ExpectedException in nUnit gave me an error
Solution 1
If you're using NUnit 3.0, then your error is because the ExpectedExceptionAttribute
has been removed. You should instead use a construct like the Throws Constraint.
For example, the tutorial you linked has this test:
[Test]
[ExpectedException(typeof(InsufficientFundsException))]
public void TransferWithInsufficientFunds()
{
Account source = new Account();
source.Deposit(200m);
Account destination = new Account();
destination.Deposit(150m);
source.TransferFunds(destination, 300m);
}
To change this to work under NUnit 3.0, change it to the following:
[Test]
public void TransferWithInsufficientFunds()
{
Account source = new Account();
source.Deposit(200m);
Account destination = new Account();
destination.Deposit(150m);
Assert.That(() => source.TransferFunds(destination, 300m),
Throws.TypeOf<InsufficientFundsException>());
}
Solution 2
Not sure if this changed recently but with NUnit 3.4.0 it provides Assert.Throws<T>
.
[Test]
public void TransferWithInsufficientFunds() {
Account source = new Account();
source.Deposit(200m);
Account destination = new Account();
destination.Deposit(150m);
Assert.Throws<InsufficientFundsException>(() => source.TransferFunds(destination, 300m));
}
Solution 3
If you still want to use Attributes, consider this:
[TestCase(null, typeof(ArgumentNullException))]
[TestCase("this is invalid", typeof(ArgumentException))]
public void SomeMethod_With_Invalid_Argument(string arg, Type expectedException)
{
Assert.Throws(expectedException, () => SomeMethod(arg));
}
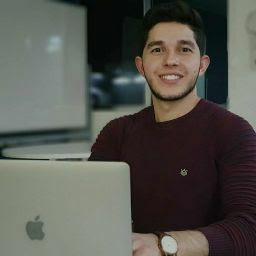
Basheer AL-MOMANI
about me is currently blank. :P no thing to see here go away :p
Updated on July 17, 2022Comments
-
Basheer AL-MOMANI almost 2 years
I'm new to using Testing Tools on the .NET Framework, so I downloaded it from NuGet with help from ReSharper.
I am using this Quick Start to learn how to use nUnit. I had just copied the code and an error came up on this attribute:
[ExpectedException(typeof(InsufficientFundsException))] //it is user defined Exception
The error is:
The type or namespace name 'ExpectedException' could not be found (are you missing a using directive or an assembly reference?)
Why? And if I need such functionality, what should I replace it with?
-
Najeeb about 5 yearsThanks for this. A question, though, what advantage does this grant over the previous attribute-based spec for exceptions? I don't think it adds any real benefit and only serves to make the code more unreadable.
-
Patrick Quirk about 5 yearsReadability is subjective (I prefer this syntax), but the biggest advantage is you can be precise about which statement throws the exception. With the attribute, if your setup code throws your expected exception, the test passes. Using this syntax, the test will fail.