extract human vocals from song
Solution 1
You can always use the librosa library, which is favorite library for audio processing in python. It can be helpful in separating vocals (and other sporadic foreground signals) from accompanying instrumentation.
https://librosa.github.io/librosa_gallery/auto_examples/plot_vocal_separation.html
It takes the slice and plot the same slice, but separated into its foreground and background
To save the extracted foreground, you can use:
import librosa.output
new_y = librosa.istft(S_foreground*phase)
librosa.output.write_wav("./new-audio.wav", new_y, sr)
Solution 2
This is an answer to the question mentioned in the comments of the answer above:
how to save the generated S_foreground? @MaryamRahmaniMoghaddam
At first, import the output module of librosa package:
import librosa.output
Then, add the following code at the end of the python file:
new_y = librosa.istft(S_foreground*phase)
librosa.output.write_wav("./new-audio.wav", new_y, sr)
The python file I referred is accessible at the end of this website:
https://librosa.github.io/librosa_gallery/auto_examples/plot_vocal_separation.html
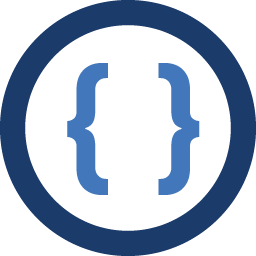
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
my question is about how to proceed in extracting human voice in music using the language python i have gone through this code but it extracts background music
from pydub import AudioSegment from pydub.playback import play # read in audio file and get the two mono tracks sound_stereo = AudioSegment.from_file(myAudioFile, format="mp3") sound_monoL = sound_stereo.split_to_mono()[0] sound_monoR = sound_stereo.split_to_mono()[1] # Invert phase of the Right audio file sound_monoR_inv = sound_monoR.invert_phase() # Merge two L and R_inv files, this cancels out the centers sound_CentersOut = sound_monoL.overlay(sound_monoR_inv) # Export merged audio file fh = sound_CentersOut.export(myAudioFile_CentersOut, format="mp3")
i need to extract human voice in song
if not this then how to subtract one audio file from another audio file
-
MRM over 4 yearshow to save the generated S_foreground ?
-
Alireza Kavian about 4 years@MaryamRahmaniMoghaddam I'll write the code for saving the new audio in the answers section