Extract token from a curl request and use in another shell command
13,071
Solution 1
This is where a JSON parsing tool comes in handy (such as jq):
$ echo '{"token":"ac07098ad59ca6f3fccea0e2a2f6cb080df55c9a52fc9d65"}' | jq -r .token
ac07098ad59ca6f3fccea0e2a2f6cb080df55c9a52fc9d65
So
json=$( curl -k 'https://server:port/session' -X POST -H 'Content-Type: application/json' -d '{"username":"admin","password":"password"}' )
token=$( jq -r ".token" <<<"$json" )
curl https://server:port/ -k -X POST -H "X-Cookie:token=$token" ...
Solution 2
With no further tool than a bash (tested Centos/Rhel7/GitBash) :
json=$(curl -k 'https://server:port/session' \
-X POST -H 'Content-Type: application/json' \
-d '{"username":"admin","password":"password"}') \
&& token=$(echo $json | sed "s/{.*\"token\":\"\([^\"]*\).*}/\1/g") \
&& echo "token = $token"
then use your authentication needing commands like that :
curl https://server:port/ -k -X POST \
-H 'Content-Type: application/json' \
-H 'X-Cookie:token=$token' -d ...'
Solution 3
If Python is installed, and hopefully it is on modern systems, you can do something like:
OUTPUT="$(curl -k 'https://server:port/session' -X POST -H 'Content-Type: application/json' -d '{"username":"admin","password":"password"}' | python -c "import sys, json; print json.load(sys.stdin)['token']")"
This will give you:
echo $OUTPUT
ec2e99a1d294fd4bc0a04da852ecbdeed3b55671c08cc09f
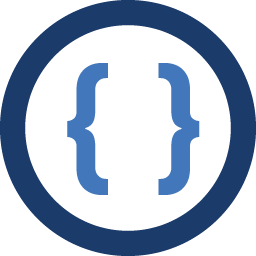
Author by
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I've started picking up bash scripting and I'm stuck at something I'm trying to wrap my head around.
I have a curl command that outputs a token and I need to use it in the following command:
curl -k 'https://server:port/session' -X POST -H 'Content-Type: application/json' -d '{"username":"admin","password":"password"}'
It then outputs a token here:
{"token":"ac07098ad59ca6f3fccea0e2a2f6cb080df55c9a52fc9d65"}
I then need to use it in the follow up command
curl https://server:port/ -k -X POST -H 'Content-Type: application/json' -H 'X-Cookie:token=token' -d '
I was thinking I could output the token to a file, then have a sed command write the token to a file, then the new command use a variable where token=$token
Thanks!