Escape text for HTML
Solution 1
using System.Web;
var encoded = HttpUtility.HtmlEncode(unencoded);
Solution 2
Also, you can use this if you don't want to use the System.Web
assembly:
var encoded = System.Security.SecurityElement.Escape(unencoded)
Per this article, the difference between System.Security.SecurityElement.Escape()
and System.Web.HttpUtility.HtmlEncode()
is that the former also encodes apostrophe (')
characters.
Solution 3
If you're using .NET 4 or above and you don't want to reference System.Web
, you can use WebUtility.HtmlEncode
from System
var encoded = WebUtility.HtmlEncode(unencoded);
This has the same effect as HttpUtility.HtmlEncode
and should be preferred over System.Security.SecurityElement.Escape
.
Solution 4
In ASP.NET 4.0 there's new syntax to do this. Instead of
<%= HttpUtility.HtmlEncode(unencoded) %>
you can simply do
<%: unencoded %>
Read more here:
New <%: %> Syntax for HTML Encoding Output in ASP.NET 4 (and ASP.NET MVC 2)
Solution 5
.NET 4.0 and above:
using System.Web.Security.AntiXss;
//...
var encoded = AntiXssEncoder.HtmlEncode("input", useNamedEntities: true);
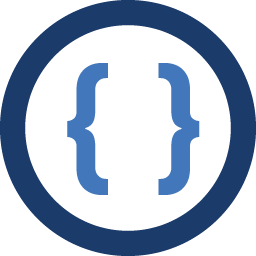
Admin
Updated on July 26, 2022Comments
-
Admin almost 2 years
How do i escape text for html use in C#? I want to do
sample="<span>blah<span>"
and have
<span>blah<span>
show up as plain text instead of blah only with the tags part of the html :(. Using C# not ASP
-
Admin about 15 yearsI made the question more clear. I dont want the tags to be part of html as the user can do </pre> and break it.
-
Gyuri over 14 yearsIf you also want to encode unicode characters to non-unicode, check out this: stackoverflow.com/questions/82008/…
-
Victor Sergienko about 11 yearsNot to say
SecurityElement.Escape()
escapes for XML which is not exactly HTML. -
Travis over 10 yearsWhy should it be preferred over SecurityElement.Escape? Are there vulnerabilities in the latter, or is the former just more capable?
-
Alex over 10 years@Travis There are no vulnerabilities in either, it's just that
SecurityElement.Escape
operates on XML andHtmlEncode
operates on HTML, and XML and HTML encoding have slightly different requirements (see this answer for details). So, for example,SecurityElement.Escape
is allowed to use'
, whileHtmlEncode
is not. -
pkExec over 9 yearsSomething that you don't want to find out the bad way: The above method by itself does not escape control characters. See the accepted answer here: stackoverflow.com/a/4501246/1543677 and use both.
-
Spets over 9 yearsGreat post thanks man this fixed exactly what I was looking for!
-
Tertium over 7 yearsHttpUtility does not exist anymore (win store apps)
-
Tertium over 7 yearsSystem.Security.SecurityElement does not exist in windows store apps
-
ruffin over 7 years@Travis I think the even better "excuse" is that System.Net is available to Portable Class Libraries and the other two options aren't/don't seem to be this morning. ;^)
-
mortb about 7 years
-
Admin over 6 yearsplease give a syntax for Razor? @Nacht
-
Bouke over 4 yearsYou are probably serving content using the wrong encoding. IE and Edge have no problems displaying such characters.
-
Peter Mortensen about 3 yearsRe "htmlencode": Do you mean "HtmlEncode"?
-
user2728841 almost 3 yearsNote that System.Security.SecurityElement.Escape does not remove or escape character 31, which hit us once from an extract of active directory data