rot, since you're going to have to change that enum every time you want to support a different minute of the day.
But, if your business logic is really that specific to those three minutes, what you want is something like:
enum Minute
{
TenThirty,
TenThirtyOne,
TenThirtyTwo
}
The enum just takes identifiers, and they have to be a single word with no whitespace.
Solution 4
One option will be not to use enums in your case but something like this:
public static class Times {
public static DateTime AM1030 = new DateTime(1,1,0,10,30);
public static DateTime AM1031 = new DateTime(1,1,0,10,31);
public static DateTime AM1032 = new DateTime(1,1,0,10,32);
}
var time = Times.AM1030;
The truth is it doesn't give you any value, because you are hardcoding the values anyway. The best will be giving it a business concept, naming those dates as something (I mean, with a word).
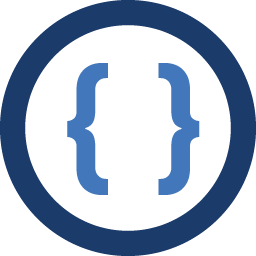
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I have never used enums before i have a problem while creating enums
i want to create an enum in C# which is some thing like
enum e{10:30 a.m.,10:31 a.m.,10:32 a.m.}
but it says identifier expected.. can u help me create it.
-
TomTom over 12 yearsActually you can not ahve values like "10:30am" as IDENTIFIERS - that is methodn ame, variable name, class name, nothing. As a comment on the question said: time for reading "C# for absolute beginners" or some similar book.
-
John Sobolewski over 12 yearsyou can use any integral type as the value of the enum identifiers but they have to all be the same type... as the enum itself is actually of that type. you could have 10:30 a.m. as the value of the enum but you would have to do something crazy like convert 10:30 AM to an int and use that as the value... smells like a code smell
-
MyKuLLSKI over 12 yearsUm no...enums can be in a class.
-
Ralph Willgoss over 12 years+1 for the use of the Description attribute, to go one step further add an extension method to enums so its easier to get the description e.g. enum.GetDescription()
-
MyKuLLSKI over 12 yearsYea, but why give out all my utility wrappers :)
-
Koen027 over 12 years@MyKuLLSKI I tested it and turns out you can. I think my error comes from the fact I was taught never to actually put an enum inside a class. Our professors always said they don't belong in a class and should be in the namespace scope.
-
Koen027 over 12 years@ivowiblo not gonna fix it now, too late for that. Might as well leave it as it is, to warn others who make the same assumption.
-
Koen027 over 12 years@MyKuLLSKI Never had any trouble with it really. Just put all the enums on the namespace scope and they work just fine. As I see it now, it's a choice you make as a programmer to do it like that. I haven't experienced any downsides to it yet.
-
Ralph Willgoss about 12 yearsbecause sharing is caring! :-)