ExtJS 4 Show data from multiple stores in grid
Solution 1
You should do this with your server side code and get this all data into a single store.
Solution 2
If you want to do this with associations, you need to define a renderer for your grid column.
Like so:
{
text: "orderDescription",
renderer: function(value,meta,record){//1.
//var orderStore = record.orderStore;//2a.
//var orderList = ordersStore.data.items;//2b.
var orderList = record.orders().data.items; //3.This line is the same as 2 the lines above(2a,2b).
var order = orderList[0]; //4. TODO..loop through your list.
var description = order.data.description; //5.
return description ;
},
I will try to explain for anyone who wants to do it this way.
- The third parameter is the current record in your 'User' Store being renderd for the grid. The first two just need to be there, to receive record. Leaving them out will not work.
- 1a/1b. I left these there to demonstrate how the association works. Basically, each record in your 'User' Store gets its own corresponding 'ordersStore'. It will be called 'ordersStore', because you put in your association
[name: 'orders']
. - The
orders()
method is automatically created, again based on the name 'orders'. This just returns theorderStore
. The store contains a fielddata
-> which contains a fielditems
.items
is the actual list of orders. - Now you have access to your list of orders. You can loop trough the orders now. Or if you have only one order, just the first one.
- Your
order
again contains a fielddata
which contains your actual data.
Solution 3
Lets try with below example-
Step 1: Adding records from Store 2 to Store 2.
var store2 = new Ext.data.Store({
...
});
var store1 = new Ext.data.Store({
...
listeners: {
load: function(store) {
store2.addRecords({records: store.getRange()},{add: true});
}
}
});
Step 2: Using records from Store 2 with Store 1.
For example, the first data col comes from Store 1 and the data from Store 2 forms cols 2 and 3. You can use a renderer that finds the data in the second store if the 'other' columns are just 'lookup' data, e.g.:
var store1 = new Ext.data.Store({
...,
fields: ['field1', 'field2']
});
var store2 = new Ext.data.Store({
...
id: 'field2',
fields: ['field2', 'fieldA', 'fieldB']
});
var renderA = function(value) {
var rec = store2.getById(value);
return rec ? rec.get('fieldA') : '';
}
var renderB = function(value) {
var rec = store2.getById(value);
return rec ? rec.get('fieldB') : '';
}
var columns = [
{header: 'Field 1', dataIndex: 'field1'},
{header: 'Field A', dataIndex: 'field2', renderer: renderA},
{header: 'Field B', dataIndex: 'field2', renderer: renderB}
];
Best of luck.
Ref. from here
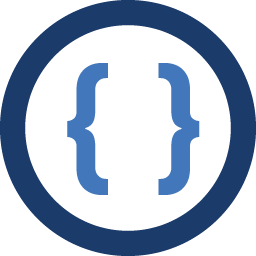
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I have 2 models - for example - Users and Orders
Ext.define('AM.model.User', { extend: 'Ext.data.Model', fields: ['id', 'username', 'firstName', 'lastName', 'state', 'city'], associations: [ { type: 'hasMany', model: 'Order', name: 'orders' },], }); Ext.define('AM.model.Order', { extend: 'Ext.data.Model', fields: ['id', 'userId', 'date', 'description', 'value'], belongsTo: 'User', });
and their stores. I'm looking for a way to display data from both stores in grid. (so my columns would be
firstName
,lastName
,orderDate
,orderDescription
,orderValue
...What is the proper way to display them?
Thanks.
-
Miles about 12 yearsWell, keep in mind that the orders() method not only returns a store for Orders records, but it's automatically filtered to only include orders that match the association you defined. In other words, when record is a user, and you call record.orders(), you get a store that includes only orders for that specific user, NOT every single order in the Order store.
-
Miles about 12 yearsI also question whether or not the store that is returned is pre-loaded as the answer suggests. In my projects, I've had to do a load after getting the store in order to get the related data records.
-
Renato H. about 12 yearsIt depends how you load your data. When you load a user and include the users orders in the json result, the related data is already loaded. Otherwise you have to load the related orders manually..