extract individual words from string c++
Solution 1
#include <string>
#include <sstream>
#include <vector>
using namespace std;
void split(const string &s, char delim, vector<string> &elems) {
stringstream ss(s);
string item;
while (getline(ss, item, delim)) {
elems.push_back(item);
}
}
vector<string> split(const string &s, char delim) {
vector<string> elems;
split(s, delim, elems);
return elems;
}
So in your case just do:
words = split(temp,' ');
Solution 2
You can use the operator >> direct to a microbuffer (string) to extract the word. (getline is not needed). Take a look at the function below:
vector<string> Extract(const string& Text) {
vector<string> Words;
stringstream ss(Text);
string Buf;
while (ss >> Buf)
Words.push_back(Buf);
return Words;
}
Solution 3
#include <algorithm> // std::(copy)
#include <iostream> // std::(cin, cout)
#include <iterator> // std::(istream_iterator, back_inserter)
#include <sstream> // std::(istringstream)
#include <string> // std::(string)
#include <vector> // std::(vector)
using namespace std;
auto main()
-> int
{
string user_input;
getline( cin, user_input );
vector<string> words;
{
istringstream input_as_stream( user_input );
copy(
istream_iterator<string>( input_as_stream ),
istream_iterator<string>(),
back_inserter( words )
);
}
for( string const& word : words )
{
cout << word << '\n';
}
}
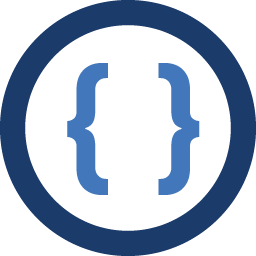
Admin
Updated on December 18, 2021Comments
-
Admin over 2 years
I am trying to make a C++ program that receives user input, and extracts the individual words in the string, e.g. "Hello to Bob" would get "Hello", "to", "Bob". Eventually, I will be pushing these into a string vector. This is the format I tried to use when designing the code:
//string libraries and all other appropriate libraries have been included above here string UserInput; getline(cin,UserInput) vector<string> words; string temp=UserInput; string pushBackVar;//this will eventually be used to pushback words into a vector for (int i=0;i<UserInput.length();i++) { if(UserInput[i]==32) { pushBackVar=temp.erase(i,UserInput.length()-i); //something like words.pushback(pushBackVar) will go here; } }
However, this only works for the first space encountered in the string.It does not work if there are any spaces before the word (e.g. if we have "Hello my World", pushBackVar will be "Hello" after the first loop, and then "Hello my" after the second loop, when I want "Hello" and "my".) How do I fix this? Is there any other better way to extract individual words from a string? I hope I haven't confused anyone.
-
Rakete1111 over 7 yearsYou should probably quote it, but I'm not sure.