Ext.onReady() vs $(document).ready()
Solution 1
No they're not the same, the first one will proc when your jQuery library is loaded, the Ext.onReady(.. will proc when your ExtJS library is loaded.
If you want to combine them you could do something like this:
var extReady = false;
var jQueryReady = false;
var librariesReady = function () {
if (jQueryReady && extReady) {
//They're both ready
}
};
$(document).ready(function () {
jQueryReady = true;
librariesReady();
});
Ext.onReady(function () {
extReady = true;
librariesReady();
});
Solution 2
Ext.onReady()
and $(document).ready()
have nothing to do about either library being loaded as the current accepted answer suggests.
According to the documentation both are about the DOM being loaded and ready.
Documentation
- Ext JS: https://docs.sencha.com/extjs/6.7.0/modern/Ext.html#method-onReady
- jQuery: https://api.jquery.com/ready/
An Answer to Your Case
It's possible that you're loading the Ext JS resource after your script fires, but jQuery is already loaded above your script. Thus using jQuery to wait until the DOM is loaded guarantees that the DOM has been loaded and thus by then Ext JS has also been loaded.
If you try to invert them and us Ext JS first you'll likely have an error.
According to the documentation they're doing the same thing so you shouldn't need to nest them
A Fix for this Scenario
If you are loading your resources like so:- jQuery
- Your Script
- Ext JS
- jQuery and/or Ext JS
- Order shouldn't matter as they can stand by themselves without requiring one or the other
- Your Script
Additional Explanation
Due to how the DOM is loaded and parsed by the time it reads your script it guarantees that jQuery and Ext JS are available. This is why you can reference their libraries in your script; you're not waiting for them to load they're already there and available to be used which is why you can call them and use their ready calls.
You need to use the ready event of one of the libraries to guarantee that all elements are loaded into the DOM and available to be accessed. You also shouldn't try to add anything to the DOM until it's ready although you can append to current elements that have been loaded above your element/script tag. It's just best practice to not touch the DOM until it's finished loading.
Additional Explanation Nobody Asked For 🔥
Handling DOM ready is more involved than these libraries make it which is why they both include such an event handler.
The following link explains with vanilla JS how you cannot only add your event listener you also need to check if it has already fired when you go to add your event listener for DOM ready. This is a common case to handle with eventing - where you create a race condition where an event may fire before you start listening for it - then you don't know that it ever happened without another way to check.
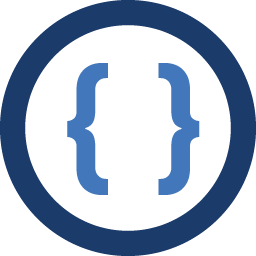
Admin
Updated on July 18, 2022Comments
-
Admin almost 2 years
Whats the difference? I have on $(document).ready function which should check if extjs is loaded but the main problem is extjs does not load on time and things inside $(document).ready starts to execute, extjs create function which produces the main error 'cannot execute create of undefined' on Ext.create("...", {..}); line. If i put double check like this:
$(document).ready(function() { Ext.onReady(function() { Ext.create('Ext.Button', {...}); }); });
Things magically work. Now I'm using ext-all.js which has ~1.3MB minified which is pretty large imho...and things get magically loaded while he does the second check...but I think those 2 functions are not the same as they definitions suggest, because if I put another $(document).ready instead of Ext.onReady() line, things break again. I think Ext.onReady({}); function does some other black magic which $(document).ready() does not, and I'm interested if someone knows what is this kind of magic?
Because it work's and I don't know why which is killing me.
Thanks for reading the post. =) ps. I'm using ExtJS for about day so I'm pretty new to it.
-
zloctb over 9 yearssencha.com/forum/… Ext.onReady() means wait til the DOM is ready and all required classes are loaded.
-
-
sra over 11 yearsYou were faster than me :) good one +1 (you may mention the load order of the scripts, cause that may also produce errors)
-
Admin over 11 yearsYes, I completely agree they are not quite the same as their definitions suggest. I actually have alot more complicated story but this is the essence of the solution. However, I still wonder how does he check if he is loaded? If he is not loaded wouldn't the Ext.onReady() function also drop error 'undefined' if library din't load this function on time? I'm really interested of the entire procedure of self checking(both of extjs and jquery). Because those 2 functions really do act differently ...edit: +1 from me to =)
-
Admin over 11 yearsok I found the answer which was bothering me here ...developer.mozilla.org/en-US/docs/Mozilla_event_reference/… ...thank you all for answers, especially Johan.
-
user2686101 about 10 yearsThe -2 is because you're wrong. The jquery ready loads when the DOM is ready. Ext.onReady is called when the DOM AND ext is ready.
-
Marco Sulla over 8 yearsThis is wrong. jQuery consists in only a single js file, so there's no need to wait for jQuery loading. jQuery.ready() checks if DOM is ready. Ext.onReady() checks if ExtJS classes are loaded AND if DOM is ready. IMHO it's more correct the answerof the poor @Mini0n that was downvoted.
-
CTS_AE about 5 yearsIf they were the same you wouldn't need to call both of them 😉 Unfortunately the accepted answer is wrong too. jQuery's ready isn't about the library being ready it's a shorthand for the DOM being ready. A vanilla DOM ready is more involved which is why people love jQuery's :)
-
CTS_AE about 5 years@MarcoSulla is correct, this answer is incorrect. According to both documentations they are for DOM ready event handling. Neither is about the library being loaded. I've added my own answer that digs deeper into the idea of the library being loaded and what the ready events are accomplishing.